Node.js SDK
This section describes how to use the new Node.js SDK to quickly develop OCR services.
Prerequisites
- You have registered a Huawei ID and enabled Huawei Cloud services. Your account cannot be in arrears or frozen.
- Node 10.16.1 or later is available.
- You have obtained an AK and an SK on the My Credentials > Access Keys page. The AK and SK are contained in the credentials.csv file.
Figure 1 Creating an access key
- You have obtained the IAM user name, account name, and the project ID of your target region on the My Credentials > API Credentials page. The information will be used during service calling. Save it in advance.
Figure 2 API Credentials
Installing the SDK
Before you use SDK, install @huaweicloud/huaweicloud-sdk-core and @huaweicloud/huaweicloud-sdk-ocr.
You are advised to use npm to install the SDK.
npm install @huaweicloud/huaweicloud-sdk-core npm i @huaweicloud/huaweicloud-sdk-ocr
Getting Started
- Import dependency modules.
const core = require('@huaweicloud/huaweicloud-sdk-core'); const ocr = require("@huaweicloud/huaweicloud-sdk-ocr");
- Configure client connection parameters.
- Using the default configuration
const client = ocr.OcrClient.newBuilder()
- (Optional) Configuring a network proxy
// (Optional) Use a proxy server. client.withProxyAgent("http://username:password@proxy.huaweicloud.com:8080")
- (Optional) Configuring an SSL
// (Optional) Skip server certificate verification. process.env.NODE_TLS_REJECT_UNAUTHORIZED = "0"
- Using the default configuration
- Configure authentication information.
Configure ak, sk, and project_id. AK is used together with SK to sign requests cryptographically, ensuring that the requests are secret, complete, and correct.
Initialize authentication information.
const ak = process.env.HUAWEICLOUD_SDK_AK; const sk = process.env.HUAWEICLOUD_SDK_SK; const credentials = new core.BasicCredentials() .withAk(ak) .withSk(sk) .withProjectId(project_id)
- Hard-coded or plaintext AK and SK are risky. For security purposes, encrypt your AK and SK and store them in the configuration file or environment variables.
- In this example, the AK and SK are stored in environment variables for identity authentication. Before running this example, configure environment variables HUAWEICLOUD_SDK_AK and HUAWEICLOUD_SDK_SK.
The authentication parameters are described as follows:
- ak and sk: access key and secrete access key, respectively. For how to obtain them, see Prerequisites.
- project_id: Huawei Cloud project ID. For details about how to obtain the ID, see Prerequisites.
- Initialize the client.
Specifying an endpoint for a cloud service
// Specify the endpoint for OCR, for example, CN North-Beijing4. const client = ocr.OcrClient.newBuilder() .withCredential(credentials) .withEndpoint(endpoint) .build();
endpoint indicates the endpoints for Huawei Cloud services. For details, see Regions and Endpoints.
- Send a request and check the response.
// The following uses calling the RecognizePassport API of Passport OCR as an example. const request = new ocr.RecognizePassportRequest(); const body = new ocr.PassportRequestBody(); body.withUrl("Image URL"); request.withBody(body); const result = client.recognizePassport(request); result.then(result => { console.log("JSON.stringify(result)::" + JSON.stringify(result)); }).catch(ex => { console.log("exception:" + JSON.stringify(ex)); });
Automatic Generation of Sample Code
API Explorer allows for API search and platform debugging, with features such as quick and comprehensive search, visual debugging, access to help documentation, and online consultation.
You only need to modify API parameters in the API Explorer to automatically generate the corresponding sample code.
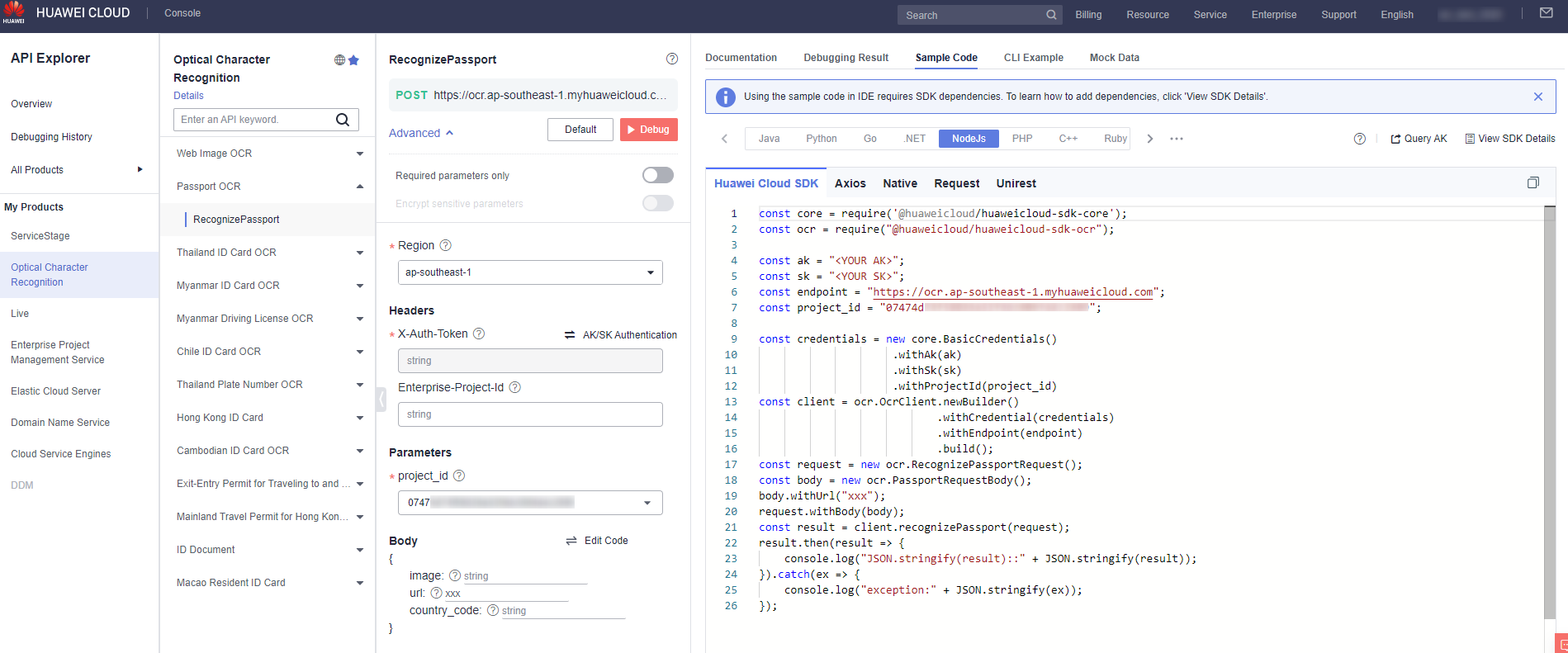
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot