Signing Browser-Based Upload Requests
Function
OBS supports browser-based uploads using POST requests. Authenticating such a request uses the signature carried in the form. Before calculating the signature for a POST request, you need to first create a security policy. This policy is used to restrict what is allowed in the browser-based upload request. For example, you can specify the prefix of an object to be uploaded must start with prefix01 to make it easier to manage objects. The procedure is as follows:
- Create a policy that specifies the conditions to restrict what you want to allow in the request, such as the bucket name and object name prefix.
- Calculate a signature based on the policy.
- Create a form that contains a valid signature and the policy. The created form is used to upload objects.
Step 1: Creating a Policy
The elements and syntax of a policy are as shown below. The example policy here allows users to upload objects prefixed with user/ to the bucket book before 12:00 on December 31, 2024. The objects uploaded must allow public-read and the x-obs-security-token request header must be YwkaRTbdY8g7q.....
1 2 3 4 5 6 7 8 |
{ "expiration": "2024-12-31T12:00:00.000Z", "conditions": [ {"x-obs-acl": "public-read" }, {"x-obs-security-token": "YwkaRTbdY8g7q...." }, {"bucket": "book" }, ["starts-with", "$key", "user/"] ] } |
A policy consists of Expiration and Conditions.
Expiration
Parameter |
Type |
Mandatory (Yes/No) |
Description |
---|---|---|---|
Expiration |
String |
Yes |
Explanation: When a signature expires. In the example above, "expiration": "2024-12-31T12:00:00.000Z" indicates that the signature becomes invalid after 12:00 on December 31, 2024. Restrictions: The value must be a UTC time in ISO 8601. Its format can be "yyyy-MM-dd'T'HH:mm:ss'Z'" or "yyyy-MM-dd'T'HH:mm:ss.SSS'Z'". Value range: None Default value: None |
Conditions
You can use conditions to restrict what is allowed in the request. The example above requires the requested bucket name to be book, the object uploaded to use user/ as the name prefix, and the ACL of the object to be public-read. A policy can restrict all form fields except AccessKeyId, Signature, file, policy, token, and field names that have an x-ignore- prefix. The following table lists the supported condition elements.
Element |
Type |
Description |
Match Type |
||
---|---|---|---|---|---|
x-obs-acl |
String |
The ACL that must be used in the request. |
Exact Matches starts-with |
||
content-length-range |
int |
The maximum and minimum allowable size for the uploaded content. Example:
|
Specifying Ranges |
||
Cache-Control, Content-Type, Content-Disposition, Content-Encoding, Expires |
String |
REST-specific headers. |
Exact Matches starts-with |
||
key |
String |
The acceptable key name of the uploaded object. |
Exact Matches starts-with |
||
bucket |
String |
The acceptable bucket name. |
Exact Matches |
||
success_action_redirect |
String |
The URL that the client is redirected to after a successful upload. For details, see Uploading an Object - POST. |
Exact Matches starts-with |
||
success_action_status |
String |
The status code returned to the client upon successful upload if success_action_redirect is not specified. For details, see Uploading an Object - POST. |
Exact Matches |
||
x-obs-meta-* |
String |
User-defined metadata. Keywords in this element cannot include non-ASCII or unrecognizable characters. If such characters are necessary, they must be encoded and decoded on the client side in either URL or Base64. The server does not perform decoding. |
Exact Matches starts-with |
||
x-obs-* |
String |
Other headers prefixed with x-obs-. |
Exact Matches starts-with |
||
x-obs-security-token |
String |
A security token. This header is mandatory if you are using a temporary AK/SK and security token for authentication. For details about how to obtain a temporary access key and security token, see Obtaining a Temporary Access Key and Security Token Through a Token. |
Exact Matches |
The table below describes the supported condition matching types:
Condition Match Type |
Description |
||||
---|---|---|---|---|---|
Exact Matches |
The default type. The form field value must match the value specified in conditions. This example indicates the object ACL must be set to public-read:
This example is an alternate way to indicate that the ACL must be set to public-read:
|
||||
Starts With |
The form field value must start with the specified value. This example indicates the object key must start with user/:
|
||||
Matching Any Content |
To allow any content within a form field, use "starts-with" with an empty value (""). This example allows any value for success_action_redirect:
|
||||
Specifying Ranges |
Only used to restrict the size of the uploaded file. Separate the upper and lower limits with a comma (,). Quotation marks are not allowed for element values. This example allows a file size from 1 to 10 MB, that is, from 1048576 to 10485760:
|

Policies use the JSON format. Use curly brackets ({}) or square brackets ([]) to specify conditions. Curly brackets ({}) can enclose a key and a value separated by a colon (:). Square brackets ([]) can contain a condition type, key, and value separated by commas (,). Use the dollar sign ($) ahead of a key to mark a variable.
The table below lists the characters that must be escaped in a policy.
Escape Sequence |
Description |
---|---|
\\ |
Backslash |
\$ |
Dollar symbol |
\b |
Backspace |
\f |
Form feed |
\n |
New line |
\r |
Carriage return |
\t |
Horizontal tab |
\v |
Vertical tab |
\uxxxx |
All Unicode characters |
Step 2: Calculating a Signature
The following table shows the ways to calculate a form-carried signature:
Method |
Description |
Link |
---|---|---|
Using SDKs |
All available OBS SDKs provide automatic calculation. Save time by using them directly. |
|
Manually calculating a signature |
You can manually calculate a signature based on the provided signing algorithm. |
Using SDKs for Signing
Using SDKs |
Signature Source File |
---|---|
Java |
|
Python |
|
Go |
|
C |
- |
Node.Js |
|
Browser.Js |
|
PHP |
|
.NET |
- |
Using a Signing Algorithm
Use the following algorithm to calculate a signature carried in a form:
Signature = Base64( HMAC-SHA1( YourSecretAccessKeyID, StringToSign ) ) StringToSign = Base64( UTF-8-Encoding-Of( policy ) )
The process of calculating a signature is as follows:
- Construct the StringToSign by encoding the created policy in UTF8 and then in Base64.
- Use the SK to calculate the HMAC-SHA1 of the result from step 1.
- Base64 encode the result from step 2 to obtain the signature.
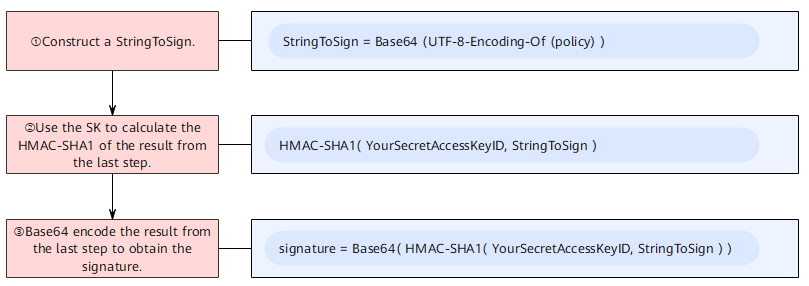
Code Examples
The following are some code examples for calculating a signature carried in a form:
Java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 |
import java.text.SimpleDateFormat;↵ import java.util.ArrayList;↵ import java.util.Base64;↵ import java.util.Collections;↵ import java.util.Date;↵ import java.util.List;↵ import java.util.TimeZone;↵ ↵ import javax.crypto.Mac;↵ import javax.crypto.spec.SecretKeySpec;↵ ↵ public class SignDemo {↵ ↵ private static final String DEFAULT_ENCODING = "UTF-8";↵ private static final String EXPIRATION_DATE_FORMATTER = "yyyy-MM-dd'T'HH:mm:ss.SSS'Z'";↵ private static final TimeZone GMT_TIMEZONE = TimeZone.getTimeZone("GMT");↵ private static final long DEFAULT_EXPIRE_SECONDS = 300;↵ ↵ private String ak;↵ private String sk;↵ ↵ private String join(List items) {↵ StringBuilder sb = new StringBuilder();↵ for (int i = 0; i < items.size(); i++) { String item = items.get(i).toString();sb.append(item); if (i < items.size() - 1) { sb.append(","); } } return sb.toString(); } // Construct a StringToSign. private String stringToSign(String[] tmpConditions, String expiration) { List↵conditions = new ArrayList<>(); Collections.addAll(conditions, tmpConditions); return "{\"expiration\":" + "\"" + expiration + "\"," + "\"conditions\":[" + join(conditions) + "]}"; } private String getFormatExpiration(Date requestDate, long expires) { requestDate = requestDate != null ? requestDate : new Date(); SimpleDateFormat expirationDateFormat = new SimpleDateFormat(EXPIRATION_DATE_FORMATTER); expirationDateFormat.setTimeZone(GMT_TIMEZONE); Date expiryDate = new Date(requestDate.getTime() + (expires <= 0 ? DEFAULT_EXPIRE_SECONDS : expires) * 1000); return expirationDateFormat.format(expiryDate); } // Calculate the signature. public String postSignature(String policy) throws Exception { byte[] policyBase64 = Base64.getEncoder().encode(policy.getBytes(DEFAULT_ENCODING)); SecretKeySpec signingKey = new SecretKeySpec(this.sk.getBytes(DEFAULT_ENCODING), "HmacSHA1"); Mac mac = Mac.getInstance("HmacSHA1");mac.init(signingKey); return Base64.getEncoder().encodeToString(mac.doFinal(policyBase64)); } public static void main(String[] args) throws Exception { SignDemo demo = new SignDemo(); /* Hard-coded or plaintext AK and SK are risky. For security purposes, encrypt your AK and SK and store them in the configuration file or environment variables. In this example, the AK and SK are stored in environment variables for identity authentication. Before running the code in this example, configure environment variables HUAWEICLOUD_SDK_AK and HUAWEICLOUD_SDK_SK. */ demo.ak = System.getenv("HUAWEICLOUD_SDK_AK"); demo.sk = System.getenv("HUAWEICLOUD_SDK_SK"); String authExpiration = demo.getFormatExpiration(null, 0); String[] tmpConditions = { "{\"bucket\": \"bucketName\" }", "[\"starts-with\", \"$key\", \"obj\"]" }; String policy = demo.stringToSign(tmpConditions, authExpiration); String policyBase64 = Base64.getEncoder().encodeToString(policy.getBytes(DEFAULT_ENCODING)); String signature = demo.postSignature(policy); // Print the signature that carries AccessKeyId, policy, and Signature in a form. System.out.println("authExpiration=" + authExpiration); System.out.println("policy=" + policy); System.out.println("policyBase64=" + policyBase64); System.out.println("Signature=" + signature); // Print the signature that carries token in a form. System.out.println("token=" + demo.ak + ":" + signature + ":" + policyBase64); } }↵ |
Python
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 |
# coding=utf-8 import binascii import hashlib import hmac import os import time from datetime import datetime import pytz class SignatureDemo: EXPIRATION_DATE_FORMATTER = "%Y-%m-%dT%H:%M:%S.%f" DEFAULT_ENCODING = "UTF-8" # Set the default expiration time to 300 (5 minutes). DEFAULT_EXPIRE_SECONDS = 300 GMT_TIMEZONE = "GMT" def __init__(self, ak=None, sk=None): self.ak = ak self.sk = sk # Specify request_date and expires as timestamps, for example, 1675651495.979. def get_format_expiration(self, request_date, expires): request_date = request_date if request_date else time.time() expiry_date = request_date + (expires if expires > 0 else self.DEFAULT_EXPIRE_SECONDS) expiration = datetime.fromtimestamp(expiry_date, pytz.timezone(self.GMT_TIMEZONE)).strftime( self.EXPIRATION_DATE_FORMATTER)[:-3] + "Z" return expiration def post_signature(self, policy): # If binascii or encode("base64") is used, newline characters must be removed. policy_base64 = binascii.b2a_base64(policy.encode(self.DEFAULT_ENCODING)).rstrip() hashed = hmac.new(self.sk.encode(self.DEFAULT_ENCODING), policy_base64, hashlib.sha1) return binascii.b2a_base64(hashed.digest()).rstrip() @staticmethod def string_to_sign(conditions, expiration): return "{\"expiration\":" + "\"" + expiration + "\"," + "\"conditions\":[" + ",".join(conditions) + "]}" if __name__ == "__main__": demo = SignatureDemo() # Hard-coded or plaintext AK and SK are risky. For security purposes, encrypt your AK and SK and store them in the configuration file or environment variables. # In this example, the AK and SK are stored in environment variables used for identity authentication. Before running the code in this example, configure environment variables HUAWEICLOUD_SDK_AK and HUAWEICLOUD_SDK_SK. demo.ak = os.getenv('HUAWEICLOUD_SDK_AK') demo.sk = os.getenv('HUAWEICLOUD_SDK_SK') auth_expiration = demo.get_format_expiration(None, 0) conditions_example = [ "{\"bucket\": \"bucketName\" }", "[\"starts-with\", \"$key\", \"obj\"]" ] post_policy = demo.string_to_sign(conditions_example, auth_expiration) policy_base64 = binascii.b2a_base64(post_policy.encode(demo.DEFAULT_ENCODING)).rstrip() signature = demo.post_signature(post_policy) # Print the signature that carries AccessKeyId, policy, and signature in a form. print("authExpiration=" + auth_expiration) print("policy=" + post_policy) print("policyBase64=" + policy_base64) print("Signature=" + signature) # Print the signature that carries token in a form. print("token=" + demo.ak + ":" + signature + ":" + policy_base64) |
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot