Performing a Streaming Upload
Streaming upload uses System.IO.Stream as the data source of an object. You can call ObsClient.PutObject to upload the data streams to OBS. Sample code is as follows:
// Initialize configuration parameters. ObsConfig config = new ObsConfig(); config.Endpoint = "https://your-endpoint"; // Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables AccessKeyID and SecretAccessKey. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/eu/usermanual-ca/ca_01_0003.html. string accessKey= Environment.GetEnvironmentVariable("AccessKeyID", EnvironmentVariableTarget.Machine); string secretKey= Environment.GetEnvironmentVariable("SecretAccessKey", EnvironmentVariableTarget.Machine); // Create an instance of ObsClient. ObsClient client = new ObsClient(accessKey, secretKey, config); // Upload a stream. try { String str = "Hello OBS"; Stream stream = new MemoryStream(System.Text.Encoding.Default.GetBytes(str)); PutObjectRequest request = new PutObjectRequest() { BucketName = "bucketname", ObjectKey = "objectname", InputStream = stream, }; PutObjectResponse response = client.PutObject(request); Console.WriteLine("put object response: {0}", response.StatusCode); } catch (ObsException ex) { Console.WriteLine("ErrorCode: {0}", ex.ErrorCode); Console.WriteLine("ErrorMessage: {0}", ex.ErrorMessage); }
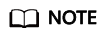
- To upload a large file, you are advised to use multipart upload.
- The content to be uploaded cannot exceed 5 GB.
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.