.NET SDK
This section describes how to use the .NET SDK to quickly develop OCR services as needed.
Prerequisites
- You have registered a Huawei ID and enabled Huawei Cloud services. Your account cannot be in arrears or frozen.
- .NET Standard 2.0 or later and C# 4.0 or later are available.
- You have obtained an AK and an SK on the My Credentials > Access Keys page. The AK and SK are contained in the credentials.csv file.
Figure 1 Creating an access key
- You have obtained the IAM user name, account name, and the project ID of your target region on the My Credentials > API Credentials page. The information will be used during service calling. Save it in advance.
Figure 2 API Credentials
Installing the SDK
Before you use the SDK, install HuaweiCloud.SDK.Core and HuaweiCloud.SDK.Ocr in either of the following ways:
- Using .NET CLI
dotnet add package HuaweiCloud.SDK.Core dotnet add package HuaweiCloud.SDK.Ocr
- Using Package Manager
Install-Package HuaweiCloud.SDK.Core Install-Package HuaweiCloud.SDK.Ocr
Getting Started
- Import dependency modules.
using System; using System.Collections.Generic; using HuaweiCloud.SDK.Core; using HuaweiCloud.SDK.Core.Auth; using HuaweiCloud.SDK.Ocr; using HuaweiCloud.SDK.Ocr.V1; using HuaweiCloud.SDK.Ocr.V1.Model;
- Configure client connection parameters.
- Using the default configuration
// Use the default configuration. var config = HttpConfig.GetDefaultConfig();
- (Optional) Configuring a network proxy
// Configure network proxy as needed. config.ProxyHost = "proxy.huaweicloud.com"; config.ProxyPort = 8080; config.ProxyUsername = "test"; config.ProxyPassword = "test";
- (Optional) Configuring the timeout
// The default connection timeout interval is 120 seconds. You can change it as needed. config.Timeout = 120;
- (Optional) Configuring an SSL
// Configure whether to skip SSL certificate verification as needed. config.IgnoreSslVerification = true;
- Using the default configuration
- Configure authentication information.
Configure ak and sk. AK is used together with SK to sign requests cryptographically, ensuring that the requests are secret, complete, and correct. For details about how to obtain the AK and SK, see Prerequisites.
const string ak = Environment.GetEnvironmentVariable("HUAWEICLOUD_SDK_AK"); const string sk = Environment.GetEnvironmentVariable("HUAWEICLOUD_SDK_SK"); var auth = new BasicCredentials(ak, sk);
NOTE:
- Hard-coded or plaintext AK and SK are risky. For security purposes, encrypt your AK and SK and store them in the configuration file or environment variables.
- In this example, the AK and SK are stored in environment variables for identity authentication. Before running this example, configure environment variables HUAWEICLOUD_SDK_AK and HUAWEICLOUD_SDK_SK.
- Initialize the client (using either of the following methods).
- (Recommended) Specifying a region for a cloud service
// Initialize the client {Service}Client of a specified cloud service. The following uses OcrClient of OCR as an example. var client = OcrClient.NewBuilder() .WithCredential(auth) .WithRegion(OcrRegion.ValueOf("ap-southeast-2")) .WithHttpConfig(config) .Build();
- Specifying an endpoint for a cloud service
// Specify the endpoint for OCR, for example, AP-Bangkok. String endpoint = "https://ocr.ap-southeast-2.myhuaweicloud.com"; // Initialize the client authentication information. You need to enter the corresponding project ID. The following uses BasicCredentials as an example. var auth = new BasicCredentials(ak, sk, projectId); // Initialize the client {Service}Client of a specified cloud service. The following uses OcrClient of OCR as an example. var client = OcrClient.NewBuilder() .WithCredential(auth) .WithEndPoint(endpoint) .WithHttpConfig(config) .Build();
endpoint indicates the endpoints for Huawei Cloud services. For details, see Regions and Endpoints.
- (Recommended) Specifying a region for a cloud service
- Send a request and check the response.
// The following uses calling the RecognizePassport API of Passport OCR as an example. var req = new RecognizePassportRequest { }; req.Body = new PassportRequestBody() { Url = "Image URL" }; try { var resp = client.RecognizePassport(req); var respStatusCode = resp.HttpStatusCode; Console.WriteLine(respStatusCode); }
- Handle the exception.
Table 1 Exception handling Level-1 Category
Level-1 Category Description
Level-2 Category
Level-2 Category Description
ConnectionException
Connection exception
HostUnreachableException
The network is unreachable or access is rejected.
SslHandShakeException
SSL authentication is abnormal.
RequestTimeoutException
Response timeout exception
CallTimeoutException
The server fails to respond to a single request before timeout.
RetryOutageException
No valid response is returned after the maximum number of retries specified in the retry policy is reached.
ServiceResponseException
Server response exception
ServerResponseException
Internal server error. HTTP response code: [500,].
ClientRequestException
Invalid request parameter. HTTP response code: [400, 500).
try { var resp = client.RecognizePassport(req); var respStatusCode = resp.HttpStatusCode; Console.WriteLine(respStatusCode); } catch (RequestTimeoutException requestTimeoutException) { Console.WriteLine(requestTimeoutException.ErrorMessage); } catch (ServiceResponseException clientRequestException) { Console.WriteLine(clientRequestException.HttpStatusCode); Console.WriteLine(clientRequestException.ErrorCode); Console.WriteLine(clientRequestException.ErrorMsg); } catch (ConnectionException connectionException) { Console.WriteLine(connectionException.ErrorMessage); }
For details about how to use the asynchronous client and configure logs, see SDK Center and .NET SDK Usage Guide.
Automatic Generation of Sample Code
API Explorer allows for API search and platform debugging, with features such as quick and comprehensive search, visual debugging, access to help documentation, and online consultation.
You only need to modify API parameters in the API Explorer to automatically generate the corresponding sample code.
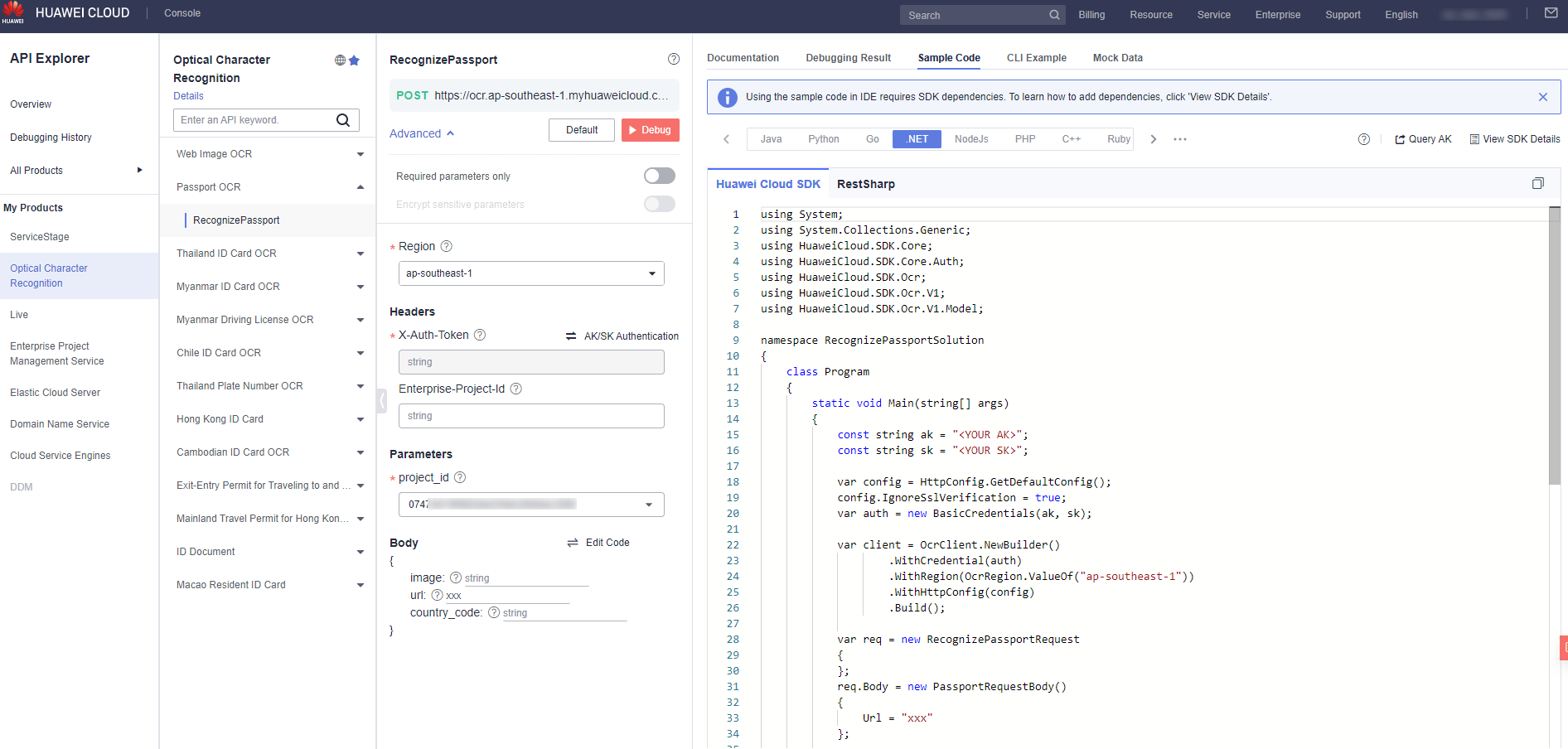
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot