Developing an HTTP Function
Introduction
When developing an HTTP function using a custom image, implement an HTTP server in the image and listen on port 8000 for requests. (Do not change port 8000 in the examples provided in this section.) HTTP functions support only APIG triggers.
Step 1: Prepare the Environment
To perform the operations described in this section, ensure that you have the FunctionGraph Administrator permissions, that is, the full permissions for FunctionGraph. For more information, see Permissions Management.
Step 2: Create an Image
Take the Linux x86 64-bit OS as an example. (No system configuration is required.)
- Create a folder.
mkdir custom_container_http_example && cd custom_container_http_example
- Implement an HTTP server. Node.js is used as an example. For details about other languages, see Creating an HTTP Function.
Create the main.js file to introduce the Express framework, receive POST requests, print the request body as standard output, and return "Hello FunctionGraph, method POST" to the client.
const express = require('express'); const PORT = 8000; const app = express(); app.use(express.json()); app.post('/*', (req, res) => { console.log('receive', req.body); res.send('Hello FunctionGraph, method POST'); }); app.listen(PORT, () => { console.log(`Listening on http://localhost:${PORT}`); });
- Create the package.json file for npm so that it can identify the project and process project dependencies.
{ "name": "custom-container-http-example", "version": "1.0.0", "description": "An example of a custom container http function", "main": "main.js", "scripts": {}, "keywords": [], "author": "", "license": "ISC", "dependencies": { "express": "^4.17.1" } }
- name: project name
- version: project version
- main: application entry file
- dependencies: all available dependencies of the project in npm
- Create a Dockerfile.
FROM node:12.10.0 ENV HOME=/home/custom_container ENV GROUP_ID=1003 ENV GROUP_NAME=custom_container ENV USER_ID=1003 ENV USER_NAME=custom_container RUN mkdir -m 550 ${HOME} && groupadd -g ${GROUP_ID} ${GROUP_NAME} && useradd -u ${USER_ID} -g ${GROUP_ID} ${USER_NAME} COPY --chown=${USER_ID}:${GROUP_ID} main.js ${HOME} COPY --chown=${USER_ID}:${GROUP_ID} package.json ${HOME} RUN cd ${HOME} && npm install RUN chown -R ${USER_ID}:${GROUP_ID} ${HOME} RUN find ${HOME} -type d | xargs chmod 500 RUN find ${HOME} -type f | xargs chmod 500 USER ${USER_NAME} WORKDIR / EXPOSE 8000 ENTRYPOINT ["node", "main.js"]
- FROM: Specify base image node:12.10.0. The base image is mandatory and its value can be changed.
- ENV: Set environment variables HOME (/home/custom_container), GROUP_NAME and USER_NAME (custom_container), USER_ID and GROUP_ID (1003). These environment variables are mandatory and their values can be changed.
- RUN: Use the format RUN <Command>. For example, RUN mkdir -m 550 ${HOME}, which means to create the home directory for user ${USER_NAME} during container building.
- USER: Switch to user ${USER_NAME}.
- WORKDIR: Switch the working directory to the / directory of user ${USER_NAME}.
- COPY: Copy main.js and package.json to the home directory of user ${USER_NAME} in the container.
- EXPOSE: Expose port 8000 of the container. Do not change this parameter.
- ENTRYPOINT: Run the node main.js command to start the container. Do not change this parameter.
- You can use any base image.
- In the cloud environment, UID 1003 and GID 1003 are used to start the container by default. The two IDs can be modified by choosing Configuration > Basic Settings > Container Image Override on the function details page. They cannot be root or a reserved ID.
- Do not change port 8000 in the example HTTP function.
- Build an image.
In the following example, the image name is custom_container_http_example, the tag is latest, and the period (.) indicates the directory where the Dockerfile is located. Run the image build command to pack all files in the directory and send the package to a container engine to build an image.
docker build -t custom_container_http_example:latest .
Step 3: Perform Local Verification
- Start the Docker container.
docker run -u 1003:1003 -p 8000:8000 custom_container_http_example:latest
- Open a new Command Prompt, and send a message through port 8000. You can access all paths in the root directory in the template code. The following uses helloworld as an example.
curl -XPOST -H 'Content-Type: application/json' -d '{"message":"HelloWorld"}' localhost:8000/helloworld
The following information is returned based on the module code:Hello FunctionGraph, method POST
- Check whether the following information is displayed:
receive {"message":"HelloWorld"}
Alternatively, run the docker logs command to obtain container logs.
Step 4: Upload the Image
Step 5: Create a Function
- In the left navigation pane of the management console, choose Compute > FunctionGraph. On the FunctionGraph console, choose Functions > Function List from the navigation pane.
- Click Create Function in the upper right corner and choose Container Image.
- Set the basic information.
- Function Type: Select HTTP Function.
- Function Name: Enter custom_container_http.
- Container Image: Select the image uploaded to SWR. Example: swr.{Region ID}.myhuaweicloud.com/{Organization name}/{Image name}:{Image tag}
- Agency: Select an agency with the SWR Admin permission. If no agency is available, create one by referring to Creating an Agency.
- After the configuration is complete, click Create Function.
Step 6: Test the Function
- On the function details page, click Test. In the displayed dialog box, create a test event.
- Select apig-event-template, set Event Name to helloworld, modify the test event as follows, and click Create.
{ "body": "{\"message\": \"helloworld\"}", "requestContext": { "requestId": "11cdcdcf33949dc6d722640a13091c77", "stage": "RELEASE" }, "queryStringParameters": { "responseType": "html" }, "httpMethod": "POST", "pathParameters": {}, "headers": { "Content-Type": "application/json" }, "path": "/helloworld", "isBase64Encoded": false }
Step 7: View the Execution Result
Click Test and view the execution result on the right.
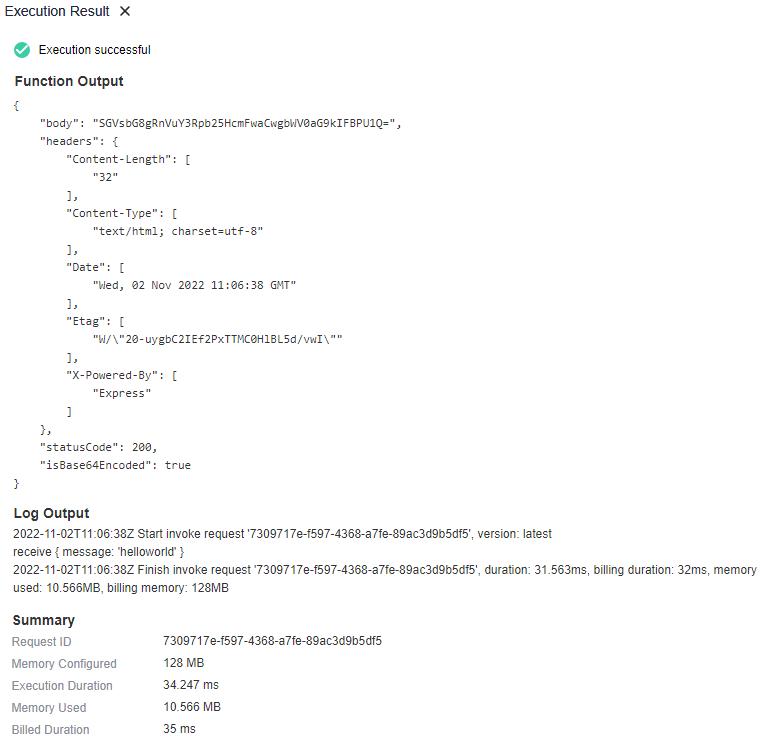
- Function Output: displays the return result of the function.
- Log Output: displays the execution logs of the function.
- Summary: displays key information of the logs.
Step 8: View Monitoring Metrics
On the function details page, click the Monitoring tab.
- On the Monitoring tab page, choose Metrics, and select a time range (such as 5 minutes, 15 minutes, or 1 hour) to query the function.
- The following metrics are displayed: invocations, errors, duration (maximum, average, and minimum durations), throttles, and instance statistics (reserved instances).
Step 9: Delete the Function
- On the function details page, choose Operation > Delete Function in the upper right corner.
- In the confirmation dialog box, enter DELETE and click OK to release resources in a timely manner.
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot