Java SDK使用指导
MSGSMS提供Java语言的应用侧SDK供开发者使用,您可以直接集成SDK来调用MSGSMS的短信发送API,从而实现对MSGSMS的快速操作。
开发时序图
开发前准备
获取和安装SDK
您可以通过Maven方式获取和安装SDK,首先需要在您的操作系统中下载并安装Maven ,安装完成后您只需要在Java项目的pom.xml文件中加入相应的依赖项即可。
使用SDK前,您需要安装“huaweicloud-sdk-smsapi”,具体的SDK版本号请参见SDK开发中心。
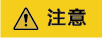
以下代码示例中的version值,请根据实际的SDK版本号进行替换。
<dependency> <groupId>com.huaweicloud.sdk</groupId> <artifactId>huaweicloud-sdk-smsapi</artifactId> <version>3.1.125</version> </dependency>
代码示例
package com.huawei.smsapi; import com.huaweicloud.sdk.core.auth.ICredential; import com.huaweicloud.sdk.core.exception.ConnectionException; import com.huaweicloud.sdk.core.exception.RequestTimeoutException; import com.huaweicloud.sdk.core.exception.ServiceResponseException; import com.huaweicloud.sdk.core.http.HttpConfig; import com.huaweicloud.sdk.core.region.Region; import com.huaweicloud.sdk.smsapi.v1.SMSApiClient; import com.huaweicloud.sdk.smsapi.v1.SMSApiCredentials; import com.huaweicloud.sdk.smsapi.v1.model.BatchSendDiffSmsRequest; import com.huaweicloud.sdk.smsapi.v1.model.BatchSendDiffSmsRequestBody; import com.huaweicloud.sdk.smsapi.v1.model.BatchSendDiffSmsResponse; import com.huaweicloud.sdk.smsapi.v1.model.BatchSendSmsRequest; import com.huaweicloud.sdk.smsapi.v1.model.BatchSendSmsRequestBody; import com.huaweicloud.sdk.smsapi.v1.model.BatchSendSmsResponse; import com.huaweicloud.sdk.smsapi.v1.model.SmsContent; import java.util.ArrayList; import java.util.Arrays; /** * Use the SDK interface to send an SMS message. * Before using this example, you need to create an SMS application, signature, * and template through the console or by invoking the open APIs of the MSGSMS. */ public class SendSms { public static void main(String[] args) { System.out.println("Start HUAWEI CLOUD MSGSMS SEND SMS Java Demo..."); /* * Send an SMS message using a special AK/SK authentication algorithm. * When the MSGSMS is used to send SMS messages, the AK is app_key, and the SK is app_secret. * There will be security risks if the app_key/app_secret used for authentication is directly written into code. * We suggest encrypting the app_key/app_secret in the configuration file or environment variables for storage. * In this sample, the app_key/app_secret is stored in environment variables for identity authentication. * Before running this sample, set the environment variables CLOUD_SDK_MSGSMS_APPKEY and CLOUD_SDK_MSGSMS_APPSECRET. * CLOUD_SDK_MSGSMS_APPKEY indicates the application key (app_key), and CLOUD_SDK_MSGSMS_APPSECRET indicates the application secret (app_secret). * You can obtain the value from Application Management on the console or by calling the open API of Application Management. */ String ak = System.getenv("CLOUD_SDK_MSGSMS_APPKEY"); String sk = System.getenv("CLOUD_SDK_MSGSMS_APPSECRET"); /* Creating an SmsApiClient Instance * current support region: * CN North-Beijing4: RegionId: cn-north-4, endpoint: https://smsapi.cn-north-4.myhuaweicloud.com:8443 * CN South-Guangzhou: RegionId: cn-south-1, endpoint: https://smsapi.cn-south-1.myhuaweicloud.com:8443 */ SMSApiClient client = getClient(new Region("cn-north-4", new String[]{"https://smsapi.cn-north-4.myhuaweicloud.com:8443"}), getCredential(ak, sk)); try { // Example 1: Use POST /sms/batchSendSms/v1 to send an SMS message. System.out.println("----Example 1 use POST /sms/batchSendSms/v1 send msg now."); int httpcode = batchSendSms(client); System.out.println((httpcode == 200) ? "----Example 1 send sms success." : "----Example 1 send sms failed."); // Example 2: Using the POST /sms/batchSendDiffSms/v1 to Send SMS Messages in Batches System.out.println("----Example 2 use POST /sms/batchSendDiffSms/v1 send msg now."); httpcode = batchSendDiffSms(client); System.out.println((httpcode == 200) ? "----Example 2 send sms success." : "----Example 2 send sms failed."); } catch (ConnectionException | RequestTimeoutException e) { System.out.println(e.getMessage()); } catch (ServiceResponseException e) { System.out.println(e.getHttpStatusCode()); System.out.println(e.getErrorCode()); System.out.println(e.getErrorMsg()); } } /** * Sending SMS Messages * * @param client SMSApiClient Instance * @return Indicates whether the SMS message is sent successfully. */ private static int batchSendSms(SMSApiClient client) { // Construct a request for sending an SMS message. BatchSendSmsRequest request = new BatchSendSmsRequest(); request.withBody(new BatchSendSmsRequestBody() // Channel ID for sending SMS messages. .withFrom("8824110605***") // List of numbers that receive SMS messages. // Note: When there are multiple numbers, do not contain spaces between the numbers. .withTo("+86137****3774,+86137****3776") // Template Id .withTemplateId("e1440669a4354ccdb56ebf2283c6***") // Template parameter, which must be enclosed in square brackets ([]). .withTemplateParas("[\"12\",\"23\",\"e\"]") // Status report callback URL. Set this parameter to a valid value. If status reports are not required, you do not need to set this parameter. .withStatusCallback("https://test/report")); // Invoke the SDK interface to send an SMS message. BatchSendSmsResponse response = client.batchSendSms(request); // Print the response result. System.out.println(response.toString()); return response.getHttpStatusCode(); } /** * Sending SMS Messages in Batches * * @param client SMSApiClient Instance * @return Whether the batch SMS message is sent successfully. */ private static int batchSendDiffSms(SMSApiClient client) { //Construct a request for sending SMS messages in batches. BatchSendDiffSmsRequest request = new BatchSendDiffSmsRequest(); request.withBody(new BatchSendDiffSmsRequestBody() // Channel ID for sending SMS messages. .withFrom("8824110605***") // Status report callback URL. Set this parameter to a valid value. If status reports are not required, you do not need to set this parameter. .withStatusCallback("https://test/report") .withSmsContent(new ArrayList<>(Arrays.asList( // Content of the first batch of group SMS messages new SmsContent() // List of SMS Recipient Numbers .withTo(new ArrayList<>((Arrays.asList("+86137****3774", "+86137****3775")))) // Template Id .withTemplateId("cefada4b8eaa4835864eb5b5eae1****") // Template Parameters .withTemplateParas(new ArrayList<>(Arrays.asList("1", "23", "45"))), // Content of the second batch of group SMS messages new SmsContent() // List of SMS Recipient Numbers .withTo(new ArrayList<>((Arrays.asList("+86137****3777", "+86137****3778")))) // Template Id .withTemplateId("e1440669a4354ccdb56ebf2283c6****") // Template Parameters .withTemplateParas(new ArrayList<>(Arrays.asList("3", "4", "5"))))))); //Invoke the SDK interface to send SMS messages in batche BatchSendDiffSmsResponse response = client.batchSendDiffSms(request); // Print the response result. System.out.println(response.toString()); return response.getHttpStatusCode(); } /** * Creating an Authentication Credential * * @param ak Application key (AK) generated after an app is created for the SMS service. * @param sk Application Secret (SK) generated after an app is created for the SMS service. * @return Authentication credential */ private static SMSApiCredentials getCredential(String ak, String sk) { return new SMSApiCredentials() .withAk(ak) .withSk(sk); } /** * Create SMSApiClient Instance * * @param region region * @param auth Authentication credential * @return SMSApiClient Instance */ private static SMSApiClient getClient(Region region, ICredential auth) { // Use the default configuration. HttpConfig config = HttpConfig.getDefaultHttpConfig(); config.withIgnoreSSLVerification(true); // Initializing the Client of the SMSApi Service return SMSApiClient.newBuilder() .withHttpConfig(config) .withCredential(auth) .withRegion(region) .build(); } }
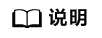
发送短信SDK默认使用的是okhttp组件发送消息,最大链接数为5;线程池使用ForkJoinPool,默认线程池与服务器的CPU数一样。这两个参数会限制系统的最大并发数,如果出现时延变长、性能无法提升时,可重定义HttpConfig的这两个参数以提升系统性能,例如:
HttpConfig config = HttpConfig.getDefaultHttpConfig(); config.withConnectionPool(new ConnectionPool(50, 5L, TimeUnit.MINUTES)); config.withExecutorService(new ForkJoinPool(200));
运行结果
- 发送短信(BatchSendSms)
```json class BatchSendSmsResponse { code: 000000 description: Success result: [class SmsID { createTime: 2024-11-22T02:16:46Z from: 8824110605*** originTo: +86137****3774 smsMsgId: eb4e63dd-0945-4231-a48d-6b54c1a8b614_3434030 status: 000000 countryId: CN total: 1 }, class SmsID { createTime: 2024-11-22T02:16:46Z from: 8824110605*** originTo: +86137****3776 smsMsgId: eb4e63dd-0945-4231-a48d-6b54c1a8b614_3434031 status: 000000 countryId: CN total: 1 }] } ```
- 发送分批短信(BatchSendDiffSms)
```json class BatchSendDiffSmsResponse { code: 000000 description: Success result: [class SmsID { createTime: 2024-11-22T02:16:46Z from: 8824110605*** originTo: +86137****3774 smsMsgId: eb4e63dd-0945-4231-a48d-6b54c1a8b614_3435044 status: 000000 countryId: CN total: 0 }, class SmsID { createTime: 2024-11-22T02:16:46Z from: 8824110605*** originTo: +86137****3775 smsMsgId: eb4e63dd-0945-4231-a48d-6b54c1a8b614_3435045 status: 000000 countryId: CN total: 0 }, class SmsID { createTime: 2024-11-22T02:16:46Z from: 8824110605*** originTo: +86137****3777 smsMsgId: eb4e63dd-0945-4231-a48d-6b54c1a8b614_3435046 status: 000000 countryId: CN total: 1 }, class SmsID { createTime: 2024-11-22T02:16:46Z from: 8824110605*** originTo: +86137****3778 smsMsgId: eb4e63dd-0945-4231-a48d-6b54c1a8b614_3435047 status: 000000 countryId: CN total: 1 }] } ```