C++ SDK
This section describes how to use the new C++ SDK to quickly develop OCR services.
Prerequisites
- You have registered a Huawei ID and enabled Huawei Cloud services. Your account cannot be in arrears or frozen.
- C++ 14 or later and CMake 3.10 or later are available.
- You have obtained an AK and an SK on the My Credentials > Access Keys page. The AK and SK are contained in the credentials.csv file.
Figure 1 Creating an access key
- You have obtained the IAM user name, account name, and the project ID of your target region on the My Credentials > API Credentials page. The information will be used during service calling. Save it in advance.
Figure 2 API Credentials
Installing the SDK
- Installing the SDK on a Linux VM
- Obtain dependency packages.
The required third-party software packages are contained in the package management tools of most Linux OSs, for example, Debian and Ubuntu.
sudo apt-get install libcurl4-openssl-dev libboost-all-dev libssl-dev libcpprest-dev
spdlog needs to be installed using the source code.
git clone https://github.com/gabime/spdlog.git cd spdlog mkdir build cd build cmake -DCMAKE_POSITION_INDEPENDENT_CODE=ON .. // Used to generate a dynamic library. make sudo make install
- Perform compilation and installation.
git clone https://github.com/huaweicloud/huaweicloud-sdk-cpp-v3.git cd huaweicloud-sdk-cpp-v3 mkdir build cd build cmake .. make sudo make install
After the operations are complete, the installation directory of the C++ SDK is /usr/local.
- Obtain dependency packages.
- Installing the SDK on a Windows VM
- Install vcpkg and use it to install required software packages.
vcpkg install curl cpprestsdk boost openssl spdlog
- Use CLion for compilation.
- Use CLion to open the huaweicloud-sdk-cpp-v3 directory.
- Choose .
- Choose Build > Execution > Deployment > CMake.
- Add the following content to CMake options:
-DCMAKE_TOOLCHAIN_FILE={your vcpkg install dir}/scripts/buildsystems/vcpkg.cmake
- Right-click CMakeLists.txt and choose Load CMake Project from the shortcut menu.
- Choose Build to start compilation.
- Install the C++ SDK.
After the compilation is complete, choose .
After the operations are complete, the installation directory of the C++ SDK is C:\Program File (x86)\huaweicloud-sdk-cpp-v3.
- Install vcpkg and use it to install required software packages.
Getting Started
- Import dependency modules.
//include <cstdlib> //include <iostream> //include <string> //include <memory> //include <huaweicloud/core/exception/Exceptions.h> //include <huaweicloud/core/Client.h> //include <huaweicloud/ocr/v1/OcrClient.h> using namespace HuaweiCloud::Sdk::Ocr::V1; using namespace HuaweiCloud::Sdk::Ocr::V1::Model; using namespace HuaweiCloud::Sdk::Core; using namespace HuaweiCloud::Sdk::Core::Exception; using namespace std;
- Configure client connection parameters.
- Using the default configuration
// Use the default configuration. HttpConfig httpConfig = HttpConfig();
- (Optional) Configuring a network proxy
// Configure network proxy as needed. httpConfig.setProxyProtocol("http"); httpConfig.setProxyHost("proxy.huawei.com"); httpConfig.setProxyPort("8080"); httpConfig.setProxyUser("username"); httpConfig.setProxyPassword("password");
- (Optional) Configuring the timeout
// The default connection timeout interval is 60 seconds, and the default read timeout interval is 120 seconds. You can change the default values as needed. httpConfig.setConnectTimeout(60); httpConfig.setReadTimeout(120);
- (Optional) Configuring an SSL
// Skip server certificate verification. httpConfig.setIgnoreSslVerification(true);
- Using the default configuration
- Configure authentication information.
Configure ak, sk, and projectId. AK is used together with SK to sign requests cryptographically, ensuring that the requests are secret, complete, and correct.
string ak = getenv("HUAWEICLOUD_SDK_AK"); string sk = getenv("HUAWEICLOUD_SDK_SK"); string projectId = getenv("PROJECT_ID"); auto auth = std::make_unique<BasicCredentials>(); auth->withAk(ak) .withSk(sk) .withProjectId(projectId);
NOTE:
- Hard-coded or plaintext AK and SK are risky. For security purposes, encrypt your AK and SK and store them in the configuration file or environment variables.
- In this example, the AK and SK are stored in environment variables for identity authentication. Before running this example, configure environment variables HUAWEICLOUD_SDK_AK and HUAWEICLOUD_SDK_SK.
The authentication parameters are described as follows:
- ak and sk: access key and secrete access key, respectively. For how to obtain them, see Prerequisites.
- projectId: Huawei Cloud project ID. For details about how to obtain the ID, see Prerequisites.
- Initialize the client.
Specifying an endpoint for a cloud service
string endpoint = "https://ocr.cn-north-4.myhuaweicloud.com"; auto client = OcrClient::newBuilder() .withCredentials(std::unique_ptr<Credentials>(auth.release())) .withHttpConfig(httpConfig) .withEndPoint(endpoint) .build();
endpoint: endpoints for Huawei Cloud services. For details, see Regions and Endpoints.
- Send a request and check the response.
// The following uses calling the RecognizePassport API of Passport OCR as an example. RecognizePassportRequest request; PassportRequestBody body; body.setUrl("Image URL"); request.setBody(body); std::cout << "-----begin execute request-------" << std::endl;
- Handle the exception.
Table 1 Exception handling Level-1 Category
Level-1 Category Description
Level-2 Category
Level-2 Category Description
ConnectionException
Connection exception
HostUnreachableException
The network is unreachable or access is rejected.
SslHandShakeException
SSL authentication is abnormal.
RequestTimeoutException
Response timeout exception
CallTimeoutException
The server fails to respond to a single request before timeout.
RetryOutageException
No valid response is returned after the maximum number of retries specified in the retry policy is reached.
ServiceResponseException
Server response exception
ServerResponseException
Internal server error. HTTP response code: [500,].
ClientRequestException
Invalid request parameter. HTTP response code: [400, 500).
std::cout << "-----begin execute request-------" << std::endl; try { auto reponse = client->recognizePassport(request); std::cout << reponse->getHttpBody() << std::endl; } catch (HostUnreachableException& e) { std::cout << "host unreachable:" << e.what() << std::endl; } catch (SslHandShakeException& e) { std::cout << "ssl handshake error:" << e.what() << std::endl; } catch (RetryOutageException& e) { std::cout << "retryoutage error:" << e.what() << std::endl; } catch (CallTimeoutException& e) { std::cout << "call timeout:" << e.what() << std::endl; } catch (ServiceResponseException& e) { std::cout << "http status code:" << e.getStatusCode() << std::endl; std::cout << "error code:" << e.getErrorCode() << std::endl; std::cout << "error msg:" << e.getErrorMsg() << std::endl; std::cout << "RequestId:" << e.getRequestId() << std::endl; } catch (exception& e) { std:cout << "unknown exception:" << e.what() << std::endl; }
For details about how to use the asynchronous client and configure logs, see SDK Center and C++ SDK Usage Guide.
Automatic Generation of Sample Code
API Explorer allows for API search and platform debugging, with features such as quick and comprehensive search, visual debugging, access to help documentation, and online consultation.
You only need to modify API parameters in the API Explorer to automatically generate the corresponding sample code.
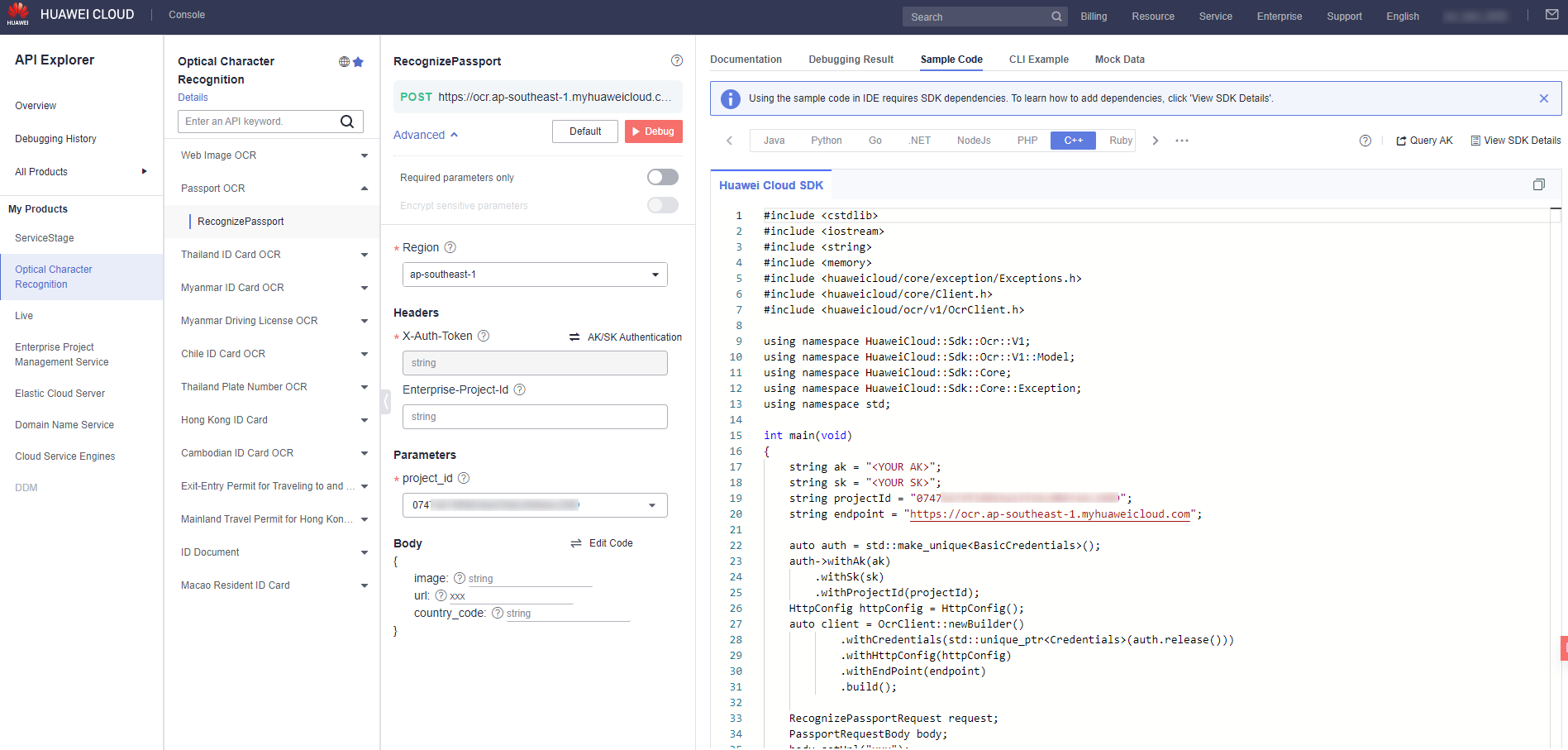
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot