Java SDK
A synchronous function execution SDK is used as an example. To use the sample code, you must add the SDK dependency with the same language.
SDK Info |
Description |
---|---|
Version |
3.0.97 |
Installation |
<dependency> <groupId>com.huaweicloud.sdk</groupId> <artifactId>huaweicloud-sdk-functiongraph</artifactId> <version>3.0.97</version> </dependency> |
Links |
The request/response parameters and example requests/responses of the SDK are the same as those of the corresponding APIs. For details about the parameters and examples, see the API for executing a function synchronously.
SDK Request Example
package com.huaweicloud.sdk.test; import com.huaweicloud.sdk.core.auth.ICredential; import com.huaweicloud.sdk.core.auth.BasicCredentials; import com.huaweicloud.sdk.core.exception.ConnectionException; import com.huaweicloud.sdk.core.exception.RequestTimeoutException; import com.huaweicloud.sdk.core.exception.ServiceResponseException; import com.huaweicloud.sdk.functiongraph.v2.region.FunctionGraphRegion; import com.huaweicloud.sdk.functiongraph.v2.*; import com.huaweicloud.sdk.functiongraph.v2.model.*; public class InvokeFunctionSolution { public static void main(String[] args) { // This example is only used for testing purposes. Do not hardcode your AK/SK in the production environment. String ak = "<YOUR AK>"; String sk = "<YOUR SK>"; ICredential auth = new BasicCredentials() .withAk(ak) .withSk(sk); FunctionGraphClient client = FunctionGraphClient.newBuilder() .withCredential(auth) .withRegion(FunctionGraphRegion.valueOf("<region>")) .build(); InvokeFunctionRequest request = new InvokeFunctionRequest(); Map<String, Object> listbodyInvokeFunctionRequestBody = new HashMap<>(); listbodyInvokeFunctionRequestBody.put("k", "v"); request.withBody(listbodyInvokeFunctionRequestBody); request.withFunctionUrn("urn:fss:<region>:<project_id>:function:default:<func_name>:<version>"); request.withXCffLogType("tail"); request.withXCFFRequestVersion("v1"); try { InvokeFunctionResponse response = client.invokeFunction(request); System.out.println(response.toString()); } catch (ConnectionException e) { e.printStackTrace(); } catch (RequestTimeoutException e) { e.printStackTrace(); } catch (ServiceResponseException e) { e.printStackTrace(); System.out.println(e.getHttpStatusCode()); System.out.println(e.getErrorCode()); System.out.println(e.getErrorMsg()); } } }
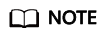
Obtain AK/SK, region (endpoint), and project_id by referring to AK/SK Signing and Authentication Guide.
Obtain func_name and version from the function details page.
X-CFF-Request-Version indicates the response body format. v0: text format; v1: JSON format. Select this format when using an SDK.
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot