Deploying a Function Using a Container Image
Introduction
Package your container images complying with the Open Container Initiative (OCI) standard, and upload them to FunctionGraph. The images will be loaded and run by FunctionGraph. Unlike the code upload mode, you can use a custom code package, which is flexible and reduces migration costs. You can create HTTP functions by using a custom image.
For details about how to develop and deploy an HTTP function using a container image, see Developing an HTTP Function.
- Downloading images
Images are stored in SoftWare Repository for Container (SWR) and can only be downloaded by users with the SWR Admin permission. FunctionGraph will call the SWR API to generate and set temporary login commands before creating instances.
- Setting environment variables
Encryption settings and environment variables are supported. For details, see Configuring Environment Variables.
- Attaching external data disks
External data disks can be attached. For details, see Configuring Disk Mounting.
- Reserved instances
For details, see the description about reserved instances.
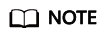
User containers will be started using UID 1003 and GID 1003, which are the same as other types of functions.
Prerequisites
You have created an agency with the SWR Admin permission by referring to Configuring Agency Permissions. Images are stored in SWR, and only users with this permission can invoke and pull images.
Procedure
- Log in to the FunctionGraph console. In the navigation pane, choose Functions > Function List.
- On the Function List page, click Create Function in the upper right corner.
- Select Container Image. For details, see Table 1.
Figure 1 Creating a function using a container image
Table 1 Parameter description Parameter
Description
*Function Type
Select a function type.
HTTP function: triggered once HTTP requests are sent to specific URLs.NOTE:- The custom container image must contain an HTTP server with listening port 8000.
- HTTP functions support APIG and APIC triggers only.
- When calling a function using APIG, isBase64Encoded is valued true by default, indicating that the request body transferred to FunctionGraph is encoded using Base64 and must be decoded for processing.
- The function must return characters strings by using the following structure.
{ "isBase64Encoded": true|false, "statusCode": httpStatusCode, "headers": {"headerName":"headerValue",...}, "body": "..." }
*Region
Select a region where you will deploy your code.
*Function Name
Name of the function, which must meet the following requirements:
- Consists of 1 to 60 characters, and can contain letters, digits, hyphens (-), and underscores (_).
- Starts with a letter and ends with a letter or digit.
*Enterprise Project
Select a created enterprise project and add the function to it. By default, default is selected.
Container Image
Enter an image URL, that is, the location of the container image. You can click View Image to view private and shared images.
Container Image Override
- CMD: container startup command. Example: /bin/sh. If no command is specified, the entrypoint or CMD in the image configuration will be used. Enter one or more commands separated with commas (,).
- Args: container startup parameter. Example: -args,value1. If no argument is specified, CMD in the image configuration will be used. Enter one or more arguments separated with commas (,).
- Working Dir: working directory of the container. The folder path can only be / and cannot be created or modified. The path will be / by default if not specified.
- User ID: user ID for running the image. If no user ID is specified, the default value 1003 will be used.
- Group ID: user group ID. If no user group ID is specified, the default value 1003 will be used.
Agency
Select an agency with the SWR Admin permission. To create an agency, see Creating an Agency.
- Command, Args, and Working dir can contain up to 5120 characters.
- When a function is executed at the first time, the image is pulled from SWR, and the container is started during cold start of the function, which takes a certain period of time. If there is no image on a node during subsequent cold starts, an image will be pulled from SWR.
- Public and private images are supported. For details, see Setting Image Attributes.
- The port of a custom container image must be 8000.
- The image package cannot exceed 10 GB. For a larger package, reduce the capacity. For example, mount the data of a question library to a container where the data was previously loaded through an external file system.
- FunctionGraph uses LTS to collect all logs that the container outputs to the console. These logs can be redirected to and printed on the console through standard output or an open-source log framework. The logs should include the system time, component name, code line, and key data, to facilitate fault locating.
- When an out of memory (OOM) error occurs, view the memory usage in the function execution result.
- Functions must return a valid HTTP response.
Sample Code
The following uses Node.js Express as an example. During function initialization, FunctionGraph uses the POST method to access the /init path (optional). Each time when a function is called, FunctionGraph uses the POST method to access the /invoke path. The function obtains context from req.headers, obtains event from req.body, and returns an HTTP response struct.
const express = require('express'); const app = express(); const PORT = 8000; app.post('/init', (req, res) => { res.send('Hello init\n'); }); app.post('/invoke', (req, res) => { res.send('Hello invoke\n'); }); app.listen(PORT, () => { console.log(`Listening on http://localhost:${PORT}`); });
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot