Building an HTTP Function with Go
Introduction
This chapter describes how to deploy services on FunctionGraph using Go.
HTTP functions do not support direct code deployment using Go. This section uses binary conversion as an example to describe how to deploy Go programs on FunctionGraph.
Procedure
Building a code package
Create the source file main.go. The code is as follows:
// main.go package main import ( "fmt" "net/http" "github.com/emicklei/go-restful" ) func registerServer() { fmt.Println("Running a Go Http server at localhost:8000/") ws := new(restful.WebService) ws.Path("/") ws.Route(ws.GET("/hello").To(Hello)) c := restful.DefaultContainer c.Add(ws) fmt.Println(http.ListenAndServe(":8000", c)) } func Hello(req *restful.Request, resp *restful.Response) { resp.Write([]byte("nice to meet you")) } func main() { registerServer() }
# bootstrap /opt/function/code/go-http-demo
In main.go, an HTTP server is started using port 8000, and an API whose path is /hello is registered. When the API is invoked, "nice to meet you" is returned.
Compiling and packaging
- On the Linux server, compile the preceding code using the go build -o go-http-demo main.go command. Then, compress go-http-demo and bootstrap into a ZIP package named xxx.zip.
- To use the Golang compiler to complete packaging on a Windows host, perform the following steps:
# Switch the compilation environment # Check the previous Golang compilation environment go env # Set the following parameters to the corresponding value of Linux set GOARCH=amd64 go env -w GOARCH=amd64 set GOOS=linux go env -w GOOS=linux # go build -o [target executable program] [source program] # Example go build -o go-http-demo main.go # Restore the compilation environment set GOARCH=amd64 go env -w GOARCH=amd64 set GOOS=windows go env -w GOOS=windows
Creating an HTTP function and uploading code
Create an HTTP function and upload the xxx.zip package. For details, see Creating an HTTP Function.
Creating an APIG trigger
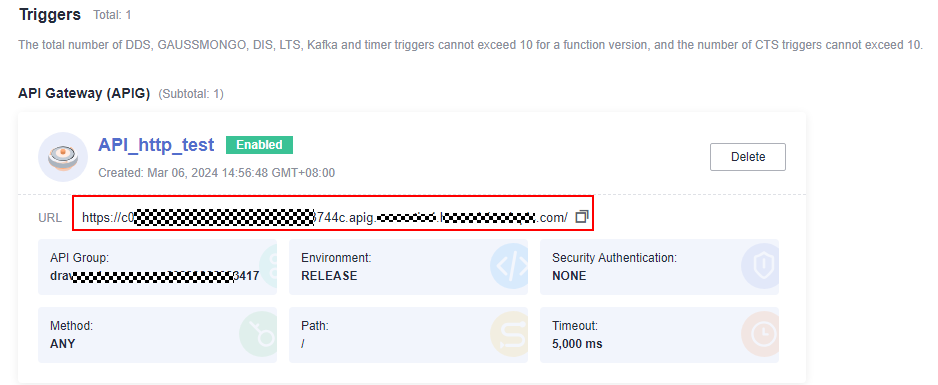
Invocation test

Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot