Go SDK
A synchronous function execution SDK is used as an example. To use the sample code, you must add the SDK dependency with the same language.
SDK Info |
Description |
---|---|
Version |
0.0.97 |
Installation |
go get -u github.com/huaweicloud/huaweicloud-sdk-go-v3 |
Links |
The request/response parameters and example requests/responses of the SDK are the same as those of the corresponding APIs. For details about the parameters and examples, see the API for executing a function synchronously.
SDK Request Example
package main import ( "fmt" "github.com/huaweicloud/huaweicloud-sdk-go-v3/core/auth/basic" functiongraph "github.com/huaweicloud/huaweicloud-sdk-go-v3/services/functiongraph/v2" "github.com/huaweicloud/huaweicloud-sdk-go-v3/services/functiongraph/v2/model" region "github.com/huaweicloud/huaweicloud-sdk-go-v3/services/functiongraph/v2/region" ) func main() { // This example is only used for testing purposes. Do not hardcode your AK/SK in the production environment. ak := "<YOUR AK>" sk := "<YOUR SK>" auth := basic.NewCredentialsBuilder(). WithAk(ak). WithSk(sk). Build() client := functiongraph.NewFunctionGraphClient( functiongraph.FunctionGraphClientBuilder(). WithRegion(region.ValueOf("<region>")). WithCredential(auth). Build()) request := &model.InvokeFunctionRequest{} request.FunctionUrn = "urn:fss:<region>:<project_id>:function:default:<func_name>:<version>" response, err := client.InvokeFunction(request) if err == nil { fmt.Printf("%+v\n", response) } else { fmt.Println(err) } }
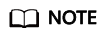
Obtain AK/SK, region (endpoint), and project_id by referring to AK/SK Signing and Authentication Guide.
Obtain func_name and version from the function details page.
X-CFF-Request-Version indicates the response body format. v0: text format; v1: JSON format. Select this format when using an SDK.
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot