Configuring RabbitMQ Dead Letter Messages
Dead lettering is a message mechanism in RabbitMQ. When a message is consumed, it becomes a dead letter message if any of the following happens:
- requeue is set to false, and the consumer uses basic.reject or basic.nack to negatively acknowledge (NACK) the message.
- The message has stayed in the queue for longer than the configured TTL.
- The number of messages in the queue exceeds the maximum queue length.
Such a message will be stored in a dead letter queue, if any, for special treatment. If there is no dead letter queue, the message will be discarded.
For more information about dead lettering, see Dead Letter Exchanges.
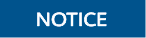
RabbitMQ dead letter messages may compromise performance. Exercise caution.
Configuring dead letter exchanges and routes using queue parameters
To configure a dead letter exchange for a queue, specify the x-dead-letter-exchange and x-dead-letter-routing-key parameters when creating the queue. The queue sends the dead letter message to the dead letter exchange based on x-dead-letter-exchange and sets the dead letter routing key for the dead letter message based on x-dead-letter-routing-key.
The following example shows how to configure a dead letter exchange and routing information on a Java client.
channel.exchangeDeclare("some.exchange.name", "direct"); Map<String, Object> args = new HashMap<String, Object>(); args.put("x-dead-letter-exchange", "some.exchange.name"); args.put("x-dead-letter-routing-key", "some-routing-key"); channel.queueDeclare("myqueue", false, false, false, args);
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot