更新时间:2024-10-21 GMT+08:00
Demo2
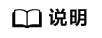
该Demo主要展示edge_driver.h里的回调函数和接口函数使用。
#include "edge_driver.h" #include <stdio.h> #include <string.h> #include <unistd.h> /** * 驱动Demo * Demo内容涉及函数函数,接口函数,Modbus Demo和other Demo * Modbus Demo和other Demo主要是展示调用有关接口 * ***************************************/ /****************************** 回调函数Demo ***************************************/ /** * 描述:子设备添加的回调 * 参数: * event_id: null * add_sub_devices_event:添加的子设备信息 */ EDGE_RETCODE sub_device_add_cb_1(const char* event_id, ST_ADD_SUB_DEVICES_EVENT* add_sub_devices_event) { int i; printf("add_sub_device_event payload shows below:\n"); printf("device's version:%lld\n", (long long)add_sub_devices_event->version); printf("devices:\n"); for(i = 0; i < add_sub_devices_event->device_len; i++) { printf(" {parent_device_id:%s, ", add_sub_devices_event->devices[i].parent_device_id); printf("node_id:%s, ", add_sub_devices_event->devices[i].node_id); printf("device_id:%s, ", add_sub_devices_event->devices[i].device_id); printf("name:%s, ", add_sub_devices_event->devices[i].name); printf("description:%s, ", add_sub_devices_event->devices[i].description); printf("product_id:%s, ", add_sub_devices_event->devices[i].product_id); printf("fw_version:%s, ", add_sub_devices_event->devices[i].fw_version); printf("sw_version:%s, ", add_sub_devices_event->devices[i].sw_version); printf("status:%s}\n", add_sub_devices_event->devices[i].status); } return EDGE_SUCCESS; } /** * 描述:子设备刪除的回调 * 参数: * event_id: null * delete_sub_devices_event:添加的子设备信息 */ EDGE_RETCODE sub_device_delete_cb_1(const char* event_id, ST_DELETE_SUB_DEVICES_EVENT* delete_sub_devices_event) { int i; printf("delete_sub_device_event payload shows below:\n"); printf("device's version:%lld\n", delete_sub_devices_event->version); printf("devices:\n"); for(i = 0; i < delete_sub_devices_event->device_len; i++) { printf(" {parent_device_id:%s, ", delete_sub_devices_event->devices[i].parent_device_id); printf("node_id:%s, ", delete_sub_devices_event->devices[i].node_id); printf("device_id:%s, ", delete_sub_devices_event->devices[i].device_id); printf("name:%s, ", delete_sub_devices_event->devices[i].name); printf("description:%s, ", delete_sub_devices_event->devices[i].description); printf("product_id:%s, ", delete_sub_devices_event->devices[i].product_id); printf("fw_version:%s, ", delete_sub_devices_event->devices[i].fw_version); printf("sw_version:%s, ", delete_sub_devices_event->devices[i].sw_version); printf("status:%s}\n", delete_sub_devices_event->devices[i].status); } return EDGE_SUCCESS; } /** * 描述:针对子设备的命令的回调函数 * 参数: * command_name:命令名称 * device_id:子设备Id * service_id:服务Id * request_id:请求Id(响应命令) * body:命令体 * body_len:命令体长度 */ EDGE_RETCODE deviceCmdCb(const char* command_name, const char* device_id, const char* service_id, const char* request_id, const char* body, unsigned int body_len) { printf("command_name:%s, device_id:%s, service_id:%s, request_id:%s, body:%s, body_len:%d", command_name, device_id, service_id, request_id, body, body_len); char* rsp = "{\"error_desc\":\"ok\"}"; edge_send_command_rsp(NULL, request_id, 0, rsp, strlen(rsp)); return EDGE_SUCCESS; } /** * 描述:子设备事件的回调 * 参数: * device_id:子设备Id * body:事件体 * len:长度 */ EDGE_RETCODE sub_device_event_cb(const char* device_id, const char* body, unsigned int body_len) { printf("%s\n", device_id); printf("%s", body); return EDGE_SUCCESS; } /** * 描述:子设备start_scan事件的回调 * 参数: * protocol:协议 * channel:通道 * parentDeviceId:父设备Id * scan_setting:扫描设置 * body_len:长度 */ EDGE_RETCODE sub_device_start_scan_cb(const char* protocol, const char* channel, const char* parentDeviceId,const char* scan_setting, unsigned int body_len) { printf("protocol = %s, channel = %s, parentDeviceId = %s, scan_setting = %s", protocol, channel, parentDeviceId, scan_setting); return EDGE_SUCCESS; } /** * 描述:子设备消息的回调 * 参数: * message_id:消息Id * channel:通道 * device_id:设备Id * body:消息体 * body_len:长度 */ EDGE_RETCODE sub_device_messages_down_cb(const char* message_id, const char* message_name, const char* device_id, const char* body, unsigned int body_len) { printf("message_id = %s, message_name = %s, device_id = %s, body = %s", message_id, message_name, device_id, body); return EDGE_SUCCESS; } /** * 描述:子设备收到属性设置的回调 * 参数: * sub_device_property_set:属性设置的具体数据 */ EDGE_RETCODE sub_device_properties_set_cb(ST_PROPERTY_SET* sub_device_property_set) { printf("object_device_id = %s, request_id = %s\n", sub_device_property_set->object_device_id, sub_device_property_set->request_id); printf("services_size = %d\n", sub_device_property_set->services_size); int i; for(i = 0; i< sub_device_property_set->services_size; i++) { printf("service_id = %s, properties = %s\n", sub_device_property_set->services[i].service_id, sub_device_property_set->services[i].properties); } ST_IOT_RESULT result; result.result_desc = "ok"; result.result_code = "0"; edge_send_sub_device_property_set_rsp(sub_device_property_set->request_id, &result); return EDGE_SUCCESS; } /** * 描述:子设备属性获取的回调 * 参数: * sub_device_property_get:获取到的子设备属性 */ EDGE_RETCODE sub_device_properties_get_cb(ST_PROPERTY_GET* sub_device_property_get) { printf("object_device_id = %s, request_id = %s, service_id = %s", sub_device_property_get->object_device_id, sub_device_property_get->request_id, sub_device_property_get->service_id); char* data_body1 = "{\n" "\t\"PhV_phsA\":1,\n" "\t\"PhV_phsB\":2\n" "}"; ST_DEVICE_PROPERTY_GET_RSP device_property_get_rsp; ST_SERVICE_DATA service_data_1 = {0}; service_data_1.service_id = "service_id_1"; service_data_1.properties = data_body1; service_data_1.event_time = "20200520T115630Z"; device_property_get_rsp.services = &service_data_1; device_property_get_rsp.services_size = 1; edge_send_sub_device_property_get_rsp(sub_device_property_get->request_id, &device_property_get_rsp); return EDGE_SUCCESS; } /** * 描述:获取子设备影子的回调 * 参数: * sub_device_shadow:子设备影子 */ EDGE_RETCODE sub_device_shadow_cb(ST_DEVICE_SHADOW* sub_device_shadow) { printf("object_device_id = %s, request_id = %s\n", sub_device_shadow->object_device_id, sub_device_shadow->request_id); printf("services_size = %d\n", sub_device_shadow->shadow_size); int i; for(i = 0; i< sub_device_shadow->shadow_size; i++) { printf("service_id = %s, properties = %s\n", sub_device_shadow->shadow[i].service_id, sub_device_shadow->shadow[i].desired_properties); } return EDGE_SUCCESS; } /** * 描述:子设备请求响应的回调 * 参数: * getProductsRspEvent:获取到的子设备请求响应信息 */ EDGE_RETCODE get_products_response_cb(ST_GET_PRODUCTS_RSP_EVENT* getProductsRspEvent) { int i, j, k, l, m; for (i = 0; i < getProductsRspEvent->product_len; i++) { printf("%d-th product's info shows below:\n", i+1); printf("product_id:%s\n", getProductsRspEvent->products[i].product_id); printf("name:%s\n", getProductsRspEvent->products[i].name); printf("device_type:%s\n", getProductsRspEvent->products[i].device_type); printf("protocol_type:%s\n", getProductsRspEvent->products[i].protocol_type); printf("data_format:%s\n", getProductsRspEvent->products[i].data_format); printf("industry:%s\n", getProductsRspEvent->products[i].industry); printf("name:%s\n", getProductsRspEvent->products[i].name); printf("service_capabilities:\n"); for (j = 0; j < getProductsRspEvent->products[i].service_capability_len; j++) { printf(" service_id:%s, ", getProductsRspEvent->products[i].serviceCapabilities[j].service_id); printf("service_type:%s, ", getProductsRspEvent->products[i].serviceCapabilities[j].service_type); printf("description:%s, ", getProductsRspEvent->products[i].serviceCapabilities[j].description); printf("option:%s\n", getProductsRspEvent->products[i].serviceCapabilities[j].option); printf(" properties:\n"); for(k = 0; k < getProductsRspEvent->products[i].serviceCapabilities[j].property_len; k++) { printf(" property_name:%s, ", getProductsRspEvent->products[i].serviceCapabilities[j].properties[k].property_name); printf("required:%s, ", getProductsRspEvent->products[i].serviceCapabilities[j].properties[k].required ? "true" : "false"); printf("data_type:%s, ", getProductsRspEvent->products[i].serviceCapabilities[j].properties[k].data_type); printf("enum_list:%s, ", getProductsRspEvent->products[i].serviceCapabilities[j].properties[k].enum_list); printf("min:%s, ", getProductsRspEvent->products[i].serviceCapabilities[j].properties[k].min); printf("max:%s, ", getProductsRspEvent->products[i].serviceCapabilities[j].properties[k].max); printf("max_length:%d, ", getProductsRspEvent->products[i].serviceCapabilities[j].properties[k].max_length); printf("step:%lf, ", getProductsRspEvent->products[i].serviceCapabilities[j].properties[k].step); printf("unit:%s, ", getProductsRspEvent->products[i].serviceCapabilities[j].properties[k].unit); printf("method:%s, ", getProductsRspEvent->products[i].serviceCapabilities[j].properties[k].method); printf("description:%s, ", getProductsRspEvent->products[i].serviceCapabilities[j].properties[k].description); printf("default_value:%s\n", getProductsRspEvent->products[i].serviceCapabilities[j].properties[k].default_value); } printf(" commands:\n"); for(k = 0; k < getProductsRspEvent->products[i].serviceCapabilities[j].command_len; k++) { printf(" command_name:%s\n", getProductsRspEvent->products[i].serviceCapabilities[j].commands[k].command_name); printf(" paras:\n"); for(l = 0; l < getProductsRspEvent->products[i].serviceCapabilities[j].commands[k].para_len; l++) { printf(" para_name:%s, ", getProductsRspEvent->products[i].serviceCapabilities[j].commands[k].paras[l].para_name); printf("required:%s, ", getProductsRspEvent->products[i].serviceCapabilities[j].commands[k].paras[l].required ? "true" : "false"); printf("data_type:%s, ", getProductsRspEvent->products[i].serviceCapabilities[j].commands[k].paras[l].data_type); printf("enum_list:%s, ", getProductsRspEvent->products[i].serviceCapabilities[j].commands[k].paras[l].enum_list); printf("min:%s, ", getProductsRspEvent->products[i].serviceCapabilities[j].commands[k].paras[l].min); printf("max:%s, ", getProductsRspEvent->products[i].serviceCapabilities[j].commands[k].paras[l].max); printf("max_length:%d, ", getProductsRspEvent->products[i].serviceCapabilities[j].commands[k].paras[l].max_length); printf("step:%lf, ", getProductsRspEvent->products[i].serviceCapabilities[j].commands[k].paras[l].step); printf("unit:%s, ", getProductsRspEvent->products[i].serviceCapabilities[j].commands[k].paras[l].unit); printf("description:%s\n", getProductsRspEvent->products[i].serviceCapabilities[j].commands[k].paras[l].description); } printf(" responses:\n"); for(l = 0; l < getProductsRspEvent->products[i].serviceCapabilities[j].commands[k].response_len; l++) { printf(" response_name:%s\n", getProductsRspEvent->products[i].serviceCapabilities[j].commands[k].responses[l].response_name); printf(" paras:\n"); for(m = 0; m < getProductsRspEvent->products[i].serviceCapabilities[j].commands[k].responses[l].service_command_para_len; m++) { printf(" para_name:%s, ", getProductsRspEvent->products[i].serviceCapabilities[j].commands[k].responses[l].paras[m].para_name); printf("required:%s, ", getProductsRspEvent->products[i].serviceCapabilities[j].commands[k].responses[l].paras[m].required ? "true" : "false"); printf("data_type:%s, ", getProductsRspEvent->products[i].serviceCapabilities[j].commands[k].responses[l].paras[m].data_type); printf("enum_list:%s, ", getProductsRspEvent->products[i].serviceCapabilities[j].commands[k].responses[l].paras[m].enum_list); printf("min:%s, ", getProductsRspEvent->products[i].serviceCapabilities[j].commands[k].responses[l].paras[m].min); printf("max:%s, ", getProductsRspEvent->products[i].serviceCapabilities[j].commands[k].responses[l].paras[m].max); printf("max_length:%d, ", getProductsRspEvent->products[i].serviceCapabilities[j].commands[k].responses[l].paras[m].max_length); printf("step:%lf, ", getProductsRspEvent->products[i].serviceCapabilities[j].commands[k].responses[l].paras[m].step); printf("unit:%s, ", getProductsRspEvent->products[i].serviceCapabilities[j].commands[k].responses[l].paras[m].unit); printf("description:%s\n", getProductsRspEvent->products[i].serviceCapabilities[j].commands[k].responses[l].paras[m].description); } } } } printf("\n"); } return EDGE_SUCCESS; }
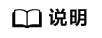
以下是接口函数部分
/****************************** 接口函数Demo ***************************************/ /** * 上报子设备属性数据 */ EDGE_RETCODE send_batch_device_data() { /* * 具体上报的子设备数据和设备的产品模型有关系,和属性对应 * */ char* data_body1 = "{\n" "\t\"PhV_phsA\":1,\n" "\t\"PhV_phsB\":2\n" "}"; char* data_body2 = "{\"PhV_phsA\":\"1\",\"PhV_phsB\":\"2\"}"; printf("data_body1:%s\n", data_body1); printf("data_body2:%s\n", data_body2); ST_DEVICE_SERVICE device_data[2]; ST_DEVICE_SERVICE devcie_data_1 = {0}; ST_DEVICE_SERVICE devcie_data_2 = {0}; ST_SERVICE_DATA service_data[2]; ST_SERVICE_DATA service_data_1 = {0}; service_data_1.service_id = "service_id_1"; service_data_1.properties = data_body1; service_data_1.event_time = "20200520T115630Z"; ST_SERVICE_DATA service_data_2 = {0}; service_data_2.service_id = "service_id_2"; service_data_2.properties = data_body2; service_data_2.event_time = "20200520T115630Z"; service_data[0] = service_data_1; service_data[1] = service_data_2; devcie_data_1.services = service_data; devcie_data_1.device_id = "device_id_1"; devcie_data_1.size = 2; devcie_data_2.services = service_data; devcie_data_2.device_id = "device_id_2"; devcie_data_2.size = 2; device_data[0] = devcie_data_1; device_data[1] = devcie_data_2; ST_SUB_DEVICES_PROPERTIES_REPORT subDevicesPropertiesReport = {0}; subDevicesPropertiesReport.device_size = 2; subDevicesPropertiesReport.devices = device_data; return edge_driver_report_sub_device_properties(&subDevicesPropertiesReport); } /** * 描述:获取设备影子事件示例 */ void get_device_shadow() { ST_DEVICE_SHADOW_GET device_shadow_get = {0}; device_shadow_get.service_id = "service1"; device_shadow_get.object_device_id = "deviceId1"; char* request_id = "123"; edge_driver_get_device_shadow(request_id, &device_shadow_get); } /** * 描述:获取产品示例 * */ void get_products() { char* product_ids[10] = {0}; product_ids[0] = "1234"; product_ids[1] = "1234"; edge_driver_get_products(product_ids, 2); } /** * 描述:发送设备事件示例 */ void send_sub_device_event() { ST_DEVICE_EVENT device_event = {0}; device_event.object_device_id = "deviceId1"; ST_SERVICE_EVENT service_event = {0}; service_event.service_id = "message_name"; service_event.event_id = "message_id"; service_event.event_type = "message_name"; service_event.paras = "{\n" "\t\"module_id\": \"module_id\",\n" "\t\"old_status\": \"STOPPED|RUNNING|UNHEALTHY\",\n" "\t\"new_status\": \"STOPPED|RUNNING|UNHEALTHY\"\n" "}"; service_event.paras_len = (int)strlen(service_event.paras); device_event.services = &service_event; device_event.services_size = 1; edge_driver_send_device_event(&device_event); } /** * 描述:发送设备消息示例 */ void send_sub_device_message() { ST_DEVICE_MESSAGE device_message = {0}; device_message.object_device_id = "deviceId1"; device_message.name = "message_name"; device_message.id = "message_id"; device_message.content = "{\n" "\t\"module_id\": \"module_id\",\n" "\t\"old_status\": \"STOPPED|RUNNING|UNHEALTHY\",\n" "\t\"new_status\": \"STOPPED|RUNNING|UNHEALTHY\"\n" "}"; device_message.content_len = (int)strlen(device_message.content); edge_driver_send_device_message(&device_message); } /** * 描述:更新子设备状态方式示例 * */ void update_sub_device_status(){ /* * 构造 {"device_statuses":[{ * "device_id":"deviceId", * "status":"status" * }]} * */ ST_DEVICE_STATUS device_status[2]; ST_DEVICE_STATUS device_status_1 = {0}; device_status_1.device_id = "device_id_1"; device_status_1.status = "RUNNING"; device_status[0] = device_status_1; ST_DEVICE_STATUS device_status_2 = {0}; device_status_2.device_id = "device_id_2"; device_status_2.status = "RUNNING"; device_status[1] = device_status_2; const char* event_id = "event_id1"; edge_driver_update_sub_devices_status(event_id, device_status, 2); } /** * 描述:同步子设备方式示例 */ void sync_sub_devices() { const char* event_id = "event_id1"; long long version = 123; edge_driver_sync_sub_devices(event_id, version); } /** * 描述:发送服务事件方式示例 */ void send_service_event() { char* body = "{\n" "\t\"module_id\": \"module_id\",\n" "\t\"old_status\": \"STOPPED|RUNNING|UNHEALTHY\",\n" "\t\"new_status\": \"STOPPED|RUNNING|UNHEALTHY\"\n" "}"; edge_driver_send_service_event("module_management", "module_status_change", body, strlen(body)); }
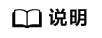
下面是Modbus Demo和其他Demo,里面包括调用上面所有的回调函数和接口函数,用不到的地方注释掉即可。
/****************************** Modbus Demo和其他Demo ***************************************/ /** * modbus demo */ void modbus_driver_demo() { EDGE_RETCODE ret; // 设置定时任务 while(1) { ret = send_batch_device_data(); if (EDGE_SUCCESS != ret) { printf("failed to report sub device data.\n"); } // 每1000秒重复上报数据 sleep(1000); } } /** * 其他demo,可根据需要自行选择 */ void other_demo() { edge_driver_get_shadow(); send_service_event(); sleep(5); get_device_shadow(); sleep(1); get_products(); sleep(1); send_sub_device_event(); sleep(1); send_sub_device_message(); sleep(1); update_sub_device_status(); sleep(1); sync_sub_devices(); // 这里是为了使应用能够长时间运行 while (1) { sleep(1000); } } void driver_demo() { // 禁用缓冲区 setvbuf(stdout, NULL, _IONBF, 0); //初始化sdk,工作路径设置(工作路径下需要含有 /conf 目录(该目录下包含证书等信息)) edge_driver_init("../code/api_test/workdir"); printf("driver demo start.\n"); // 请根据需要选择相应的回调处理函数 ST_GATEWAY_CBS gateway_cbs = {0}; gateway_cbs.pfn_sub_device_add_cb_1 = sub_device_add_cb_1; gateway_cbs.pfn_sub_device_deleted_cb_1 = sub_device_delete_cb_1; gateway_cbs.pfn_device_command_cb = deviceCmdCb; gateway_cbs.pfn_device_event_cb = sub_device_event_cb; gateway_cbs.pfn_on_start_scan_cb = sub_device_start_scan_cb; gateway_cbs.pfn_device_message_cb = sub_device_messages_down_cb; gateway_cbs.pfn_on_get_products_rsp_cb = get_products_response_cb; gateway_cbs.pfn_device_properties_set_cb = sub_device_properties_set_cb; gateway_cbs.pfn_device_properties_get_cb = sub_device_properties_get_cb; gateway_cbs.pfn_device_shadow_cb = sub_device_shadow_cb; //设置网关回调函数(无需全部设置,按需设置需要接收的回调) edge_driver_set_gateway_callback(&gateway_cbs); //连接hub edge_driver_login(); sleep(10); // 自定义demo, 请根据需要替换或修改 // 如果不需要,请注释掉 modbus_driver_demo(); // 如果不需要,请注释掉 other_demo(); edge_driver_logout(); sleep(1000); edge_driver_destroy(); } int main() { driver_demo(); return 0; }
父主题: ModuleSDK-C Demo展示