Help Center>
IoT Device Access>
User Guide>
Message Communications>
Data Reporting>
Device Reporting Properties>
Property Reporting Example
Updated on 2024-04-29 GMT+08:00
Property Reporting Example
Java SDK Usage
This document describes how to use the Java SDK for the development of property reporting.
Development Environment Requirements
JDK 1.8 or later has been installed.
Defining a Product Model
The reported properties must match the properties defined in the product model corresponding to the device. The following figure provides the information of example product model used in the SDK code.
Figure 1 Product property definition
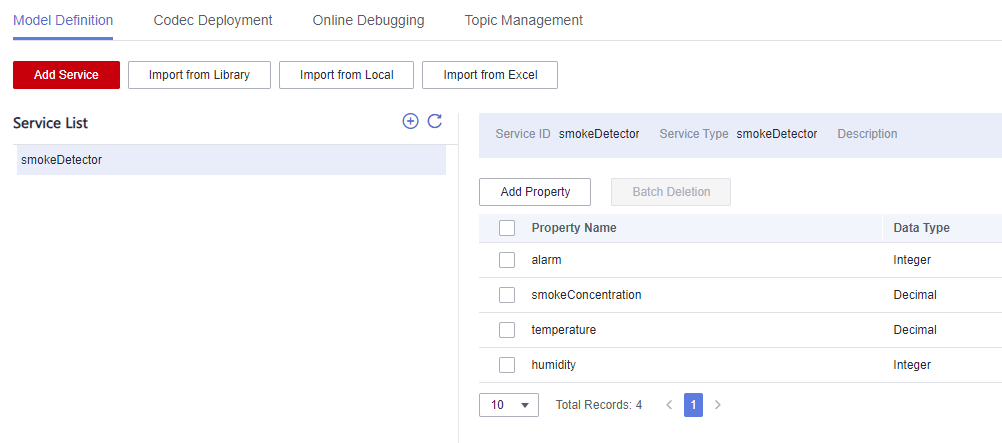
Configuring the SDK on Devices
- Download an SDK.
- Configure the Maven dependency of the SDK on devices.
<dependency> <groupId>com.huaweicloud</groupId> <artifactId>iot-device-sdk-java</artifactId> <version>1.1.4</version> </dependency>
- Configure the SDK and device connection parameters on devices.
// Load the CA certificate of the IoT platform. For details about how to obtain the certificate, visit https://support.huaweicloud.com/intl/en-us/devg-iothub/iot_02_1004.html#section3. URL resource = BroadcastMessageSample.class.getClassLoader().getResource("ca.jks"); File file = new File(resource.getPath()); // The format is ssl://Domain name:Port number. // To obtain the domain name, log in to the Huawei Cloud IoTDA console. In the navigation pane, choose Overview and click Access Details in the Instance Information area. Select the access domain name corresponding to port 8883. String serverUrl = "ssl://localhost:8883"; // Device ID created on the IoT platform String deviceId = "deviceId"; // Secret corresponding to the device ID String deviceSecret = "secret"; // Create a device. IoTDevice device = new IoTDevice(serverUrl, deviceId, deviceSecret, file); if (device.init() != 0) { return; }
- Reporting Device Properties
Map<String ,Object> json = new HashMap<>(); Random rand = new Random(); // Set properties based on the product model. json.put("alarm", alarm); json.put("temperature", rand.nextFloat()*100.0f); json.put("humidity", rand.nextFloat()*100.0f); json.put("smokeConcentration", rand.nextFloat() * 100.0f); ServiceProperty serviceProperty = new ServiceProperty(); serviceProperty.setProperties(json); serviceProperty.setServiceId("smokeDetector");// The service ID must be consistent with that defined in the product model. device.getClient().reportProperties(Arrays.asList(serviceProperty), new ActionListener() { @Override public void onSuccess(Object context) { log.info("reportProperties success" ); } @Override public void onFailure(Object context, Throwable var2) { log.error("reportProperties failed" + var2.toString()); } });
Testing and Verification
- Run the SDK code on the device. The following figure provides an example of the property reporting log.
Figure 2 Java SDK property reporting result log
- Log in to the IoTDA console, choose Devices > All Devices, and select a device to access its details page. The latest reported data is displayed on the overview tab page.
Figure 3 Platform receiving and parsing the reported device properties
Parent topic: Device Reporting Properties
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
The system is busy. Please try again later.
For any further questions, feel free to contact us through the chatbot.
Chatbot