Vector Functions and Operators
floatvector supports data conversion between the vector type and array type. In addition, strings in specific formats can be converted to the vector type.
array<->floatvector: During data type conversion, the vector data type can be freely converted to the corresponding array type. The member data type of the floatvector vector is floating point.
string ->floatvector: You need to pay attention to the character string format. You need to contain arrays using square brackets ([]) or braces ({}) and separate elements using commas (,).
floatvector
Function: Converts array data into vector data.
Scenario 1:
Input parameter type: anyarray
Output parameter type: floatvector
Code example:
gaussdb=# SELECT floatvector(ARRAY[1,2,9.3]);
Scenario 2:
Type of input parameter 1: floatvector
Type of input parameter 2: integer
Output parameter type: floatvector
Code example:
gaussdb=# SELECT floatvector(floatvector('[1,2,9.3]'),3);
vector_to_array
Function: Converts vector data into array data.
Input parameter type: floatvector
Output parameter type: real[]
Code example:
gaussdb=# SELECT vector_to_array(floatvector('[1,2,3]'));
text_to_vector
Function: Converts character data into vector data.
Input parameter type: cstring
Output parameter type: floatvector
Code example:
gaussdb=# SELECT text_to_vector('[1,2,9.3]');
vector_in
Function: Specifies the floatvector input conversion function (text format).
Type of input parameter 1: cstring
Type of input parameter 2: OID
Type of input parameter 3: int4
Output parameter type: floatvector
Code example:
gaussdb=# SELECT vector_in('[1,2,3]',701,3);
vector_out
Function: Specifies the floatvector output conversion function (text format).
Input parameter type: floatvector
Output parameter type: cstring
Code example:
gaussdb=# SELECT vector_out(floatvector('[1,2,3]'));
vector_send
Function: Specifies the floatvector input conversion function (binary format).
Input parameter type: floatvector
Output parameter type: bytea
Code example:
gaussdb=# SELECT vector_send(floatvector('[1,2,3]'));
vector_recv
Function: Specifies the floatvector output conversion function (binary format).
Type of input parameter 1: integer
Type of input parameter 2: OID
Type of input parameter 3: int4
Output parameter type: floatvector
Code example:
-- This is a system function and cannot be called by SQL statements.
vector_typmod_in
Function: Specifies the floatvector input type modifier function.
Input parameter type: cstring[]
Output parameter type: integer
Code example:
gaussdb=# SELECT vector_typmod_in( '{1}' );
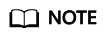
- Vector data type members support only single precision.
- Only the same dimension is supported for vector calculation. If the dimensions are different, an error is reported.
- floatvector supports vector addition and subtraction. The dot product operation is performed by the inner_product function.
- When creating a table, you must specify dimensions for each vector type. If the dimensions of the inserted data are different from the specified dimensions, the database reports an error.
- If data has been inserted into the data table, the data in the table must comply with the dimension settings when the vector dimensions are changed. Otherwise, the database reports an error. If a vector index has been created for a vector attribute, the data type of the attribute cannot be switched to another data type, and the database reports an error.
boolvector
Scenario 1:
Function: Converts array data into vector data.
Input parameter type: anyarray
Output parameter type: boolvector
Code example:
gaussdb=# SELECT boolvector(ARRAY[1,0,1]);
Scenario 2:
Function: Converts to boolvector and detects dimensions.
Type of input parameter 1: boolvector
Type of input parameter 2: integer
Output parameter type: boolvector
Code example:
gaussdb=# SELECT boolvector(boolvector('[1,0,1]'),3);
boolvector_to_array
Function: Converts vector data into array data.
Input parameter type: boolvector
Output parameter type: anyarray
Code example:
gaussdb=# SELECT boolvector_to_array(boolvector('[1,0,1]'));
text_to_boolvector
Function: Converts character data into vector data.
Input parameter type: cstring
Output parameter type: boolvector
Code example:
gaussdb=# SELECT text_to_boolvector('[1,1,1]');
bool_vector_in
Function: Specifies the boolvector input conversion function (text format).
Type of input parameter 1: cstring
Type of input parameter 2: OID
Type of input parameter 3: int4
Output parameter type: boolvector
Code example:
gaussdb=# SELECT bool_vector_in('[1,1,1]',701,3);
bool_vector_out
Function: Specifies the boolvector output conversion function (text format).
Input parameter type: boolvector
Output parameter type: cstring
Code example:
gaussdb=# SELECT bool_vector_out(boolvector('[1,1,1]'));
bool_vector_send
Function: Specifies the boolvector input conversion function (binary format).
Input parameter type: boolvector
Output parameter type: bytea
Code example:
gaussdb=# SELECT bool_vector_send(boolvector('[1,1,1]'));
bool_vector_recv
Function: Specifies the boolvector output conversion function (binary format).
Type of input parameter 1: internal
Type of input parameter 2: OID
Type of input parameter 3: int4
Output parameter type: boolvector
Code example:
-- This is a system function and cannot be called by SQL statements.
bool_vector_typmod_in
Function: Specifies the boolvector input type modifier function.
Input parameter type: cstring[]
Output parameter type: integer
Code example:
gaussdb=# SELECT bool_vector_typmod_in( '{1}' );
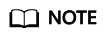
- The vector data type does not support null, nan, or inf as elements. If a vector contains NULL values, the database reports an error.
- When a vector data type is inserted, NULL cannot be used as the inserted value. When a NULL value is inserted as the vector data, the database reports an error.
- Elements of the boolvector type can express Boolean data in t(T)/f(F), y(Y)/n(N), 1/0, true/false, yes/no, or on/off mode.
- The boolvector type applies only to the equal-to operation and does not support comparison operations.
gs_vector_index_options
Function: Displays the hyperparameter values of related vector indexes.
Input parameter type: text
Output parameter type: text
Code example:
-- Create a table. gaussdb=# CREATE TABLE t1 (id int unique,repr floatvector(960)) with (storage_type=astore); Insert data. gaussdb=# copy t1 from '/data/gist1w.txt' delimiter '^'; Create an index. gaussdb=# CREATE INDEX test1v on t1 using gsdiskann (repr l2) with (pq_nseg=120,pq_nclus=64,queue_size=120,num_parallels=30,enable_pq=true,using_clustering_for_parallel=false); gaussdb=# SELECT gs_vector_index_options('test1v');
Operators for vector calculation focus on measuring similarity between vectors and binary operators of vectors.
<->
Function: Calculates the Euclidean distance (L2) between two vectors (floatvector).
Left parameter type: floatvector
Right parameter type: floatvector
Return type: double precision
Code example:
gaussdb=# SELECT floatvector('[1,1,3,2]') <-> floatvector('[1,1,3,2]'); gaussdb=# SELECT '[1,2,3,2]'<-> floatvector('[1,1,3,2]');
<+>
Function: Calculates the cosine distance between two vectors (floatvector).
Left parameter type: floatvector
Right parameter type: floatvector
Return type: double precision
Code example:
gaussdb=# SELECT floatvector('[1,1,3,2]') <+> floatvector('[1,1,3,2]'); gaussdb=# SELECT '[1,2,3,2]'<+> floatvector('[1,1,3,2]');
<#>
Function: Calculates the Hamming distance between two vectors (floatvector).
Left parameter type: boolvector
Right parameter type: boolvector
Return type: double precision
Code example:
gaussdb=# SELECT boolvector('[1,0,1,0]') <#> boolvector('[1,1,1,0]'); gaussdb=# SELECT '[1,0,1,0]'<#> boolvector('[1,1,1,0]');
+
Function: Calculates the bitwise addition of two vectors with the same dimension.
Left parameter type: floatvector
Right parameter type: floatvector
Return type: floatvector
Code example:
gaussdb=# SELECT floatvector('[1,1,3,2]') + floatvector('[1,1,3,2]'); gaussdb=# SELECT '[1,2,3,2]'+ floatvector('[1,1,3,2]');
-
Function: Calculates the bitwise subtraction of two vectors with the same dimension.
Left parameter type: floatvector
Right parameter type: floatvector
Return type: floatvector
Code example:
gaussdb=# SELECT floatvector('[1,1,3,2]') + floatvector('[1,1,3,2]'); gaussdb=# SELECT '[1,2,3,2]'+ floatvector('[1,1,3,2]');
<
Function: Compares the lexicographical order of two vectors with the same dimension.
Left parameter type: floatvector
Right parameter type: floatvector
Return type: Boolean
Code example:
gaussdb=# SELECT floatvector('[1,1,3,2]') < floatvector('[1,1,3,2]'); gaussdb=# SELECT '[1,2,3,2]'< floatvector('[1,1,3,2]');
<=
Function: Compares the lexicographical order of two vectors with the same dimension.
Left parameter type: floatvector
Right parameter type: floatvector
Return type: Boolean
Code example:
gaussdb=# SELECT floatvector('[1,1,3,2]') <= floatvector('[1,1,3,2]'); gaussdb=# SELECT '[1,2,3,2]'<= floatvector('[1,1,3,2]');
>
Function: Compares the lexicographical order of two vectors with the same dimension.
Left parameter type: floatvector
Right parameter type: floatvector
Return type: Boolean
Code example:
gaussdb=# SELECT floatvector('[1,1,3,2]') > floatvector('[1,1,3,2]'); gaussdb=# SELECT '[1,2,3,2]'> floatvector('[1,1,3,2]');
>=
Function: Compares the lexicographical order of two vectors with the same dimension.
Left parameter type: floatvector
Right parameter type: floatvector
Return type: Boolean
Code example:
gaussdb=# SELECT floatvector('[1,1,3,2]') >= floatvector('[1,1,3,2]'); gaussdb=# SELECT '[1,2,3,2]'>= floatvector('[1,1,3,2]');
=
Scenario 1:
Function: Determines whether two vectors with the same dimension are equal.
Left parameter type: floatvector
Right parameter type: floatvector
Return type: Boolean
Code example:
gaussdb=# SELECT floatvector('[1,1,3,2]') = floatvector('[1,1,3,2]'); gaussdb=# SELECT '[1,2,3,2]'= floatvector('[1,1,3,2]');
Scenario 2:
Function: Determines whether two Boolean vectors with the same dimension are consistent.
Left parameter type: boolvector
Right parameter type: boolvector
Return type: Boolean
Code example:
gaussdb=# SELECT boolvector('[1,0,1,0]') = boolvector('[1,1,1,0]'); gaussdb=# SELECT '[1,0,1,0]' = boolvector('[1,1,1,0]');
<>
Function: Determines whether two vectors with the same dimension are unequal.
Left parameter type: floatvector
Right parameter type: floatvector
Return type: Boolean
Code example:
gaussdb=# SELECT floatvector('[1,1,3,2]') <> floatvector('[1,1,3,2]'); gaussdb=# SELECT '[1,2,3,2]'<> floatvector('[1,1,3,2]');
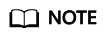
If the vector element value is large, the intermediate calculation result may overflow the single-precision range during distance calculation. As a result, the distance result is NAN or INF.
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot