Custom Topics Not Starting with $oc
Process
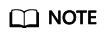
- By default, communications can be performed based on all custom topics not starting with $oc. The system_default_policy policy is added to newly created resource spaces by default, allowing all associated devices to publish or subscribe to messages through all topics. You can delete the policy if necessary.
- Policies are only used for communications with custom topics that do not start with $oc. For custom topics starting with $oc, their permissions are determined by product settings.
- Policies are not available in the following regions: CN South-Guangzhou, CN North-Beijing4, and CN East-Shanghai1.
- Create a product and create a device on the platform.
- Create a policy to control the topics subscription/publishing permissions. (optional)
- Go to the policy page. Access the IoTDA service page and click Access Console. Click the target instance card. In the navigation pane, choose .
Figure 2 Device policy - Access page
- Create a policy. Click Create Policy, set policy parameters, and click Generate. The following figure shows how to publish and subscribe to messages through topic /v1/test/hello.
Table 1 Parameters Parameter Description
Resource Space
Select a resource space from the drop-down list box or create one.
Policy Name
Customize a value, for example, PolicyTest. Max: 128 characters. Use only letters, digits, underscores (_), and hyphens (-).
Resource
For MQTT topic publishing and subscription, topic: must be used as the parameter prefix. For example, to forbid the subscription to /test/v1, set this parameter to topic:/test/v1.
Operation
Options: Publish and Subscribe, meaning the topic publishing and subscription requests of MQTT devices.
Permission
Options: Allowed and Denied, meaning whether the permission to publish or subscribe to messages through a topic is assigned.
- Go to the policy page. Access the IoTDA service page and click Access Console. Click the target instance card. In the navigation pane, choose .
- Bind the policy target. A policy can be bound to resource spaces, products, or devices. The bound devices are allowed or disallowed to publish or subscribe to messages through a specific topic accordingly. (optional)
Figure 3 Device policy - Binding a device
Table 2 Parameters Parameter Description
Target Type
You can set resource spaces, products, or devices as the target type. The three types can coexist. For example, product A and device C (under product B) can be bound to the same policy.
- Resource space: used for domain-based management of multiple service applications. After a resource space is bound to a policy, all devices in this resource space adopt the policy. You can also select multiple resource spaces for binding.
- Product: Generally, a product has multiple devices. After a product is bound to a policy, all devices of this product adopt the policy. Compared with the resource space, the binding scope is smaller. You can select products in different resource spaces for binding.
- Device: minimum unit for the target bound to a policy. You can select devices from different resource spaces and products for binding.
Target
After you select a policy target type, available targets are displayed in the Target area. Select targets as required.
- Use the device to subscribe to or publish messages through the specified topic. Only custom topics successfully bound in the policy can be used.
Java SDK Usage on the Device Side
Devices can integrate the device SDKs provided by Huawei Cloud IoT to quickly connect to Huawei Cloud IoTDA and report messages. The following example uses the Java SDK to connect a device to IoTDA for publishing and subscribing to messages through the custom topic /test/deviceToCloud.
- Configure the Maven dependency of the SDK on the device.
<dependency> <groupId>com.huaweicloud</groupId> <artifactId>iot-device-sdk-java</artifactId> <version>1.1.4</version> </dependency>
- Configure the SDK and device connection parameters on the device.
// Load the CA certificate of the IoT platform. For details about how to obtain the certificate, visit https://support.huaweicloud.com/intl/en-us/devg-iothub/iot_02_1004.html. URL resource = MessageSample.class.getClassLoader().getResource("ca.jks"); File file = new File(resource.getPath()); // The format is ssl://Domain name:Port number. // To obtain the domain name, log in to the Huawei Cloud IoTDA console. In the navigation pane, choose Overview and click Access Details in the Instance Information area. Select the access domain name corresponding to port 8883. String serverUrl = "ssl://localhost:8883"; // Device ID created on the IoT platform String deviceId = "deviceId"; // Secret corresponding to the device ID String deviceSecret = "secret"; // Initialize the device connection. IoTDevice device = new IoTDevice(serverUrl, deviceId, deviceSecret, file); if (device.init() != 0) { return; }
- Report a device message.
device.getClient().publishRawMessage(new RawMessage("/test/deviceToCloud", "hello", 1), new ActionListener() { @Override public void onSuccess(Object context) { System.out.println("reportDeviceMessage success: "); } @Override public void onFailure(Object context, Throwable var2) { System.out.println("reportDeviceMessage fail: " + var2); } });
- Subscribe to the topic.
device.getClient().subscribeTopic(new RawMessage("/test/deviceToCloud", new ActionListener() { @Override public void onSuccess(Object context) { System.out.println("subscribeTopic success: "); } @Override public void onFailure(Object context, Throwable var2) { System.out.println("subscribeTopic fail: " + var2); } }, 0);
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot