Go SDK
This section describes how to use the Go SDK to quickly develop OCR services.
Prerequisites
- You have registered a Huawei ID and enabled Huawei Cloud services. Your account cannot be in arrears or frozen.
- Go 1.14 or later is available. You can run the go version command to check the version information.
- You have obtained an AK and an SK on the My Credentials > Access Keys page. The AK and SK are contained in the credentials.csv file.
Figure 1 Creating an access key
- You have obtained the IAM user name, account name, and the project ID of your target region on the My Credentials > API Credentials page. The information will be used during service calling. Save it in advance.
Figure 2 API Credentials
Installing the SDK
Before you use the SDK, you need to install the Huawei Cloud Go SDK library.
// Install the Huawei Cloud Go SDK library. go get github.com/huaweicloud/huaweicloud-sdk-go-v3
Getting Started
- Import dependency modules.
import ( "fmt" "github.com/huaweicloud/huaweicloud-sdk-go-v3/core/auth/basic" ocr "github.com/huaweicloud/huaweicloud-sdk-go-v3/services/ocr/v1" "github.com/huaweicloud/huaweicloud-sdk-go-v3/services/ocr/v1/model" region "github.com/huaweicloud/huaweicloud-sdk-go-v3/services/ocr/v1/region" )
- Configure authentication information.
Configure ak and sk. AK is used together with SK to sign requests cryptographically, ensuring that the requests are secret, complete, and correct. For details about how to obtain the AK and SK, see Prerequisites.
func main() { ak := os.Getenv("HUAWEICLOUD_SDK_AK") sk := os.Getenv("HUAWEICLOUD_SDK_SK") auth := basic.NewCredentialsBuilder(). WithAk(ak). WithSk(sk). Build() }
- Hard-coded or plaintext AK and SK are risky. For security purposes, encrypt your AK and SK and store them in the configuration file or environment variables.
- In this example, the AK and SK are stored in environment variables for identity authentication. Before running this example, configure environment variables HUAWEICLOUD_SDK_AK and HUAWEICLOUD_SDK_SK.
- Initialize the client (using either of the following methods).
- (Recommended) Specifying a region for a cloud service
// Initialize the client New{Service}Client of a specified cloud service. The following uses the AP-Bangkok region as an example. func main() { client := ocr.NewOcrClient( ocr.OcrClientBuilder(). WithRegion(region.ValueOf("ap-southeast-2")). WithCredential(auth). Build()) }
- Specifying an endpoint for a cloud service
func main() { // Specify the endpoint for OCR, for example, AP-Bangkok. endpoint:="https://ocr.ap-southeast-2.myhuaweicloud.com" // If you choose to specify the endpoint, add projectId to the authentication information. auth := basic.NewCredentialsBuilder(). WithAk(ak). WithSk(sk). WithProjectId(projectId). Build() // Initialize the client New{Service}Client of a specified cloud service. client := ocr.NewOcrClient( ocr.OcrClientBuilder(). WithEndpoint(endpoint). WithCredential(auth). Build()) }
endpoint indicates the endpoints for Huawei Cloud services. For details, see Regions and Endpoints.
- (Recommended) Specifying a region for a cloud service
- Send a request and check the response.
// The following uses calling the RecognizePassport API of Passport OCR as an example. request := &model.RecognizePassportRequest{} urlPassportRequestBody:= "Image URL" request.Body = &model.PassportRequestBody{ Url: &urlPassportRequestBody, } response, err := client.RecognizePassport(request) if err == nil { fmt.Printf("%+v\n", response) } else { fmt.Println(err) }
- Handle the exception.
Table 1 Exception handling Level-1 Category
Level-1 Category Description
ServiceResponseError
A service response exception occurs.
url.Error
A URL exception occurs.
response, err := client.RecognizePassport(request) if err == nil { fmt.Printf("%+v\n", response) } else { fmt.Println(err) }
Automatic Generation of Sample Code
API Explorer allows for API search and platform debugging, with features such as quick and comprehensive search, visual debugging, access to help documentation, and online consultation.
You only need to modify API parameters in the API Explorer to automatically generate the corresponding sample code.
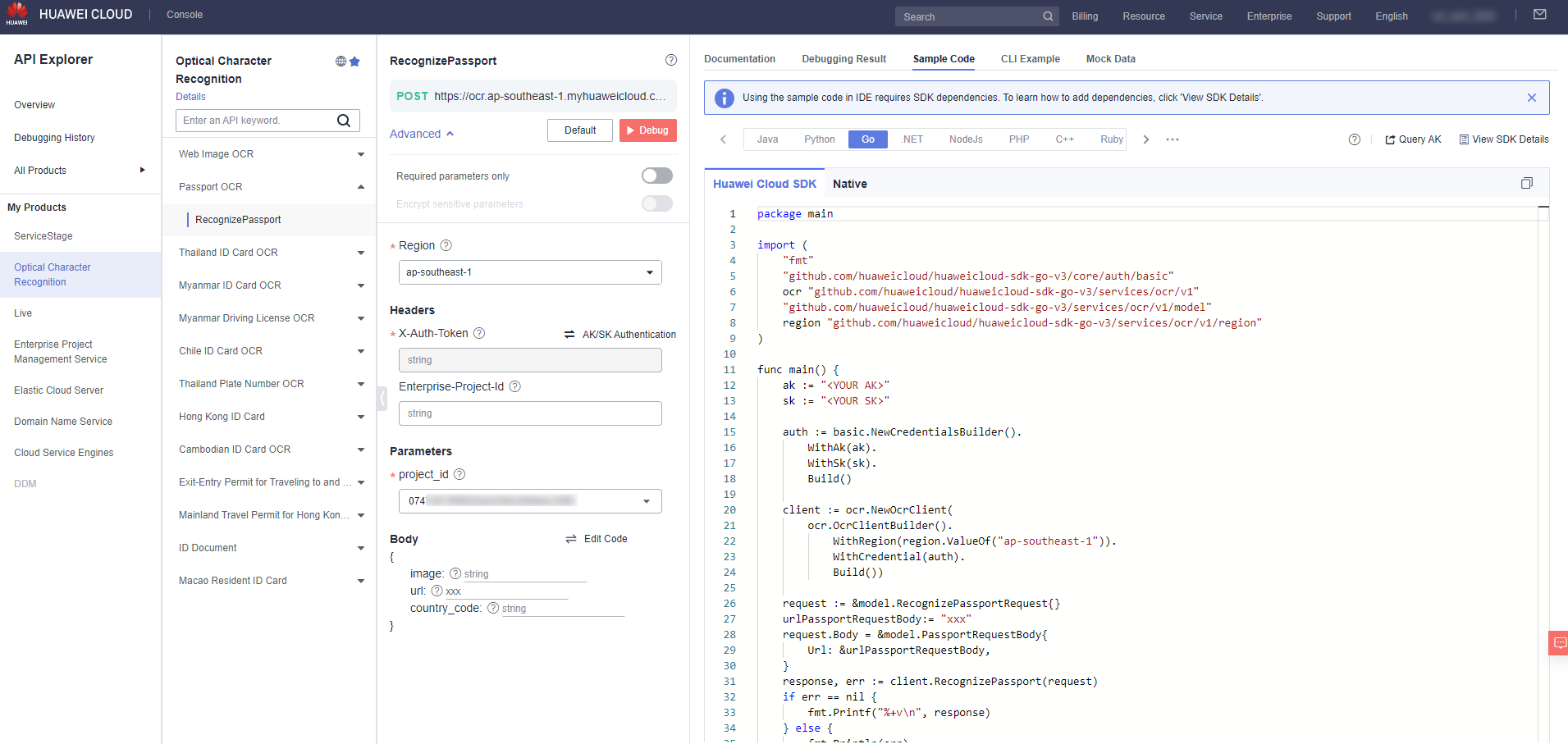
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot