Scaling a Node Pool
Function
This API is used to scale in or out a node pool.
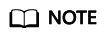
The URL for cluster management is in the format of https://Endpoint/uri, where uri specifies the resource path for API access.
Calling Method
For details, see Calling APIs.
URI
POST /api/v3/projects/{project_id}/clusters/{cluster_id}/nodepools/{nodepool_id}/operation/scale
Parameter |
Mandatory |
Type |
Description |
---|---|---|---|
project_id |
Yes |
String |
Details: Project ID. For details about how to obtain the value, see How to Obtain Parameters in the API URI. Constraints: None Options: Project IDs of the account Default value: N/A |
cluster_id |
Yes |
String |
Details: Cluster ID. For details about how to obtain the value, see How to Obtain Parameters in the API URI. Constraints: None Options: Cluster IDs Default value: N/A |
nodepool_id |
Yes |
String |
Details: Node pool ID. For details about how to obtain the ID, see How to Obtain Parameters in the API URI. Constraints: None Options: Cluster IDs Default value: N/A |
Request Parameters
Parameter |
Mandatory |
Type |
Description |
---|---|---|---|
Content-Type |
Yes |
String |
Details: Request body type or format Constraints: The GET method is not verified. Options:
Default value: N/A |
X-Auth-Token |
Yes |
String |
Details: Requests for calling an API can be authenticated using either a token or AK/SK. If token-based authentication is used, this parameter is mandatory and must be set to a user token. For details, see Obtaining a User Token. Constraints: None Options: N/A Default value: N/A |
Parameter |
Mandatory |
Type |
Description |
---|---|---|---|
kind |
Yes |
String |
API type. The value is fixed at NodePool. |
apiVersion |
Yes |
String |
API version. The value is fixed at v3. |
spec |
Yes |
ScaleNodePoolSpec object |
Parameters in the request for scaling a node pool |
Parameter |
Mandatory |
Type |
Description |
---|---|---|---|
desiredNodeCount |
Yes |
Integer |
Desired number of nodes in a node pool |
scaleGroups |
Yes |
Array of strings |
Node pool to be scaled in or out. Only one scaling group can be specified. To scale the default scaling group, set the value to default. |
options |
No |
ScaleNodePoolOptions object |
Configurations of node pool scaling options |
Parameter |
Mandatory |
Type |
Description |
---|---|---|---|
scalableChecking |
No |
String |
Scale-out status check policy. The value can be instant (synchronous checks) or async (asynchronous checks). It defaults to instant. |
billingConfigOverride |
No |
ScaleUpBillingConfigOverride object |
Overwrites the default billing mode configuration of a node during node pool scaling. |
Parameter |
Mandatory |
Type |
Description |
---|---|---|---|
billingMode |
Yes |
Integer |
Billing mode of a node. The value can be 0 (pay-per-use) or 1 (yearly/monthly). |
extendParam |
No |
ScaleUpExtendParam object |
Billing mode of a node during node pool scaling |
Parameter |
Mandatory |
Type |
Description |
---|---|---|---|
periodNum |
Yes |
Integer |
Yearly/Monthly duration. For monthly billing, the value falls between 1 and 9, while for yearly billing, it falls between 1 and 3. |
periodType |
Yes |
String |
Yearly/Monthly billing mode. The value can be year or month. |
isAutoRenew |
No |
Boolean |
(Optional) Whether auto renewal is enabled. If this parameter is left blank, the isAutoRenew settings take effect. |
isAutoPay |
No |
Boolean |
(Optional) Whether auto payment is enabled. If this parameter is left blank, the isAutoPay settings take effect. |
Response Parameters
None
Example Requests
-
Scale out nodes in the default scaling group of a node pool (pay-per-use).
{ "kind" : "NodePool", "apiVersion" : "v3", "spec" : { "desiredNodeCount" : 1, "scaleGroups" : [ "default" ] } }
-
Scale out nodes in the default scaling group of a node pool (yearly/monthly).
{ "kind" : "NodePool", "apiVersion" : "v3", "spec" : { "desiredNodeCount" : 1, "scaleGroups" : [ "default" ], "options" : { "billingConfigOverride" : { "billingMode" : 1, "extendParam" : { "periodNum" : 1, "periodType" : "month", "isAutoRenew" : false, "isAutoPay" : false } } } } }
Example Responses
None
SDK Sample Code
The SDK sample code is as follows.
-
Scale out nodes in the default scaling group of a node pool (pay-per-use).
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61
package com.huaweicloud.sdk.test; import com.huaweicloud.sdk.core.auth.ICredential; import com.huaweicloud.sdk.core.auth.BasicCredentials; import com.huaweicloud.sdk.core.exception.ConnectionException; import com.huaweicloud.sdk.core.exception.RequestTimeoutException; import com.huaweicloud.sdk.core.exception.ServiceResponseException; import com.huaweicloud.sdk.cce.v3.region.CceRegion; import com.huaweicloud.sdk.cce.v3.*; import com.huaweicloud.sdk.cce.v3.model.*; import java.util.List; import java.util.ArrayList; public class ScaleNodePoolSolution { public static void main(String[] args) { // The AK and SK used for authentication are hard-coded or stored in plaintext, which has great security risks. It is recommended that the AK and SK be stored in ciphertext in configuration files or environment variables and decrypted during use to ensure security. // In this example, AK and SK are stored in environment variables for authentication. Before running this example, set environment variables CLOUD_SDK_AK and CLOUD_SDK_SK in the local environment String ak = System.getenv("CLOUD_SDK_AK"); String sk = System.getenv("CLOUD_SDK_SK"); String projectId = "{project_id}"; ICredential auth = new BasicCredentials() .withProjectId(projectId) .withAk(ak) .withSk(sk); CceClient client = CceClient.newBuilder() .withCredential(auth) .withRegion(CceRegion.valueOf("<YOUR REGION>")) .build(); ScaleNodePoolRequest request = new ScaleNodePoolRequest(); request.withClusterId("{cluster_id}"); request.withNodepoolId("{nodepool_id}"); ScaleNodePoolRequestBody body = new ScaleNodePoolRequestBody(); List<String> listSpecScaleGroups = new ArrayList<>(); listSpecScaleGroups.add("default"); ScaleNodePoolSpec specbody = new ScaleNodePoolSpec(); specbody.withDesiredNodeCount(1) .withScaleGroups(listSpecScaleGroups); body.withSpec(specbody); body.withApiVersion("v3"); body.withKind("NodePool"); request.withBody(body); try { ScaleNodePoolResponse response = client.scaleNodePool(request); System.out.println(response.toString()); } catch (ConnectionException e) { e.printStackTrace(); } catch (RequestTimeoutException e) { e.printStackTrace(); } catch (ServiceResponseException e) { e.printStackTrace(); System.out.println(e.getHttpStatusCode()); System.out.println(e.getRequestId()); System.out.println(e.getErrorCode()); System.out.println(e.getErrorMsg()); } } }
-
Scale out nodes in the default scaling group of a node pool (yearly/monthly).
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72
package com.huaweicloud.sdk.test; import com.huaweicloud.sdk.core.auth.ICredential; import com.huaweicloud.sdk.core.auth.BasicCredentials; import com.huaweicloud.sdk.core.exception.ConnectionException; import com.huaweicloud.sdk.core.exception.RequestTimeoutException; import com.huaweicloud.sdk.core.exception.ServiceResponseException; import com.huaweicloud.sdk.cce.v3.region.CceRegion; import com.huaweicloud.sdk.cce.v3.*; import com.huaweicloud.sdk.cce.v3.model.*; import java.util.List; import java.util.ArrayList; public class ScaleNodePoolSolution { public static void main(String[] args) { // The AK and SK used for authentication are hard-coded or stored in plaintext, which has great security risks. It is recommended that the AK and SK be stored in ciphertext in configuration files or environment variables and decrypted during use to ensure security. // In this example, AK and SK are stored in environment variables for authentication. Before running this example, set environment variables CLOUD_SDK_AK and CLOUD_SDK_SK in the local environment String ak = System.getenv("CLOUD_SDK_AK"); String sk = System.getenv("CLOUD_SDK_SK"); String projectId = "{project_id}"; ICredential auth = new BasicCredentials() .withProjectId(projectId) .withAk(ak) .withSk(sk); CceClient client = CceClient.newBuilder() .withCredential(auth) .withRegion(CceRegion.valueOf("<YOUR REGION>")) .build(); ScaleNodePoolRequest request = new ScaleNodePoolRequest(); request.withClusterId("{cluster_id}"); request.withNodepoolId("{nodepool_id}"); ScaleNodePoolRequestBody body = new ScaleNodePoolRequestBody(); ScaleUpExtendParam extendParamBillingConfigOverride = new ScaleUpExtendParam(); extendParamBillingConfigOverride.withPeriodNum(1) .withPeriodType("month") .withIsAutoRenew(false) .withIsAutoPay(false); ScaleUpBillingConfigOverride billingConfigOverrideOptions = new ScaleUpBillingConfigOverride(); billingConfigOverrideOptions.withBillingMode(1) .withExtendParam(extendParamBillingConfigOverride); ScaleNodePoolOptions optionsSpec = new ScaleNodePoolOptions(); optionsSpec.withBillingConfigOverride(billingConfigOverrideOptions); List<String> listSpecScaleGroups = new ArrayList<>(); listSpecScaleGroups.add("default"); ScaleNodePoolSpec specbody = new ScaleNodePoolSpec(); specbody.withDesiredNodeCount(1) .withScaleGroups(listSpecScaleGroups) .withOptions(optionsSpec); body.withSpec(specbody); body.withApiVersion("v3"); body.withKind("NodePool"); request.withBody(body); try { ScaleNodePoolResponse response = client.scaleNodePool(request); System.out.println(response.toString()); } catch (ConnectionException e) { e.printStackTrace(); } catch (RequestTimeoutException e) { e.printStackTrace(); } catch (ServiceResponseException e) { e.printStackTrace(); System.out.println(e.getHttpStatusCode()); System.out.println(e.getRequestId()); System.out.println(e.getErrorCode()); System.out.println(e.getErrorMsg()); } } }
-
Scale out nodes in the default scaling group of a node pool (pay-per-use).
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45
# coding: utf-8 import os from huaweicloudsdkcore.auth.credentials import BasicCredentials from huaweicloudsdkcce.v3.region.cce_region import CceRegion from huaweicloudsdkcore.exceptions import exceptions from huaweicloudsdkcce.v3 import * if __name__ == "__main__": # The AK and SK used for authentication are hard-coded or stored in plaintext, which has great security risks. It is recommended that the AK and SK be stored in ciphertext in configuration files or environment variables and decrypted during use to ensure security. # In this example, AK and SK are stored in environment variables for authentication. Before running this example, set environment variables CLOUD_SDK_AK and CLOUD_SDK_SK in the local environment ak = os.environ["CLOUD_SDK_AK"] sk = os.environ["CLOUD_SDK_SK"] projectId = "{project_id}" credentials = BasicCredentials(ak, sk, projectId) client = CceClient.new_builder() \ .with_credentials(credentials) \ .with_region(CceRegion.value_of("<YOUR REGION>")) \ .build() try: request = ScaleNodePoolRequest() request.cluster_id = "{cluster_id}" request.nodepool_id = "{nodepool_id}" listScaleGroupsSpec = [ "default" ] specbody = ScaleNodePoolSpec( desired_node_count=1, scale_groups=listScaleGroupsSpec ) request.body = ScaleNodePoolRequestBody( spec=specbody, api_version="v3", kind="NodePool" ) response = client.scale_node_pool(request) print(response) except exceptions.ClientRequestException as e: print(e.status_code) print(e.request_id) print(e.error_code) print(e.error_msg)
-
Scale out nodes in the default scaling group of a node pool (yearly/monthly).
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59
# coding: utf-8 import os from huaweicloudsdkcore.auth.credentials import BasicCredentials from huaweicloudsdkcce.v3.region.cce_region import CceRegion from huaweicloudsdkcore.exceptions import exceptions from huaweicloudsdkcce.v3 import * if __name__ == "__main__": # The AK and SK used for authentication are hard-coded or stored in plaintext, which has great security risks. It is recommended that the AK and SK be stored in ciphertext in configuration files or environment variables and decrypted during use to ensure security. # In this example, AK and SK are stored in environment variables for authentication. Before running this example, set environment variables CLOUD_SDK_AK and CLOUD_SDK_SK in the local environment ak = os.environ["CLOUD_SDK_AK"] sk = os.environ["CLOUD_SDK_SK"] projectId = "{project_id}" credentials = BasicCredentials(ak, sk, projectId) client = CceClient.new_builder() \ .with_credentials(credentials) \ .with_region(CceRegion.value_of("<YOUR REGION>")) \ .build() try: request = ScaleNodePoolRequest() request.cluster_id = "{cluster_id}" request.nodepool_id = "{nodepool_id}" extendParamBillingConfigOverride = ScaleUpExtendParam( period_num=1, period_type="month", is_auto_renew=False, is_auto_pay=False ) billingConfigOverrideOptions = ScaleUpBillingConfigOverride( billing_mode=1, extend_param=extendParamBillingConfigOverride ) optionsSpec = ScaleNodePoolOptions( billing_config_override=billingConfigOverrideOptions ) listScaleGroupsSpec = [ "default" ] specbody = ScaleNodePoolSpec( desired_node_count=1, scale_groups=listScaleGroupsSpec, options=optionsSpec ) request.body = ScaleNodePoolRequestBody( spec=specbody, api_version="v3", kind="NodePool" ) response = client.scale_node_pool(request) print(response) except exceptions.ClientRequestException as e: print(e.status_code) print(e.request_id) print(e.error_code) print(e.error_msg)
-
Scale out nodes in the default scaling group of a node pool (pay-per-use).
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51
package main import ( "fmt" "github.com/huaweicloud/huaweicloud-sdk-go-v3/core/auth/basic" cce "github.com/huaweicloud/huaweicloud-sdk-go-v3/services/cce/v3" "github.com/huaweicloud/huaweicloud-sdk-go-v3/services/cce/v3/model" region "github.com/huaweicloud/huaweicloud-sdk-go-v3/services/cce/v3/region" ) func main() { // The AK and SK used for authentication are hard-coded or stored in plaintext, which has great security risks. It is recommended that the AK and SK be stored in ciphertext in configuration files or environment variables and decrypted during use to ensure security. // In this example, AK and SK are stored in environment variables for authentication. Before running this example, set environment variables CLOUD_SDK_AK and CLOUD_SDK_SK in the local environment ak := os.Getenv("CLOUD_SDK_AK") sk := os.Getenv("CLOUD_SDK_SK") projectId := "{project_id}" auth := basic.NewCredentialsBuilder(). WithAk(ak). WithSk(sk). WithProjectId(projectId). Build() client := cce.NewCceClient( cce.CceClientBuilder(). WithRegion(region.ValueOf("<YOUR REGION>")). WithCredential(auth). Build()) request := &model.ScaleNodePoolRequest{} request.ClusterId = "{cluster_id}" request.NodepoolId = "{nodepool_id}" var listScaleGroupsSpec = []string{ "default", } specbody := &model.ScaleNodePoolSpec{ DesiredNodeCount: int32(1), ScaleGroups: listScaleGroupsSpec, } request.Body = &model.ScaleNodePoolRequestBody{ Spec: specbody, ApiVersion: "v3", Kind: "NodePool", } response, err := client.ScaleNodePool(request) if err == nil { fmt.Printf("%+v\n", response) } else { fmt.Println(err) } }
-
Scale out nodes in the default scaling group of a node pool (yearly/monthly).
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67
package main import ( "fmt" "github.com/huaweicloud/huaweicloud-sdk-go-v3/core/auth/basic" cce "github.com/huaweicloud/huaweicloud-sdk-go-v3/services/cce/v3" "github.com/huaweicloud/huaweicloud-sdk-go-v3/services/cce/v3/model" region "github.com/huaweicloud/huaweicloud-sdk-go-v3/services/cce/v3/region" ) func main() { // The AK and SK used for authentication are hard-coded or stored in plaintext, which has great security risks. It is recommended that the AK and SK be stored in ciphertext in configuration files or environment variables and decrypted during use to ensure security. // In this example, AK and SK are stored in environment variables for authentication. Before running this example, set environment variables CLOUD_SDK_AK and CLOUD_SDK_SK in the local environment ak := os.Getenv("CLOUD_SDK_AK") sk := os.Getenv("CLOUD_SDK_SK") projectId := "{project_id}" auth := basic.NewCredentialsBuilder(). WithAk(ak). WithSk(sk). WithProjectId(projectId). Build() client := cce.NewCceClient( cce.CceClientBuilder(). WithRegion(region.ValueOf("<YOUR REGION>")). WithCredential(auth). Build()) request := &model.ScaleNodePoolRequest{} request.ClusterId = "{cluster_id}" request.NodepoolId = "{nodepool_id}" isAutoRenewExtendParam:= false isAutoPayExtendParam:= false extendParamBillingConfigOverride := &model.ScaleUpExtendParam{ PeriodNum: int32(1), PeriodType: "month", IsAutoRenew: &isAutoRenewExtendParam, IsAutoPay: &isAutoPayExtendParam, } billingConfigOverrideOptions := &model.ScaleUpBillingConfigOverride{ BillingMode: int32(1), ExtendParam: extendParamBillingConfigOverride, } optionsSpec := &model.ScaleNodePoolOptions{ BillingConfigOverride: billingConfigOverrideOptions, } var listScaleGroupsSpec = []string{ "default", } specbody := &model.ScaleNodePoolSpec{ DesiredNodeCount: int32(1), ScaleGroups: listScaleGroupsSpec, Options: optionsSpec, } request.Body = &model.ScaleNodePoolRequestBody{ Spec: specbody, ApiVersion: "v3", Kind: "NodePool", } response, err := client.ScaleNodePool(request) if err == nil { fmt.Printf("%+v\n", response) } else { fmt.Println(err) } }
For SDK sample code of more programming languages, see the Sample Code tab in API Explorer. SDK sample code can be automatically generated.
Status Codes
Status Code |
Description |
---|---|
202 |
The node pool scaling is accepted. Nodes in the node pool will be added or deleted based on the expected number of nodes in the node pool after scaling. |
Error Codes
See Error Codes.
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot