Obtaining the Region of a Bucket (SDK for Node.js)
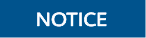
If you have any questions during development, post them on the Issues page of GitHub.
Function
This API returns the region of a bucket.
Restrictions
- To obtain the region of a bucket, you must be the bucket owner or have the required permission (obs:bucket:GetBucketLocation in IAM or GetBucketLocation in a bucket policy). For details, see Introduction to OBS Access Control, IAM Custom Policies, and Creating a Custom Bucket Policy.
Method
ObsClient.getBucketLocation(params)
Request Parameters
Parameter |
Type |
Mandatory (Yes/No) |
Description |
---|---|---|---|
Bucket |
string |
Yes |
Explanation: Bucket name. Restrictions:
Value range: The value can contain 3 to 63 characters. Default value: None |
Responses
Type |
Description |
---|---|
NOTE:
This API returns a Promise response, which requires the Promise or async/await syntax. |
Explanation: Returned results. For details, see Table 3. |
Parameter |
Type |
Description |
---|---|---|
CommonMsg |
Explanation: Common information generated after an API call is complete, including the HTTP status code and error code. For details, see Table 4. |
|
InterfaceResult |
Explanation: Results outputted for a successful call. For details, see Table 5. Restrictions: This parameter is not included if the value of Status is greater than 300. |
Parameter |
Type |
Description |
Status |
number |
Explanation: HTTP status code returned by the OBS server. Value range: A status code is a group of digits indicating the status of a response. It ranges from 2xx (indicating successes) to 4xx or 5xx (indicating errors). For details, see Status Codes. |
Code |
string |
Explanation: Error code returned by the OBS server. |
Message |
string |
Explanation: Error description returned by the OBS server. |
HostId |
string |
Explanation: Request server ID returned by the OBS server. |
RequestId |
string |
Explanation: Request ID returned by the OBS server. |
Id2 |
string |
Explanation: Request ID2 returned by the OBS server. |
Indicator |
string |
Explanation: Error code details returned by the OBS server. |
Parameter |
Type |
Description |
---|---|---|
RequestId |
string |
Explanation: Request ID returned by the OBS server |
Location |
string |
Explanation: Region where a bucket is located. Restrictions: If the endpoint used is obs.myhuaweicloud.eu, this parameter is not required. If any other endpoints are used, this parameter is required. Default value: If obs.myhuaweicloud.eu is used as the endpoint and no region is specified, eu-west-101 (the EU-Dublin region) is used by default. |
Code Examples
You can call ObsClient.getBucketLocation to obtain the location of a bucket. This example uses bucket examplebucket.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 |
// Import the OBS library. // Use npm to install the client. const ObsClient = require("esdk-obs-nodejs"); // Use the source code to install the client. // var ObsClient = require('./lib/obs'); // Create an instance of ObsClient. const obsClient = new ObsClient({ // Obtain an AK/SK pair using environment variables or import an AK/SK pair in other ways. Using hard coding may result in leakage. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/eu/usermanual-ca/ca_01_0003.html. access_key_id: process.env.ACCESS_KEY_ID, secret_access_key: process.env.SECRET_ACCESS_KEY, // (Optional) If you use a temporary AK/SK pair and a security token to access OBS, you are advised not to use hard coding, which may result in information leakage. You can obtain an AK/SK pair using environment variables or import an AK/SK pair in other ways. // security_token: process.env.SECURITY_TOKEN, // Enter the endpoint corresponding to the region where the bucket is located. EU-Dublin is used here in this example. Replace it with the one currently in use. server: "https://obs.eu-west-101.myhuaweicloud.eu" }); async function getBucketLocation() { try { const params = { // Specify the bucket name. Bucket: "examplebucket" }; // Obtain the bucket region. const result = await obsClient.getBucketLocation(params); if (result.CommonMsg.Status <= 300) { console.log("Get bucket(%s)'s location successful!", params.Bucket); console.log('Location: %s', result.InterfaceResult.Location); return; }; console.log("An ObsError was found, which means your request sent to OBS was rejected with an error response."); console.log("Status: %d", result.CommonMsg.Status); console.log("Code: %s", result.CommonMsg.Code); console.log("Message: %s", result.CommonMsg.Message); console.log("RequestId: %s", result.CommonMsg.RequestId); } catch (error) { console.log("An Exception was found, which means the client encountered an internal problem when attempting to communicate with OBS, for example, the client was unable to access the network."); console.log(error); }; }; getBucketLocation(); |
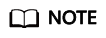
When creating a bucket, you can specify its location. For details, see Creating a Bucket (SDK for Node.js).
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.