Example of Encryption
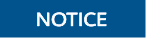
If you have any questions during development, post them on the Issues page of GitHub. For details about parameters and usage of each API, see the .
Encrypting an Object to Be Uploaded
Sample code:
// Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables ACCESS_KEY_ID and SECRET_ACCESS_KEY_ID. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/eu/usermanual-ca/ca_01_0003.html. String ak = System.getenv("ACCESS_KEY_ID"); String sk = System.getenv("SECRET_ACCESS_KEY_ID"); String endPoint = "https://your-endpoint"; // Create an ObsClient instance. ObsClient obsClient = new ObsClient(ak, sk, endPoint); PutObjectRequest request = new PutObjectRequest(); request.setBucketName("bucketname"); request.setObjectKey("objectname"); request.setFile(new File("localfile")); // Set the SSE-C encryption algorithm. SseCHeader sseCHeader = new SseCHeader(); sseCHeader.setAlgorithm(ServerAlgorithm.AES256); // Set the key used to encrypt and decrypt objects in SSE-C mode. This value is a Base64-encoded key. sseCHeader.setSseCKeyBase64("your base64 sse-c key generated by AES-256 algorithm"); request.setSseCHeader(sseCHeader); obsClient.putObject(request);
Decrypting a Downloaded Object
Sample code:
// Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables ACCESS_KEY_ID and SECRET_ACCESS_KEY_ID. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/eu/usermanual-ca/ca_01_0003.html. String ak = System.getenv("ACCESS_KEY_ID"); String sk = System.getenv("SECRET_ACCESS_KEY_ID"); String endPoint = "https://your-endpoint"; // Create an ObsClient instance. ObsClient obsClient = new ObsClient(ak, sk, endPoint); GetObjectRequest request = new GetObjectRequest("bucketname", "objectname"); // Set the decryption algorithm to SSE-C. SseCHeader sseCHeader = new SseCHeader(); sseCHeader.setAlgorithm(ServerAlgorithm.AES256); // The key used here must be the one used for uploading the object. sseCHeader.setSseCKeyBase64("your base64 sse-c key generated by AES-256 algorithm"); request.setSseCHeader(sseCHeader); ObsObject obsObject = obsClient.getObject(request); obsObject.getObjectContent().close();
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.