Deleting Objects
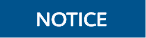
If you have any questions during development, post them on the Issues page of GitHub. For details about parameters and usage of each API, see API Reference.
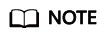
Exercise caution when performing this operation. If the versioning function is disabled for the bucket where the object is located, the object cannot be restored after being deleted.
Deleting a Single Object
You can call ObsClient->deleteObject to delete a single object. Sample code is as follows:
// Import the dependency library. require 'vendor/autoload.php'; // Import the SDK code library during source code installation. // require 'obs-autoloader.php'; // Declare the namespace. use Obs\ObsClient; // Create an instance of ObsClient. $obsClient = new ObsClient ( [ //Obtain an AK/SK pair using environment variables or import the AK/SK pair in other ways. Using hard coding may result in leakage. //Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/eu/usermanual-ca/ca_01_0003.html. 'key' => getenv('ACCESS_KEY_ID'), 'secret' => getenv('SECRET_ACCESS_KEY'), 'endpoint' => 'https://your-endpoint', 'signature' => 'obs' ] ); $resp = $obsClient->deleteObject ( [ 'Bucket' => 'bucketname', 'Key' => 'objectname' ] ); printf("RequestId:%s\n", $resp['RequestId']);
Batch Deleting Objects
You can call ObsClient->deleteObjects to delete objects in a batch.
A maximum of 1000 objects can be deleted each time. Two response modes are supported: verbose (detailed) and quiet (brief).
- In verbose mode (default mode), the returned response includes the deletion result of each requested object.
- In quiet mode, the returned response includes only results of objects failed to be deleted.
Sample code:
// Import the dependency library. require 'vendor/autoload.php'; // Import the SDK code library during source code installation. // require 'obs-autoloader.php'; // Declare the namespace. use Obs\ObsClient; // Create an instance of ObsClient. $obsClient = new ObsClient ( [ //Obtain an AK/SK pair using environment variables or import the AK/SK pair in other ways. Using hard coding may result in leakage. //Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/eu/usermanual-ca/ca_01_0003.html. 'key' => getenv('ACCESS_KEY_ID'), 'secret' => getenv('SECRET_ACCESS_KEY'), 'endpoint' => 'https://your-endpoint', 'signature' => 'obs' ] ); $resp = $obsClient->deleteObjects ( [ 'Bucket' => 'bucketname', // Set the response mode to verbose. 'Quiet' => false, 'Objects' => [ [ 'Key' => 'objectname1', 'VersionId' => null ], [ 'Key' => 'objectname2', 'VersionId' => null ] ] ] ); printf ( "RequestId:%s\n", $resp ['RequestId'] ); // Obtain the successfully deleted objects. printf ( "Deleteds:\n" ); foreach ( $resp ['Deleteds'] as $index => $deleted ) { printf ( "Deleteds[%d]", $index + 1 ); printf ( "Key:%s\n", $deleted ['Key'] ); printf ( "VersionId:%s\n", $deleted ['VersionId'] ); printf ( "DeleteMarker:%s\n", $deleted ['DeleteMarker'] ); printf ( "DeleteMarkerVersionId:%s\n", $deleted ['DeleteMarkerVersionId'] ); } // Obtain the objects failed to be deleted. printf ( "Errors:\n" ); foreach ( $resp ['Errors'] as $index => $error ) { printf ( "Errors[%d]", $index + 1 ); printf ( "Key:%s\n", $error ['Key'] ); printf ( "VersionId:%s\n", $$error ['VersionId'] ); printf ( "Code:%s\n", $error ['Code'] ); printf ( "Message:%s\n", $error ['Message'] ); }
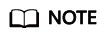
Use the Quiet parameter to specify the response mode and the Objects parameter to specify the to-be-deleted objects.
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.