更新时间:2024-11-14 GMT+08:00
C#
样例 |
|
---|---|
环境要求 |
示例1:要求.NET Core 2.0及以上版本或.NET Framework 4.6及以上版本。 示例2:要求.NET Core 1.0及以上版本或.NET Framework 2.0及以上版本。 |
引用库 |
Newtonsoft.Json 11.0.2及以上版本,请参考https://www.newtonsoft.com/json获取。 |
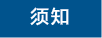
- 发送短信为单模板群发短信示例,发送分批短信为多模板群发短信示例。
- 本文档所述Demo在提供服务的过程中,可能会涉及个人数据的使用,建议您遵从国家的相关法律采取足够的措施,以确保用户的个人数据受到充分的保护。
- 本文档所述Demo仅用于功能演示,不允许客户直接进行商业使用。
- 本文档信息仅供参考,不构成任何要约或承诺。
发送短信(示例1)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 |
using System; using System.Collections.Generic; using System.Net; using System.Net.Http; using System.Security.Cryptography; using System.Text; namespace msgsms_csharp_demo { class SendSms { static void Main(string[] args) { //必填,请参考"开发准备"获取如下数据,替换为实际值 string apiAddress = "https://smsapi.cn-north-4.myhuaweicloud.com:443/sms/batchSendSms/v1"; //APP接入地址(在控制台"应用管理"页面获取)+接口访问URI // 认证用的appKey和appSecret硬编码到代码中或者明文存储都有很大的安全风险,建议在配置文件或者环境变量中密文存放,使用时解密,确保安全; string appKey = "c8RWg3ggEcyd4D3p94bf3Y7x1Ile"; //APP_Key string appSecret = "q4Ii87Bh************80SfD7Al"; //APP_Secret string sender = "csms12345678"; //国内短信签名通道号 string templateId = "8ff55eac1d0b478ab3c06c3c6a492300"; //模板ID //条件必填,国内短信关注,当templateId指定的模板类型为通用模板时生效且必填,必须是已审核通过的,与模板类型一致的签名名称 //string signature = "华为云短信测试"; //签名名称 //必填,全局号码格式(包含国家码),示例:+86151****6789,多个号码之间用英文逗号分隔 string receiver = "+86151****6789,+86152****7890"; //短信接收人号码 //选填,短信状态报告接收地址,推荐使用域名,为空或者不填表示不接收状态报告 string statusCallBack = ""; /* * 选填,使用无变量模板时请赋空值 string templateParas = ""; * 单变量模板示例:模板内容为"您的验证码是${1}"时,templateParas可填写为"[\"369751\"]" * 双变量模板示例:模板内容为"您有${1}件快递请到${2}领取"时,templateParas可填写为"[\"3\",\"人民公园正门\"]" * 模板中的每个变量都必须赋值,且取值不能为空 * 查看更多模板规范和变量规范:产品介绍>短信模板须知和短信变量须知 */ string templateParas = "[\"369751\"]"; //模板变量,此处以单变量验证码短信为例,请客户自行生成6位验证码,并定义为字符串类型,以杜绝首位0丢失的问题(例如:002569变成了2569)。 try { //为防止因HTTPS证书认证失败造成API调用失败,需要先忽略证书信任问题 HttpClient client = new HttpClient(); ServicePointManager.ServerCertificateValidationCallback = delegate { return true; }; //请求Headers client.DefaultRequestHeaders.Add("Authorization", "WSSE realm=\"SDP\",profile=\"UsernameToken\",type=\"Appkey\""); client.DefaultRequestHeaders.Add("X-WSSE", BuildWSSEHeader(appKey, appSecret)); //请求Body var body = new Dictionary<string, string>() { {"from", sender}, {"to", receiver}, {"templateId", templateId}, {"templateParas", templateParas}, {"statusCallback", statusCallBack}, //{"signature", signature} //使用国内短信通用模板时,必须填写签名名称 }; HttpContent content = new FormUrlEncodedContent(body); var response = client.PostAsync(apiAddress, content).Result; Console.WriteLine(response.StatusCode); //打印响应结果码 var res = response.Content.ReadAsStringAsync().Result; Console.WriteLine(res); //打印响应信息 } catch (Exception e) { Console.WriteLine(e.StackTrace); Console.WriteLine(e.Message); } } /// <summary> /// 构造X-WSSE参数值 /// </summary> /// <param name="appKey"></param> /// <param name="appSecret"></param> /// <returns></returns> static string BuildWSSEHeader(string appKey, string appSecret) { string now = DateTime.Now.ToString("yyyy-MM-ddTHH:mm:ssZ"); //Created string nonce = Guid.NewGuid().ToString().Replace("-", ""); //Nonce byte[] material = Encoding.UTF8.GetBytes(nonce + now + appSecret); byte[] hashed = SHA256Managed.Create().ComputeHash(material); string hexdigest = BitConverter.ToString(hashed).Replace("-", ""); string base64 = Convert.ToBase64String(Encoding.UTF8.GetBytes(hexdigest)); //PasswordDigest return String.Format("UsernameToken Username=\"{0}\",PasswordDigest=\"{1}\",Nonce=\"{2}\",Created=\"{3}\"", appKey, base64, nonce, now); } } } |
发送短信(示例2)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 |
using System; using System.Collections.Specialized; using System.IO; using System.Net; using System.Security.Cryptography; using System.Text; using System.Web; namespace msgsms_csharp_demo { class SendSms { static void Main(string[] args) { //必填,请参考"开发准备"获取如下数据,替换为实际值 string apiAddress = "https://smsapi.cn-north-4.myhuaweicloud.com:443/sms/batchSendSms/v1"; //APP接入地址(在控制台"应用管理"页面获取)+接口访问URI // 认证用的appKey和appSecret硬编码到代码中或者明文存储都有很大的安全风险,建议在配置文件或者环境变量中密文存放,使用时解密,确保安全; string appKey = "c8RWg3ggEcyd4D3p94bf3Y7x1Ile"; //APP_Key string appSecret = "q4Ii87Bh************80SfD7Al"; //APP_Secret string sender = "csms12345678"; //国内短信签名通道号 string templateId = "8ff55eac1d0b478ab3c06c3c6a492300"; //模板ID //条件必填,国内短信关注,当templateId指定的模板类型为通用模板时生效且必填,必须是已审核通过的,与模板类型一致的签名名称 //string signature = "华为云短信测试"; //签名名称 //必填,全局号码格式(包含国家码),示例:+86151****6789,多个号码之间用英文逗号分隔 string receiver = "+86151****6789,+86152****7890"; //短信接收人号码 //选填,短信状态报告接收地址,推荐使用域名,为空或者不填表示不接收状态报告 string statusCallBack = ""; /* * 选填,使用无变量模板时请赋空值 string templateParas = ""; * 单变量模板示例:模板内容为"您的验证码是${1}"时,templateParas可填写为"[\"369751\"]" * 双变量模板示例:模板内容为"您有${1}件快递请到${2}领取"时,templateParas可填写为"[\"3\",\"人民公园正门\"]" * 模板中的每个变量都必须赋值,且取值不能为空 * 查看更多模板规范和变量规范:产品介绍>短信模板须知和短信变量须知 */ string templateParas = "[\"369751\"]"; //模板变量,此处以单变量验证码短信为例,请客户自行生成6位验证码,并定义为字符串类型,以杜绝首位0丢失的问题(例如:002569变成了2569)。 try { //为防止因HTTPS证书认证失败造成API调用失败,需要先忽略证书信任问题 ServicePointManager.ServerCertificateValidationCallback = delegate { return true; }; //使用Tls1.2 = 3072 ServicePointManager.SecurityProtocol = SecurityProtocolType.Tls | (SecurityProtocolType)3072; HttpWebRequest myReq = (HttpWebRequest)WebRequest.Create(apiAddress); //请求方法 myReq.Method = "POST"; //请求Headers myReq.ContentType = "application/x-www-form-urlencoded"; myReq.Headers.Add("Authorization", "WSSE realm=\"SDP\",profile=\"UsernameToken\",type=\"Appkey\""); myReq.Headers.Add("X-WSSE", BuildWSSEHeader(appKey, appSecret)); //请求Body NameValueCollection keyValues = new NameValueCollection { {"from", sender}, {"to", receiver}, {"templateId", templateId}, {"templateParas", templateParas}, {"statusCallback", statusCallBack}, //{"signature", signature } //使用国内短信通用模板时,必须填写签名名称 }; string body = BuildQueryString(keyValues); //发送请求数据 StreamWriter req = new StreamWriter(myReq.GetRequestStream()); req.Write(body); req.Close(); //获取响应数据 HttpWebResponse myResp = (HttpWebResponse)myReq.GetResponse(); StreamReader resp = new StreamReader(myResp.GetResponseStream()); string result = resp.ReadToEnd(); myResp.Close(); resp.Close(); Console.WriteLine(result); } catch (Exception e) { Console.WriteLine(e.StackTrace); Console.WriteLine(e.Message); } } /// <summary> /// 构造X-WSSE参数值 /// </summary> /// <param name="appKey"></param> /// <param name="appSecret"></param> /// <returns></returns> static string BuildWSSEHeader(string appKey, string appSecret) { string now = DateTime.Now.ToString("yyyy-MM-ddTHH:mm:ssZ"); //Created string nonce = Guid.NewGuid().ToString().Replace("-", ""); //Nonce byte[] material = Encoding.UTF8.GetBytes(nonce + now + appSecret); byte[] hashed = SHA256Managed.Create().ComputeHash(material); string hexdigest = BitConverter.ToString(hashed).Replace("-", ""); string base64 = Convert.ToBase64String(Encoding.UTF8.GetBytes(hexdigest)); //PasswordDigest return String.Format("UsernameToken Username=\"{0}\",PasswordDigest=\"{1}\",Nonce=\"{2}\",Created=\"{3}\"", appKey, base64, nonce, now); } /// <summary> /// 构造请求body /// </summary> /// <param name="keyValues"></param> /// <returns></returns> static string BuildQueryString(NameValueCollection keyValues) { StringBuilder temp = new StringBuilder(); foreach (string item in keyValues.Keys) { temp.Append(item).Append("=").Append(HttpUtility.UrlEncode(keyValues.Get(item))).Append("&"); } return temp.Remove(temp.Length - 1, 1).ToString(); } } } |
发送分批短信(示例1)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 |
using Newtonsoft.Json; using System; using System.Collections; using System.Collections.Generic; using System.Net; using System.Net.Http; using System.Net.Http.Headers; using System.Security.Cryptography; using System.Text; namespace msgsms_csharp_demo { class SendDiffSms { static void Main(string[] args) { //必填,请参考"开发准备"获取如下数据,替换为实际值 string apiAddress = "https://smsapi.cn-north-4.myhuaweicloud.com:443/sms/batchSendDiffSms/v1"; //APP接入地址(在控制台"应用管理"页面获取)+接口访问URI // 认证用的appKey和appSecret硬编码到代码中或者明文存储都有很大的安全风险,建议在配置文件或者环境变量中密文存放,使用时解密,确保安全; string appKey = "c8RWg3ggEcyd4D3p94bf3Y7x1Ile"; //APP_Key string appSecret = "q4Ii87Bh************80SfD7Al"; //APP_Secret string sender = "csms12345678"; //国内短信签名通道号 string templateId_1 = "979b639cbd0b4b6b88e0fd5de4ad6f85"; //模板ID1 string templateId_2 = "979b639cbd0b4b6b88e0fd5de4ad6f85"; //模板ID2 //条件必填,国内短信关注,当templateId指定的模板类型为通用模板时生效且必填,必须是已审核通过的,与模板类型一致的签名名称 string signature_1 = "华为云短信测试"; //签名名称1 string signature_2 = "华为云短信测试"; //签名名称2 //必填,全局号码格式(包含国家码),示例:+86151****6789,多个号码之间用英文逗号分隔 string[] receiver_1 = { "+86151****6789", "+86152****7890" }; //模板1的接收号码 string[] receiver_2 = { "+86151****6789", "+86152****7890" }; //模板2的接收号码 //选填,短信状态报告接收地址,推荐使用域名,为空或者不填表示不接收状态报告 string statusCallBack = ""; /* * 选填,使用无变量模板时请赋空值 string[] templateParas = {}; * 单变量模板示例:模板内容为"您的验证码是${1}"时,templateParas可填写为{"369751"} * 双变量模板示例:模板内容为"您有${1}件快递请到${2}领取"时,templateParas可填写为{"3","人民公园正门"} * 模板中的每个变量都必须赋值,且取值不能为空 * 查看更多模板规范和变量规范:产品介绍>短信模板须知和短信变量须知 */ string[] templateParas_1 = {"123456"}; //模板1变量,此处以单变量验证码短信为例,请客户自行生成6位验证码,并定义为字符串类型,以杜绝首位0丢失的问题(例如:002569变成了2569)。 string[] templateParas_2 = {"234567"}; //模板2变量,此处以单变量验证码短信为例,请客户自行生成6位验证码,并定义为字符串类型,以杜绝首位0丢失的问题(例如:002569变成了2569)。 ArrayList smsContent = new ArrayList { //smsContent,不携带签名名称时,signature请填null InitDiffSms(receiver_1, templateId_1, templateParas_1, signature_1), InitDiffSms(receiver_2, templateId_2, templateParas_2, signature_2) }; try { //为防止因HTTPS证书认证失败造成API调用失败,需要先忽略证书信任问题 HttpClient client = new HttpClient(); ServicePointManager.ServerCertificateValidationCallback = delegate { return true; }; //请求Headers client.DefaultRequestHeaders.Add("Authorization", "WSSE realm=\"SDP\",profile=\"UsernameToken\",type=\"Appkey\""); client.DefaultRequestHeaders.Add("X-WSSE", BuildWSSEHeader(appKey, appSecret)); //请求Body var body = new Dictionary<string, object>{ {"from", sender}, {"statusCallback", statusCallBack}, {"smsContent", smsContent} }; HttpContent content = new StringContent(JsonConvert.SerializeObject(body)); //请求Headers中的Content-Type参数 content.Headers.ContentType = new MediaTypeHeaderValue("application/json"); var response = client.PostAsync(apiAddress, content).Result; Console.WriteLine(response.StatusCode); //打印响应结果码 var res = response.Content.ReadAsStringAsync().Result; Console.WriteLine(res); //打印响应信息 } catch (Exception e) { Console.WriteLine(e.StackTrace); Console.WriteLine(e.Message); } } /// <summary> /// 构造smsContent参数值 /// </summary> /// <param name="receiver"></param> /// <param name="templateId"></param> /// <param name="templateParas"></param> /// <param name="signature">签名名称,使用国内短信通用模板时填写</param> /// <returns></returns> static Dictionary<string, object> InitDiffSms(string[] receiver, string templateId, string[] templateParas, string signature) { Dictionary<string, object> dic = new Dictionary<string, object> { {"to", receiver}, {"templateId", templateId}, {"templateParas", templateParas} }; if (!signature.Equals(null) && signature.Length > 0) { dic.Add("signature", signature); } return dic; } /// <summary> /// 构造X-WSSE参数值 /// </summary> /// <param name="appKey"></param> /// <param name="appSecret"></param> /// <returns></returns> static string BuildWSSEHeader(string appKey, string appSecret) { string now = DateTime.Now.ToString("yyyy-MM-ddTHH:mm:ssZ"); //Created string nonce = Guid.NewGuid().ToString().Replace("-", ""); //Nonce byte[] material = Encoding.UTF8.GetBytes(nonce + now + appSecret); byte[] hashed = SHA256Managed.Create().ComputeHash(material); string hexdigest = BitConverter.ToString(hashed).Replace("-", ""); string base64 = Convert.ToBase64String(Encoding.UTF8.GetBytes(hexdigest)); //PasswordDigest return String.Format("UsernameToken Username=\"{0}\",PasswordDigest=\"{1}\",Nonce=\"{2}\",Created=\"{3}\"", appKey, base64, nonce, now); } } } |
发送分批短信(示例2)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 |
using Newtonsoft.Json; using System; using System.Collections; using System.Collections.Generic; using System.IO; using System.Net; using System.Security.Cryptography; using System.Text; namespace msgsms_csharp_demo { class SendDiffSms { static void Main(string[] args) { //必填,请参考"开发准备"获取如下数据,替换为实际值 string apiAddress = "https://smsapi.cn-north-4.myhuaweicloud.com:443/sms/batchSendDiffSms/v1"; //APP接入地址(在控制台"应用管理"页面获取)+接口访问URI // 认证用的appKey和appSecret硬编码到代码中或者明文存储都有很大的安全风险,建议在配置文件或者环境变量中密文存放,使用时解密,确保安全; string appKey = "c8RWg3ggEcyd4D3p94bf3Y7x1Ile"; //APP_Key string appSecret = "q4Ii87Bh************80SfD7Al"; //APP_Secret string sender = "csms12345678"; //国内短信签名通道号 string templateId_1 = "979b639cbd0b4b6b88e0fd5de4ad6f85"; //模板ID1 string templateId_2 = "979b639cbd0b4b6b88e0fd5de4ad6f85"; //模板ID2 //条件必填,国内短信关注,当templateId指定的模板类型为通用模板时生效且必填,必须是已审核通过的,与模板类型一致的签名名称 string signature_1 = "华为云短信测试"; //签名名称1 string signature_2 = "华为云短信测试"; //签名名称2 //必填,全局号码格式(包含国家码),示例:+86151****6789,多个号码之间用英文逗号分隔 string[] receiver_1 = { "+86151****6789", "+86152****7890" }; //模板1的接收号码 string[] receiver_2 = { "+86151****6789", "+86152****7890" }; //模板2的接收号码 //选填,短信状态报告接收地址,推荐使用域名,为空或者不填表示不接收状态报告 string statusCallBack = ""; /* * 选填,使用无变量模板时请赋空值 string[] templateParas = {}; * 单变量模板示例:模板内容为"您的验证码是${1}"时,templateParas可填写为{"369751"} * 双变量模板示例:模板内容为"您有${1}件快递请到${2}领取"时,templateParas可填写为{"3","人民公园正门"} * 模板中的每个变量都必须赋值,且取值不能为空 * 查看更多模板规范和变量规范:产品介绍>短信模板须知和短信变量须知 */ string[] templateParas_1 = {"123456"}; //模板1变量,此处以单变量验证码短信为例,请客户自行生成6位验证码,并定义为字符串类型,以杜绝首位0丢失的问题(例如:002569变成了2569)。 string[] templateParas_2 = {"234567"}; //模板2变量,此处以单变量验证码短信为例,请客户自行生成6位验证码,并定义为字符串类型,以杜绝首位0丢失的问题(例如:002569变成了2569)。 ArrayList smsContent = new ArrayList { //smsContent,不携带签名名称时,signature请填null InitDiffSms(receiver_1, templateId_1, templateParas_1, signature_1), InitDiffSms(receiver_2, templateId_2, templateParas_2, signature_2) }; try { //为防止因HTTPS证书认证失败造成API调用失败,需要先忽略证书信任问题 ServicePointManager.ServerCertificateValidationCallback = delegate { return true; }; //使用Tls1.2 = 3072 ServicePointManager.SecurityProtocol = SecurityProtocolType.Tls | (SecurityProtocolType)3072; HttpWebRequest myReq = (HttpWebRequest)WebRequest.Create(apiAddress); //请求方法 myReq.Method = "POST"; //请求Headers myReq.ContentType = "application/json"; myReq.Headers.Add("Authorization", "WSSE realm=\"SDP\",profile=\"UsernameToken\",type=\"Appkey\""); myReq.Headers.Add("X-WSSE", BuildWSSEHeader(appKey, appSecret)); //请求Body var body = new Dictionary<string, object>() { {"from", sender}, {"statusCallback", statusCallBack}, {"smsContent", smsContent} }; string jsonData = JsonConvert.SerializeObject(body); //发送请求数据 StreamWriter req = new StreamWriter(myReq.GetRequestStream()); req.Write(jsonData); req.Close(); //获取响应数据 HttpWebResponse myResp = (HttpWebResponse)myReq.GetResponse(); StreamReader resp = new StreamReader(myResp.GetResponseStream()); string result = resp.ReadToEnd(); myResp.Close(); resp.Close(); Console.WriteLine(result); } catch (Exception e) { Console.WriteLine(e.StackTrace); Console.WriteLine(e.Message); } } /// <summary> /// 构造smsContent参数值 /// </summary> /// <param name="receiver"></param> /// <param name="templateId"></param> /// <param name="templateParas"></param> /// <param name="signature">签名名称,使用国内短信通用模板时填写</param> /// <returns></returns> static Dictionary<string, object> InitDiffSms(string[] receiver, string templateId, string[] templateParas, string signature) { Dictionary<string, object> dic = new Dictionary<string, object> { {"to", receiver}, {"templateId", templateId}, {"templateParas", templateParas} }; if (!signature.Equals(null) && signature.Length > 0) { dic.Add("signature", signature); } return dic; } /// <summary> /// 构造X-WSSE参数值 /// </summary> /// <param name="appKey"></param> /// <param name="appSecret"></param> /// <returns></returns> static string BuildWSSEHeader(string appKey, string appSecret) { string now = DateTime.Now.ToString("yyyy-MM-ddTHH:mm:ssZ"); //Created string nonce = Guid.NewGuid().ToString().Replace("-", ""); //Nonce byte[] material = Encoding.UTF8.GetBytes(nonce + now + appSecret); byte[] hashed = SHA256Managed.Create().ComputeHash(material); string hexdigest = BitConverter.ToString(hashed).Replace("-", ""); string base64 = Convert.ToBase64String(Encoding.UTF8.GetBytes(hexdigest)); //PasswordDigest return String.Format("UsernameToken Username=\"{0}\",PasswordDigest=\"{1}\",Nonce=\"{2}\",Created=\"{3}\"", appKey, base64, nonce, now); } } } |
接收状态报告
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 |
using System; using System.Web; namespace msgsms_csharp_demo { class Report { static void Main(string[] args) { //string success_body = "sequence=1&total=1&updateTime=2018-10-31T08%3A43%3A41Z&source=2&smsMsgId=2ea20735-f856-4376-afbf-570bd70a46ee_11840135&status=DELIVRD"; string failed_body = "sequence=1&total=1&updateTime=2018-10-31T08%3A43%3A41Z&source=2&smsMsgId=2ea20735-f856-4376-afbf-570bd70a46ee_11840135&status=E200027"; //OnSmsStatusReport(success_body); OnSmsStatusReport(failed_body); } /// <summary> /// 解析状态报告数据 /// </summary> /// <param name="data">短信平台上报的状态报告数据</param> static void OnSmsStatusReport(string data) { var keyValues = HttpUtility.ParseQueryString(data); //解析状态报告数据 /** * Example: 此处已解析status为例,请按需解析所需参数并自行实现相关处理 * * 'smsMsgId': 短信唯一标识 * 'total': 长短信拆分条数 * 'sequence': 拆分后短信序号 * 'source': 状态报告来源 * 'updateTime': 资源更新时间 * 'status': 状态码 */ string status = keyValues.Get("status"); // 状态报告枚举值 // 通过status判断短信是否发送成功 if ("DELIVRD".Equals(status.ToUpper())) { Console.WriteLine("Send sms success. smsMsgId: " + keyValues.Get("smsMsgId")); } else { // 发送失败,打印status和orgCode Console.WriteLine("Send sms failed. smsMsgId: " + keyValues.Get("smsMsgId")); Console.WriteLine("Failed status: " + status); } } } } |
接收上行短信
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
using System; using System.Web; namespace msgsms_csharp_demo { class UpData { static void Main(string[] args) { string updata = "from=%2B86151****6789&to=1069****019&body=********&smsMsgId=9692b5be-c427-4525-8e73-cf4a6ac5b3f7"; onSmsUpData(updata); } /// <summary> /// 解析上行短信通知数据 /// </summary> /// <param name="data">短信平台推送的上行短信通知数据</param> static void onSmsUpData(string data) { var keyValues = HttpUtility.ParseQueryString(data); //解析上行短信通知数据 /** * Example: 此处已解析body为例,请按需解析所需参数并自行实现相关处理 * * 'smsMsgId': 上行短信唯一标识 * 'from': 上行短信发送方的号码 * 'to': 上行短信接收方的号码 * 'body': 上行短信发送的内容 */ string body = keyValues.Get("body"); // 上行短信发送的内容 Console.WriteLine("Sms up data. Body: " + body); } } } |
父主题: X-WSSE认证