Automatically Checking for and Retrying Failed Migration Tasks
Overview
After a migration task or migration task group is started by calling an OMS API, the task or task group may fail due to network fluctuations or other reasons. To resolve this problem, you can:
- Manually call the API to restart the task or task group.
- Embed the code in your service system to automatically check the status of the task or task group and to restart it whenever it fails.
- Use Huawei Cloud FunctionGraph to automatically check the status of the task or task group and to restart it whenever it fails.
The following describes how to use Huawei Cloud FunctionGraph to monitor a migration task and retry it if it fails.
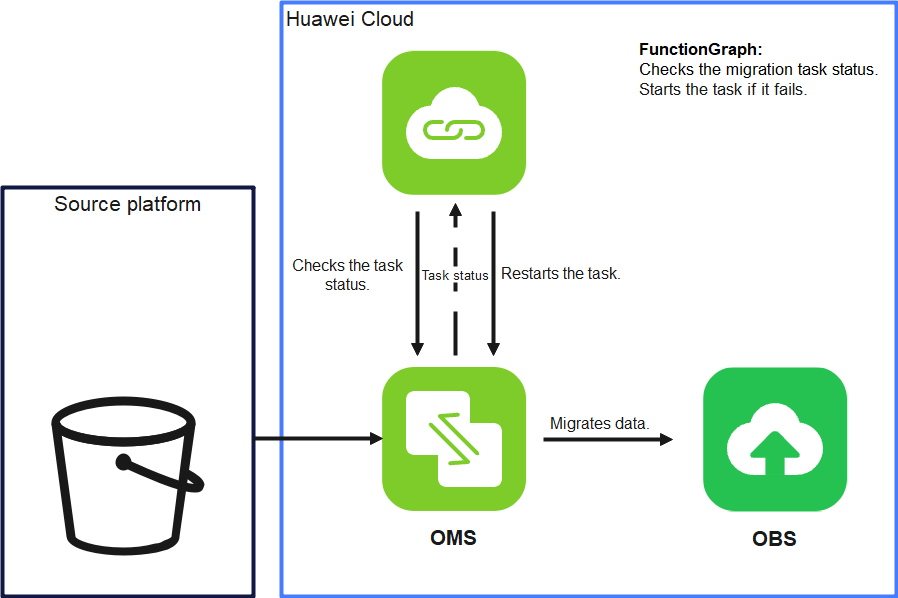
After an OMS migration task is started, FunctionGraph is triggered to periodically check the migration task status.
- If FunctionGraph detects that a migration task has failed and if the task is not included in a task group, FunctionGraph calls the OMS API to restart the migration task.
- If FunctionGraph detects that a migration task included in a task group has failed, FunctionGraph calls OMS APIs to check the status of the migration task group and keeps restarting the task until the migration task group enters a failed status.
FunctionGraph stops checking the migration task status if the migration succeeds or the number of retry times reaches a certain threshold.
Prerequisites
- You have signed up a HUAWEI ID and enabled Huawei Cloud services. If you are migrating a region within the Chinese mainland, you also must complete real-name authentication.
- You have obtained the AK/SK pairs and required permissions for the source and destination platform accounts.
- A migration task or migration task group has been started.
Step 1: Creating a Package
This example uses Java 8 to periodically check for and restart failed migration tasks. For details about function development, see Developing Functions in Java.
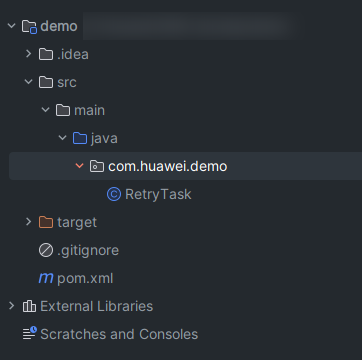
<dependency> <groupId>Runtime</groupId> <artifactId>Runtime</artifactId> <version>2.0.5</version> </dependency> <dependency> <groupId>com.huaweicloud.sdk</groupId> <artifactId>huaweicloud-sdk-oms</artifactId> <version>3.1.86</version> </dependency>
package com.huawei.demo; import com.huawei.services.runtime.Context; import com.huawei.services.runtime.RuntimeLogger; import com.huawei.services.runtime.entity.timer.TimerTriggerEvent; import com.huaweicloud.sdk.core.auth.BasicCredentials; import com.huaweicloud.sdk.core.auth.ICredential; import com.huaweicloud.sdk.core.exception.ConnectionException; import com.huaweicloud.sdk.core.exception.RequestTimeoutException; import com.huaweicloud.sdk.core.exception.ServiceResponseException; import com.huaweicloud.sdk.oms.v2.OmsClient; import com.huaweicloud.sdk.oms.v2.model.RetryTaskGroupReq; import com.huaweicloud.sdk.oms.v2.model.RetryTaskGroupRequest; import com.huaweicloud.sdk.oms.v2.model.ShowTaskGroupRequest; import com.huaweicloud.sdk.oms.v2.model.ShowTaskGroupResponse; import com.huaweicloud.sdk.oms.v2.model.ShowTaskRequest; import com.huaweicloud.sdk.oms.v2.model.ShowTaskResponse; import com.huaweicloud.sdk.oms.v2.model.StartTaskReq; import com.huaweicloud.sdk.oms.v2.model.StartTaskRequest; import com.huaweicloud.sdk.oms.v2.region.OmsRegion; public class RetryTask { private RuntimeLogger logger; private String dstAk; private String dstSk; private String srcAk; private String srcSk; public String checkAndRetryTask(TimerTriggerEvent event, Context context) { logger = context.getLogger(); String region = context.getUserData("region_id"); String taskId = context.getUserData("task_id"); int maxRetryCount = Integer.parseInt(context.getUserData("retry_count")); srcAk = context.getUserData("src_ak"); srcSk = context.getUserData("src_sk"); dstAk = context.getUserData("dst_ak"); dstSk = context.getUserData("dst_ak"); String ak = context.getAccessKey(); String sk = context.getSecretKey(); ICredential auth = new BasicCredentials() .withAk(ak) .withSk(sk); OmsClient client = OmsClient.newBuilder() .withCredential(auth) .withRegion(OmsRegion.valueOf(region)) .build(); ShowTaskRequest request = new ShowTaskRequest(); request.withTaskId(taskId); int retryCount = 0; try { while (true) { ShowTaskResponse taskResponse = client.showTask(request); Integer status = taskResponse.getStatus(); logger.info("task status: "+status); if (retryCount >= maxRetryCount || status == 5) { break; } if ("NORMAL_TASK".equals(taskResponse.getGroupType().getValue()) && status == 4) { retryTask(client, taskId); logger.info("task restart:" + taskId); retryCount++; continue; } if ("GROUP_TASK".equals(taskResponse.getGroupType().getValue()) && status == 4) { retryTasksGroup(client, taskResponse.getGroupId()); logger.info("task group restart:" + taskResponse.getGroupId()); retryCount++; } } return "ok"; } catch (ConnectionException e) { logger.error("Failed to restart the task, error_msg: " + e.getMessage()); } catch (RequestTimeoutException e) { logger.error("Time out, failed to restart the task, error_msg: " + e.getMessage()); } catch (ServiceResponseException e) { logger.error("Failed to restart the task, error_code: " + e.getErrorCode() + ", error_msg: " + e.getErrorMsg()); } return "fail"; } private void retryTasksGroup(OmsClient client, String groupId) { ShowTaskGroupRequest taskGroupRequest = new ShowTaskGroupRequest(); taskGroupRequest.withGroupId(groupId); ShowTaskGroupResponse taskGroupResponse = client.showTaskGroup(taskGroupRequest); if (taskGroupResponse.getStatus() == 5) { RetryTaskGroupRequest retryTaskGroupRequest = new RetryTaskGroupRequest(); retryTaskGroupRequest.withGroupId(groupId); RetryTaskGroupReq body = new RetryTaskGroupReq(); body.withMigrateFailedObject(false); body.withDstAk(dstAk); body.withDstSk(dstSk); body.withSrcAk(srcAk); body.withSrcSk(srcSk); retryTaskGroupRequest.withBody(body); client.retryTaskGroup(retryTaskGroupRequest); } } private void retryTask(OmsClient client, String taskId) { StartTaskRequest startTaskRequest = new StartTaskRequest(); StartTaskReq body = new StartTaskReq(); body.withMigrateFailedObject(false); body.withDstAk(dstAk); body.withDstSk(dstSk); body.withSrcAk(srcAk); body.withSrcSk(srcSk); startTaskRequest.withBody(body); startTaskRequest.withTaskId(taskId); client.startTask(startTaskRequest); } }
Step 2: Create a Function
- Log in to the FunctionGraph console, and choose Functions > Function List in the navigation pane.
- Click Create Function.
- Set the function information. After setting the basic information, click Create.
- Function Name: Enter check_retry_task.
- Agency: Select default.
- Runtime: Select Java 8.
- Click the name of the check_retry_task function. On the Code tab page, choose Upload > Local JAR, upload the JAR package compiled using the sample code, and click Deploy.
- Choose Configuration > Basic Settings, set the following parameters, and click Save.
- Memory: Select 512.
- Execution Timeout: Enter 10.
- Handler: Enter com.huawei.demo.RetryTask.checkAndRetryTask.
The handler must be named in the format of [Package name].[Class name].[Execution function name]. Modify the handler name based on the uploaded code.
- App: Retain the default value default.
- Description: Enter a description.
Step 3: Configuring Environment Variables
- On the check_retry_task details page, choose Configuration > Environment Variables.
- Click Add, configure environment variables based on Table 1, and click Save.
Table 1 Environment variables Environment Variable
Description
region_id
Specifies the region where the destination bucket is located, for example, cn-north-4.
task_id
Specifies the migration task ID.
max_retry_count
Specifies the number of retry attempts.
dst_ak
Specifies the AK of the destination account. Enable Encrypted for it.
dst_sk
Specifies the SK of the destination account. Enable Encrypted for it.
src_ak
Specifies the AK of the source account. Enable Encrypted for it.
src_sk
Specifies the SK of the source account. Enable Encrypted for it.
Step 4: Configuring an Event Source
After creating a function, you can add a timer event source for the function, so that the function can be triggered to periodically check the migration status of the migration task and restart the task if it fails.
- On the check_retry_task details page, choose Configuration > Triggers.
- Click Create Trigger.
- Configure the following parameters:
- Trigger Type: Select Timer.
- Timer Name: Enter a timer name, for example, Timer.
- Rule: Set a fixed rate or a cron expression.
- Fixed rate: The function is triggered at a fixed rate of minutes, hours, or days. You can set a fixed rate from 1 to 60 minutes, 1 to 24 hours, or 1 to 30 days.
- Cron Expression: The function is triggered based on a complex rule. For example, you can set a function to be executed at 08:30:00 from Monday to Friday. For more information, see Cron Expressions for a Function Timer Trigger.
- Enable Trigger: Choose whether to enable the timer trigger.
- Additional Information: The additional information you configure will be put into the user_event field of the timer event source. For details, see Supported Event Sources.
- Click OK.
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot