Website File Hosting
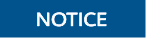
If you have any questions during development, post them on the Issues page of GitHub. For details about parameters and usage of each API, see the .
You can perform the following to implement website file hosting:
- Upload a website file to your bucket in OBS as an object and set the MIME type for the object.
- Set the ACL of the object to public-read.
- Access the object using a browser.
Sample code:
// Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables ACCESS_KEY_ID and SECRET_ACCESS_KEY_ID. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/eu/usermanual-ca/ca_01_0003.html. String ak = System.getenv("ACCESS_KEY_ID"); String sk = System.getenv("SECRET_ACCESS_KEY_ID"); String endPoint = "https://your-endpoint"; // Create an instance of ObsClient. ObsClient obsClient = new ObsClient(ak, sk, endPoint); // Upload objects and set the MIME type for the objects. PutObjectRequest request = new PutObjectRequest(); request.setBucketName("bucketname"); request.setObjectKey("test.html"); request.setFile(new File("localfile.html")); ObjectMetadata metadata = new ObjectMetadata(); metadata.setContentType("text/html"); request.setMetadata(metadata); obsClient.putObject(request); // Set the object ACL to public-read. obsClient.setObjectAcl("bucketname", "test.html", AccessControlList.REST_CANNED_PUBLIC_READ);
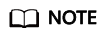
You can use https://bucketname.your-endpoint/test.html in a browser to access files hosted using the sample code.
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.