Upgrading a Cluster
Function
Cluster upgrade
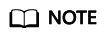
-
Cluster upgrade involves many operations on components. You are advised to upgrade your cluster on the CCE console, a more interactive and intuitive way to reduce operational risks.
-
Currently, cluster upgrade APIs are available only upon request.
Calling Method
For details, see Calling APIs.
URI
POST /api/v3/projects/{project_id}/clusters/{cluster_id}/operation/upgrade
Parameter |
Mandatory |
Type |
Description |
---|---|---|---|
project_id |
Yes |
String |
Details: Project ID. For details about how to obtain the value, see How to Obtain Parameters in the API URI. Constraints: None Options: Project IDs of the account Default value: N/A |
cluster_id |
Yes |
String |
Details: Cluster ID. For details about how to obtain the value, see How to Obtain Parameters in the API URI. Constraints: None Options: Cluster IDs Default value: N/A |
Request Parameters
Parameter |
Mandatory |
Type |
Description |
---|---|---|---|
metadata |
Yes |
Details: Cluster upgrade metadata information Constraints: None |
|
spec |
Yes |
UpgradeSpec object |
Details: Upgrade configuration information. CCE upgrades a cluster based on spec. Constraints: None |
Parameter |
Mandatory |
Type |
Description |
---|---|---|---|
apiVersion |
Yes |
String |
Details: API version Constraints: The value is fixed. Options:
|
kind |
Yes |
String |
Details: API type Constraints: The value is fixed. Options:
|
Parameter |
Mandatory |
Type |
Description |
---|---|---|---|
clusterUpgradeAction |
No |
ClusterUpgradeAction object |
Details: Detailed configuration information about the cluster upgrade Constraints: None |
Parameter |
Mandatory |
Type |
Description |
---|---|---|---|
addons |
No |
Array of UpgradeAddonConfig objects |
Details: Add-on configuration list. CCE upgrades add-ons based on the configuration during the cluster upgrade. Constraints: None |
nodeOrder |
No |
Map<String,Array<NodePriority>> |
Details: Upgrade sequence of nodes in a node pool. key indicates the node pool ID. The value for the default node pool is DefaultPool. Constraints: None |
nodePoolOrder |
No |
Map<String,Integer> |
Details: Upgrade sequence of a node pool, in key-value pairs. key indicates the node pool ID. The value for the default node pool is DefaultPool. value indicates the node pool priority. The default value is 0, which indicates the lowest priority. A larger value indicates a higher priority. Constraints: None |
strategy |
Yes |
UpgradeStrategy object |
Details: Cluster upgrade policy Constraints: None |
targetVersion |
Yes |
String |
Details: Target cluster version, for example, v1.23 Constraints: You can only upgrade a cluster to a later version. Do not enter a value that is equal to or earlier than the current cluster version. Options: Supported cluster versions |
Parameter |
Mandatory |
Type |
Description |
---|---|---|---|
addonTemplateName |
Yes |
String |
Details: CCE add-on name Constraints: None Options: Names of the add-ons installed in the cluster. |
operation |
Yes |
String |
Details: Action for upgrading an add-on Constraints: None Options: patch: specifies that the add-on version will be upgraded. |
version |
Yes |
String |
Details: Target add-on version Constraints: The target add-on version must match the target cluster version. Options: N/A |
values |
No |
Map<String,Object> |
Details: Add-on parameter list, in key-value pairs Constraints: None |
Parameter |
Mandatory |
Type |
Description |
---|---|---|---|
nodeSelector |
Yes |
NodeSelector object |
Details: Node label selector, which selects a batch of nodes Constraints: Only labels on the nodes are allowed. |
priority |
Yes |
Integer |
Details: Priority of the current batch of nodes. A larger value indicates a higher priority. Constraints: None Options: Positive integers Default value: 0 |
Parameter |
Mandatory |
Type |
Description |
---|---|---|---|
key |
Yes |
String |
Details: Key Constraints: None Options: N/A |
value |
No |
Array of strings |
Details: Tag value list Constraints: None Options: N/A |
operator |
Yes |
String |
Details: Logical operators of labels Constraints: None Options:
|
Parameter |
Mandatory |
Type |
Description |
---|---|---|---|
type |
Yes |
String |
Details: Upgrade policy type Constraints: None Options:
|
inPlaceRollingUpdate |
No |
InPlaceRollingUpdate object |
Details: Detailed configuration of an in-place upgrade policy Constraints: This parameter is mandatory when the in-place upgrade policy type is specified. |
Parameter |
Mandatory |
Type |
Description |
---|---|---|---|
userDefinedStep |
No |
Integer |
Details: Maximum number of nodes to be upgraded in a batch. Node pools will be upgraded in sequence. Nodes in node pools will be upgraded in batches. One node is upgraded in the first batch, two nodes in the second batch, and the number of nodes to be upgraded in each subsequent batch increases by a power of 2 until the maximum number of nodes to be upgraded in each batch is reached. The next cluster is upgraded after the previous one is upgraded. Constraints: None Options: 1 to 60 Default value: 20 |
scope |
No |
String |
Details: Scope of the node upgrade batch Constraints: None Options: Cluster: If the scope is set to a cluster, the upgrade batch will remain unchanged throughout the entire upgrade process. NodePool: If the scope is set to node pools, the upgrade batch will be reset for each individual node pool. Default value: Cluster |
Response Parameters
Status code: 200
Parameter |
Type |
Description |
---|---|---|
metadata |
Upgrade task metadata |
|
spec |
UpgradeResponseSpec object |
Upgrade settings |
Parameter |
Type |
Description |
---|---|---|
uid |
String |
Upgrade task ID. You can obtain the progress by calling the API for obtaining cluster upgrade task details. |
Parameter |
Type |
Description |
---|---|---|
clusterUpgradeAction |
ClusterUpgradeResponseAction object |
Cluster upgrade settings |
Parameter |
Type |
Description |
---|---|---|
version |
String |
Current cluster version |
targetVersion |
String |
Target cluster version, for example, v1.23. |
targetPlatformVersion |
String |
Platform version of the target cluster, which is an internal version of the cluster version and cannot be specified. |
strategy |
UpgradeStrategyResponse object |
Upgrade policies |
config |
Object |
Cluster configuration specified during an upgrade |
Parameter |
Type |
Description |
---|---|---|
type |
String |
Upgrade policy type |
inPlaceRollingUpdate |
InPlaceRollingUpdateResponse object |
Detailed configuration of an in-place upgrade policy |
Example Requests
Upgrade the cluster to v1.23 and set the node upgrade step to 20.
POST /api/v3/projects/{project_id}/clusters/{cluster_id}/operation/upgrade { "metadata" : { "apiVersion" : "v3", "kind" : "UpgradeTask" }, "spec" : { "clusterUpgradeAction" : { "strategy" : { "type" : "inPlaceRollingUpdate", "inPlaceRollingUpdate" : { "userDefinedStep" : 20 } }, "targetVersion" : "v1.23" } } }
Example Responses
Status code: 200
Cluster upgrade requested.
{ "metadata" : { "uid" : "976a33e2-f545-11ed-87af-0255ac1002c2" }, "spec" : { "clusterUpgradeAction" : { "version" : "v1.19.16-r20", "targetVersion" : "v1.23.8-r0", "targetPlatformVersion" : "cce.10", "strategy" : { "type" : "inPlaceRollingUpdate", "inPlaceRollingUpdate" : { "userDefinedStep" : 20 } }, "config" : { } } } }
SDK Sample Code
The SDK sample code is as follows.
Upgrade the cluster to v1.23 and set the node upgrade step to 20.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 |
package com.huaweicloud.sdk.test; import com.huaweicloud.sdk.core.auth.ICredential; import com.huaweicloud.sdk.core.auth.BasicCredentials; import com.huaweicloud.sdk.core.exception.ConnectionException; import com.huaweicloud.sdk.core.exception.RequestTimeoutException; import com.huaweicloud.sdk.core.exception.ServiceResponseException; import com.huaweicloud.sdk.cce.v3.region.CceRegion; import com.huaweicloud.sdk.cce.v3.*; import com.huaweicloud.sdk.cce.v3.model.*; public class UpgradeClusterSolution { public static void main(String[] args) { // The AK and SK used for authentication are hard-coded or stored in plaintext, which has great security risks. It is recommended that the AK and SK be stored in ciphertext in configuration files or environment variables and decrypted during use to ensure security. // In this example, AK and SK are stored in environment variables for authentication. Before running this example, set environment variables CLOUD_SDK_AK and CLOUD_SDK_SK in the local environment String ak = System.getenv("CLOUD_SDK_AK"); String sk = System.getenv("CLOUD_SDK_SK"); String projectId = "{project_id}"; ICredential auth = new BasicCredentials() .withProjectId(projectId) .withAk(ak) .withSk(sk); CceClient client = CceClient.newBuilder() .withCredential(auth) .withRegion(CceRegion.valueOf("<YOUR REGION>")) .build(); UpgradeClusterRequest request = new UpgradeClusterRequest(); request.withClusterId("{cluster_id}"); UpgradeClusterRequestBody body = new UpgradeClusterRequestBody(); InPlaceRollingUpdate inPlaceRollingUpdateStrategy = new InPlaceRollingUpdate(); inPlaceRollingUpdateStrategy.withUserDefinedStep(20); UpgradeStrategy strategyClusterUpgradeAction = new UpgradeStrategy(); strategyClusterUpgradeAction.withType("inPlaceRollingUpdate") .withInPlaceRollingUpdate(inPlaceRollingUpdateStrategy); ClusterUpgradeAction clusterUpgradeActionSpec = new ClusterUpgradeAction(); clusterUpgradeActionSpec.withStrategy(strategyClusterUpgradeAction) .withTargetVersion("v1.23"); UpgradeSpec specbody = new UpgradeSpec(); specbody.withClusterUpgradeAction(clusterUpgradeActionSpec); UpgradeClusterRequestMetadata metadatabody = new UpgradeClusterRequestMetadata(); metadatabody.withApiVersion("v3") .withKind("UpgradeTask"); body.withSpec(specbody); body.withMetadata(metadatabody); request.withBody(body); try { UpgradeClusterResponse response = client.upgradeCluster(request); System.out.println(response.toString()); } catch (ConnectionException e) { e.printStackTrace(); } catch (RequestTimeoutException e) { e.printStackTrace(); } catch (ServiceResponseException e) { e.printStackTrace(); System.out.println(e.getHttpStatusCode()); System.out.println(e.getRequestId()); System.out.println(e.getErrorCode()); System.out.println(e.getErrorMsg()); } } } |
Upgrade the cluster to v1.23 and set the node upgrade step to 20.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 |
# coding: utf-8 import os from huaweicloudsdkcore.auth.credentials import BasicCredentials from huaweicloudsdkcce.v3.region.cce_region import CceRegion from huaweicloudsdkcore.exceptions import exceptions from huaweicloudsdkcce.v3 import * if __name__ == "__main__": # The AK and SK used for authentication are hard-coded or stored in plaintext, which has great security risks. It is recommended that the AK and SK be stored in ciphertext in configuration files or environment variables and decrypted during use to ensure security. # In this example, AK and SK are stored in environment variables for authentication. Before running this example, set environment variables CLOUD_SDK_AK and CLOUD_SDK_SK in the local environment ak = os.environ["CLOUD_SDK_AK"] sk = os.environ["CLOUD_SDK_SK"] projectId = "{project_id}" credentials = BasicCredentials(ak, sk, projectId) client = CceClient.new_builder() \ .with_credentials(credentials) \ .with_region(CceRegion.value_of("<YOUR REGION>")) \ .build() try: request = UpgradeClusterRequest() request.cluster_id = "{cluster_id}" inPlaceRollingUpdateStrategy = InPlaceRollingUpdate( user_defined_step=20 ) strategyClusterUpgradeAction = UpgradeStrategy( type="inPlaceRollingUpdate", in_place_rolling_update=inPlaceRollingUpdateStrategy ) clusterUpgradeActionSpec = ClusterUpgradeAction( strategy=strategyClusterUpgradeAction, target_version="v1.23" ) specbody = UpgradeSpec( cluster_upgrade_action=clusterUpgradeActionSpec ) metadatabody = UpgradeClusterRequestMetadata( api_version="v3", kind="UpgradeTask" ) request.body = UpgradeClusterRequestBody( spec=specbody, metadata=metadatabody ) response = client.upgrade_cluster(request) print(response) except exceptions.ClientRequestException as e: print(e.status_code) print(e.request_id) print(e.error_code) print(e.error_msg) |
Upgrade the cluster to v1.23 and set the node upgrade step to 20.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 |
package main import ( "fmt" "github.com/huaweicloud/huaweicloud-sdk-go-v3/core/auth/basic" cce "github.com/huaweicloud/huaweicloud-sdk-go-v3/services/cce/v3" "github.com/huaweicloud/huaweicloud-sdk-go-v3/services/cce/v3/model" region "github.com/huaweicloud/huaweicloud-sdk-go-v3/services/cce/v3/region" ) func main() { // The AK and SK used for authentication are hard-coded or stored in plaintext, which has great security risks. It is recommended that the AK and SK be stored in ciphertext in configuration files or environment variables and decrypted during use to ensure security. // In this example, AK and SK are stored in environment variables for authentication. Before running this example, set environment variables CLOUD_SDK_AK and CLOUD_SDK_SK in the local environment ak := os.Getenv("CLOUD_SDK_AK") sk := os.Getenv("CLOUD_SDK_SK") projectId := "{project_id}" auth := basic.NewCredentialsBuilder(). WithAk(ak). WithSk(sk). WithProjectId(projectId). Build() client := cce.NewCceClient( cce.CceClientBuilder(). WithRegion(region.ValueOf("<YOUR REGION>")). WithCredential(auth). Build()) request := &model.UpgradeClusterRequest{} request.ClusterId = "{cluster_id}" userDefinedStepInPlaceRollingUpdate:= int32(20) inPlaceRollingUpdateStrategy := &model.InPlaceRollingUpdate{ UserDefinedStep: &userDefinedStepInPlaceRollingUpdate, } strategyClusterUpgradeAction := &model.UpgradeStrategy{ Type: "inPlaceRollingUpdate", InPlaceRollingUpdate: inPlaceRollingUpdateStrategy, } clusterUpgradeActionSpec := &model.ClusterUpgradeAction{ Strategy: strategyClusterUpgradeAction, TargetVersion: "v1.23", } specbody := &model.UpgradeSpec{ ClusterUpgradeAction: clusterUpgradeActionSpec, } metadatabody := &model.UpgradeClusterRequestMetadata{ ApiVersion: "v3", Kind: "UpgradeTask", } request.Body = &model.UpgradeClusterRequestBody{ Spec: specbody, Metadata: metadatabody, } response, err := client.UpgradeCluster(request) if err == nil { fmt.Printf("%+v\n", response) } else { fmt.Println(err) } } |
For SDK sample code of more programming languages, see the Sample Code tab in API Explorer. SDK sample code can be automatically generated.
Status Codes
Status Code |
Description |
---|---|
200 |
Cluster upgrade requested. |
Error Codes
See Error Codes.
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot