Preparing a PHP Development Environment
Preparations
- Sign up for a HUAWEI ID and enable Huawei Cloud services. Complete real-name authentication and ensure that your account is not in arrears or frozen.
- Obtain PHP 5.6 or later. You can run the php --version command to check the version information.
- Obtain an AK and an SK on the My Credentials > Access Keys page.
Figure 1 Obtaining an AK and an SK
- Obtain the IAM user name, account name, and the project ID of your target region on the My Credentials > API Credentials page. The information will be used during service calling. Save it in advance.
Figure 2 My Credentials
Installing the SDK
You are advised to use Composer to install the SDK.
Composer is a dependency manager for PHP. It allows you to declare and install dependencies in a project.
// Install Composer. curl -sS https://getcomposer.org/installer | php // Install the PHP SDK. composer require huaweicloud/huaweicloud-sdk-php
After the installation is complete, you need to import the autoload file of Composer.
require 'path/to/vendor/autoload.php';
Using the SDK
- Import dependency modules.
<?php namespace HuaweiCloud\SDK\Image\V2\Model; require_once "vendor/autoload.php"; use HuaweiCloud\SDK\Core\Auth\BasicCredentials; use HuaweiCloud\SDK\Core\Http\HttpConfig; use HuaweiCloud\SDK\Core\Exceptions\ConnectionException; use HuaweiCloud\SDK\Core\Exceptions\RequestTimeoutException; use HuaweiCloud\SDK\Core\Exceptions\ServiceResponseException; use HuaweiCloud\SDK\Image\V2\ImageClient;
- Configure client connection parameters.
- Using the default configuration
// Use the default configuration. $config = HttpConfig::getDefaultConfig();
- (Optional) Configuring a network proxy
// Use a proxy server. $config->setProxyProtocol('http'); $config->setProxyHost('proxy.huawei.com'); $config->setProxyPort(8080); $config->setProxyUser('username'); $config->setProxyPassword('password');
- (Optional) Configuring the timeout
// The default connection timeout interval is 60 seconds, and the default read timeout interval is 120 seconds. You can change the default values as needed. $config->setTimeout(120); $config->setConnectionTimeout(60);
- (Optional) Configuring an SSL
// Skip server certificate verification. $config->setIgnoreSslVerification(true); // Configure the server CA certificate so that the SDK can verify the server certificate. $config->setCertFile("{yourCertFile}");
- Using the default configuration
- Configure authentication information.
Configure ak, sk, and projectId. AK is used together with SK to sign requests cryptographically, ensuring that the requests are secret, complete, and correct.
$ak = getenv('HUAWEICLOUD_SDK_AK'); $sk = getenv('HUAWEICLOUD_SDK_SK') $projectId = getenv("PROJECT_ID");; $credentials = new BasicCredentials($ak,$sk,$projectId);
The authentication parameters are as follows:
- ak and sk: access key and secrete access key, respectively. For how to obtain them, see Preparations.
- projectId: Huawei Cloud project ID. For details about how to obtain the ID, see Preparations.
- endpoint indicates the regions and endpoints for Huawei Cloud services. For details, see Regions and Endpoints.
- Hard-coded or plaintext AK and SK are risky. For security purposes, encrypt your AK and SK and store them in the configuration file or environment variables.
- In this example, the AK and SK are stored in environment variables for identity authentication. Before running this example, configure environment variables HUAWEICLOUD_SDK_AK, HUAWEICLOUD_SDK_SK, and PROJECT_ID.
- Initialize the client.
Specify the cloud service endpoint and initialize the client.
$endpoint = "https://image.xx-xxx-xx.myhuaweicloud.com"; $client = ImageClient::newBuilder(new ImageClient) ->withHttpConfig($config) ->withEndpoint($endpoint) ->withCredentials($credentials) ->build();
- Send a request and check the response.
$request = new RunImageMediaTaggingRequest(); $body = new ImageMediaTaggingReq(); $body->setLimit(10); $body->setThreshold(60); $body->setLanguage("en"); $body->setImage ("Image Base64"); $request->setBody($body); try { $response = $client->RunImageMediaTagging($request); echo "\n"; echo $response; } catch (ConnectionException $e) { $msg = $e->getMessage(); echo "\n". $msg ."\n"; } catch (RequestTimeoutException $e) { $msg = $e->getMessage(); echo "\n". $msg ."\n"; } catch (ServiceResponseException $e) { echo "\n"; echo $e->getHttpStatusCode(). "\n"; echo $e->getRequestId(). "\n"; echo $e->getErrorCode() . "\n"; echo $e->getErrorMsg() . "\n"; }
- Handle exceptions.
Table 1 Exceptions Level-1 Category
Level-1 Category Description
Level-2 Category
Level-2 Category Description
ConnectionException
Connection exception
HostUnreachableException
The network is unreachable or access is rejected.
SslHandShakeException
SSL authentication is abnormal.
RequestTimeoutException
Response timeout exception
CallTimeoutException
The server fails to respond to a single request before timeout.
RetryOutageException
No valid response is returned after the maximum number of retries specified in the retry policy is reached.
ServiceResponseException
Server response exception
ServerResponseException
Internal server error. HTTP response code: [500,].
ClientRequestException
Invalid request parameter. HTTP response code: [400, 500).
// Capture and process different types of exceptions. try { $response = $client->RunImageMediaTagging($request); echo "\n"; echo $response; } catch (ConnectionException $e) { $msg = $e->getMessage(); echo "\n". $msg ."\n"; } catch (RequestTimeoutException $e) { $msg = $e->getMessage(); echo "\n". $msg ."\n"; } catch (ServiceResponseException $e) { echo "\n"; echo $e->getHttpStatusCode(). "\n"; echo $e->getRequestId(). "\n"; echo $e->getErrorCode() . "\n"; echo $e->getErrorMsg() . "\n"; }
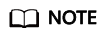
For details about how to use an asynchronous client and configure logs, see SDK Center and PHP SDK Usage Guide.
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot