Filtering Messages by Message Label
Overview
In an actual scenario, messages stored in a queue may contain different practical purposes. If the messages are not differentiated, consumers will pull messages in sequence until the consumption of all messages is complete.
If consumers are interested only in a certain type of messages, consumption of all messages will affect the processing efficiency.
Optimization Solution
DMS provides the message label capability. A producer can provide one or more labels for each message. Messages are filtered based on the label content to ensure that consumers only consume the message type that they are interested in.
For example, in a financial scenario, multiple types of messages may be generated in a transaction, such as a stock, fund, and loan. These messages are transferred to different processing systems, such as the stock system, fund system, loan system, and real-time analysis system, through business topics. However, the fund system concerns only messages of the fund type, and the real-time analysis system may need to obtain all types of messages.
When producing a message, the producer adds a label to each message. When the consumer pulls messages, the consumer determines whether to obtain only messages with a specific label. This improves the message consumption efficiency, as shown in Figure 1.
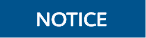
DMS standard queues and FIFO queues support the message label function. Kafka queues do not support this function.
Sample Code
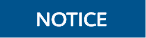
The following describes only the codes related to the message label. To run the entire demo, download the complete sample code package and refer to the DMS Development Guide for deploying and running the code.
The example provides code based on the HTTP RESTful interface. For details about the APIs, see the Distributed Message Service API Reference in Help Center.
Sample code for message label design:
package com.cloud.dms; import java.net.URL; import java.util.Properties; import com.cloud.dms.access.AccessServiceUtils; public class DMSHttpClient { private static String endpointUrl = ""; private static String region = ""; private static String serviceName = "dms"; private static String aKey = ""; private static String sKey = ""; private static String projectId = "546e52331ea74cd49722fda4fb23bf55"; private static String queueId = "39cd8dcb-b901-43b4-9ea1-48730e9adc58"; private static String queueGroupId = "g-ae8ed05f-464c-452c-9e37-d3bdd081000d"; /* * Read Configure File And Initialize Variables */ static { URL configPath = ClassLoader.getSystemResource("dms-service-config.properties"); Properties prop = AccessServiceUtils.getPropsFromFile(configPath.getFile()); region = prop.getProperty(Constants.DMS_SERVICE_REGION); aKey = prop.getProperty(Constants.DMS_SERVICE_AK); sKey = prop.getProperty(Constants.DMS_SERVICE_SK); endpointUrl = prop.getProperty(Constants.DMS_SERVICE_ENDPOINT_URL); if (endpointUrl.endsWith("/")) { endpointUrl = endpointUrl + "v1.0/"; } else { endpointUrl = endpointUrl + "/v1.0/"; } projectId = prop.getProperty(Constants.DMS_SERVICE_PROJECT_ID); } public static void main(String[] args) { runAllApiMethods(); } public static void runAllApiMethods() { MsgAttri msg = new MsgAttri(); msg.setaKey(aKey); msg.setEndpointUrl(endpointUrl); msg.setProjectId(projectId); msg.setQueueId(queueId); msg.setsKey(sKey); msg.setRegion(region); msg.setServiceName(serviceName); msg.setMsgLimit("10"); msg.setGroupId(queueGroupId); /** * * Construct producers and four types of consumers and set labels that they are interested in. */ MsgProducer msgProducer = new MsgProducer(msg); MsgConsumer stock = new MsgConsumer(msg, "stock"); MsgConsumer fund = new MsgConsumer(msg, "fund"); MsgConsumer loan = new MsgConsumer(msg, "loan"); MsgConsumer all = new MsgConsumer(msg, null); /** * Create threads, simulate production and consumption behaviors, and set thread names for differentiation. */ Thread producer = new Thread(msgProducer); Thread stockThread = new Thread(stock); Thread fundThread = new Thread(fund); Thread loanThread = new Thread(loan); Thread alls = new Thread(all); producer.setName("producer"); stockThread.setName("stock"); fundThread.setName("fund"); loanThread.setName("loan"); alls.setName("Analysis"); /** * Start threads. */ producer.start(); stockThread.start(); fundThread.start(); // loanThread.start(); // alls.start(); } }
Sample code for producing messages:
package com.cloud.dms; import static com.cloud.dms.ApiUtils.constructTempMessages; import static com.cloud.dms.ApiUtils.sendMessages; import java.util.concurrent.TimeUnit; public class MsgProducer implements Runnable{ private MsgAttri msgAttri; public MsgProducer(MsgAttri msg) { this.msgAttri = msg; } public void run() { while (true) { /** * Simulate producers to construct messages. JSON contains the stock, fund, and loan messages. **/ String messages = constructTempMessages(null); sendMessages(messages, this.msgAttri); try { TimeUnit.SECONDS.sleep(1); } catch (InterruptedException e) { } } } }
Sample code for consuming messages:
package com.cloud.dms; import static com.cloud.dms.ApiUtils.acknowledgeMessages; import static com.cloud.dms.ApiUtils.consumeMessages; import static com.cloud.dms.ApiUtils.parseHandlerIds; import java.util.ArrayList; import java.util.List; import java.util.concurrent.TimeUnit; public class MsgConsumer implements Runnable{ private MsgAttri msgAttri; private String tag; /** *Construct a function to obtain the label that consumers are interested in. **/ public MsgConsumer(MsgAttri msg, String tag) { this.tag = tag; this.msgAttri = msg; } public void run() { while (true) { /** *Consume messages and obtain messages with the label. **/ ResponseMessage consumeMessagesResMsg = consumeMessages(msgAttri, tag); /** *Parse messages. **/ if (consumeMessagesResMsg.getStatusCode() == 200) { List<String> msgStrings = ApiUtils.decodeMsg(consumeMessagesResMsg); /** *Simulate message processing and print messages with the label. **/ for (String s : msgStrings) { System.out.println("Thread--"+ Thread.currentThread().getName() + "--Message Body is: "+ s); } /** * Confirm message consumption. **/ ArrayList<String> handlerIds = parseHandlerIds(consumeMessagesResMsg); if (handlerIds.size() > 0) { acknowledgeMessages(handlerIds, msgAttri); } } else { System.out.println("Http Response Code is: " + consumeMessagesResMsg.getStatusCode() + "\n Http Body is: " + consumeMessagesResMsg.getBody()); } try { TimeUnit.SECONDS.sleep(2); } catch (InterruptedException e) { } } } }
Running Results of Sample Code
The loan thread has specified the tag (loan) and therefore can consume only messages with the loan label.
The fund and stock threads can consume only the specified messages.
The analysis thread does not specify a label and can consume all messages in the topic.
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot