Internal Functions
Description
Huawei Cloud IoTDA provides multiple internal functions to use in templates. This section introduces these functions, including the input parameter type, parameter length, and return value type.
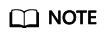
- The entire function must be in valid JSON format.
- In a function, the variable placeholders (${}) or the Ref function can be used to reference the value defined by the input parameter.
- The parameters used by the function must be declared in the template.
- A function with a single input parameter is followed by a parameter, for example, "Fn::Base64Decode": "${iotda::mqtt::username}".
- A function with multiple input parameters is followed by an array, for example, "Fn::HmacSHA256": ["${iotda::mqtt::username}", "${iotda::device::secret}"].
- Functions can be nested. That is, the parameter of a function can be another function. Note that the return value of a nested function must match its parameter type in the outer function, for example, {"Fn::HmacSHA256": ["${iotda::mqtt::username}", {"Fn::Base64Encode": "${iotda::device::secret}"}]}.
Fn::ArraySelect
The internal function Fn::ArraySelect returns a string element whose index is index in a string array.
JSON
{"Fn::ArraySelect": [index, [StringArray]]}
Parameter |
Type |
Description |
---|---|---|
index |
int |
Index of an array element. The value is an integer and starts from 0. |
StringArray |
String[] |
String array element. |
Return values |
String |
Element whose index is index. |
Example:
{ "Fn::ArraySelect": [1, ["123", "456", "789"]] } return: "456"
Fn::Base64Decode
The internal function Fn::Base64Decode decodes a string into a byte array using Base64.
JSON
{ "Fn::Base64Decode" : "content" }
Parameter |
Type |
Description |
---|---|---|
content |
String |
String to be decoded. |
Return Values |
byte[] |
Base64-decoded byte array. |
Example:
{ "Fn::Base64Decode": "123456"] } return: d76df8e7 // The value is converted into a hexadecimal string for display.
Fn::Base64Encode
The internal function Fn::Base64Encode encodes a string using Base64.
JSON
{"Fn::Base64Encode": "content"}
Parameter |
Type |
Description |
---|---|---|
content |
String |
String to be encoded. |
Return Values |
String |
Base64-encoded string. |
Example:
{ "Fn::Base64Encode": "testvalue" } return: "dGVzdHZhbHVl"
Fn::GetBytes
The internal function Fn::GetBytes returns a byte array encoded from a string using UTF-8.
JSON
{"Fn::GetBytes": "content"}
Parameter |
Type |
Description |
---|---|---|
content |
String |
String to be encoded. |
Return Values |
byte[] |
Byte array converted from a string encoded using UTF-8. |
Example:
{ "Fn::GetBytes": "testvalue" } return: "7465737476616c7565" // The value is converted into a hexadecimal string for display.
Fn::HmacSHA256
The internal function Fn::HmacSHA256 encrypts a string using the HMACSHA256 algorithm based on a given secret.
JSON
{"Fn::HmacSHA256": ["content", "secret"]}
Parameter |
Type |
Description |
---|---|---|
content |
String |
String to be encrypted. |
secret |
String or byte[] |
Secret key, which can be a string or byte array. |
Return Values |
String |
Value encrypted using the HMACSHA256 algorithm. |
Example:
{ "Fn::HmacSHA256": ["testvalue", "123456"] } return: "0f9fb47bd47449b6ffac1be951a5c18a7eff694940b1a075b973ff9054a08be3"
Fn::Join
The internal function Fn::Join can concatenate up to 10 strings into one string.
JSON
{"Fn::Join": ["element", "element"...]}
Parameter |
Type |
Description |
---|---|---|
element |
String |
String to be concatenated. |
Return Values |
String |
String obtained by concatenating substrings. |
Example:
{ "Fn::Join": ["123", "456", "789"] } return: "123456789"
Fn::MathAdd
The internal function Fn::MathAdd performs mathematical addition on two integers.
JSON
{"Fn::MathAdd": [X, Y]}
Parameter |
Type |
Description |
---|---|---|
X |
long |
Augend. |
Y |
long |
Addend. |
Return Values |
long |
Sum of X and Y. |
Example:
{ "Fn::MathAdd": [1, 1] } return: 2
Fn::MathDiv
The internal function Fn::MathDiv performs a mathematical division on two integers.
JSON
{"Fn::MathDiv": [X, Y]}
Parameter |
Type |
Description |
---|---|---|
X |
long |
Dividend. |
Y |
long |
Divisor. |
Return Values |
long |
Value of X divided by Y. |
Example:
{ "Fn::MathDiv": [10, 2] } return: 5 { "Fn::MathDiv": [10, 3] } return: 3
Fn::MathMod
The internal function Fn::MathMod performs the mathematical modulo on two integers.
JSON
{"Fn::MathMod": [X, Y]}
Parameter |
Type |
Description |
---|---|---|
X |
long |
Dividend. |
Y |
long |
Divisor. |
Return Values |
long |
Residue of X modulo Y. |
Example:
{ "Fn::MathMod": [10, 3] } return: 1
Fn::MathMultiply
The internal function Fn::MathMultiply performs mathematical multiplication on two integers.
JSON
{"Fn::MathMultiply": [X, Y]}
Parameter |
Type |
Description |
---|---|---|
X |
long |
Multiplicand. |
Y |
long |
Multiplier. |
Return Values |
long |
Value of X multiplied by Y. |
Example:
{ "Fn::MathMultiply": [3, 3] } return: 9
Fn::MathSub
The internal function Fn::MathSub performs mathematical subtraction on two integers.
JSON
{"Fn::MathSub": [X, Y]}
Parameter |
Type |
Description |
---|---|---|
X |
long |
Minuend. |
Y |
long |
Subtrahend. |
Return Values |
long |
Value of X minus Y. |
Example:
{ "Fn::MathSub": [9, 3] } return: 6
Fn::ParseLong
The internal function Fn::ParseLong can convert a numeric string into an integer.
JSON
{"Fn::ParseLong": "String"}
Parameter |
Type |
Description |
---|---|---|
String |
String |
String to be converted. |
Return Values |
long |
Value obtained after a string is converted into an integer. |
Example:
{ "Fn::ParseLong": "123" } return: 123
Fn::Split
The internal function Fn::Split splits a string into a string array based on the specified separator.
JSON
{ "Fn::Split" : ["String", "Separator"] }
Parameter |
Type |
Description |
---|---|---|
String |
String |
String to be split. |
Separator |
String |
Separator. |
Return Values |
String[] |
String array obtained after String is split by Separator. |
Example:
{ "Fn::Split": ["a|b|c", "|"] } return: ["a", "b", "c"]
Fn::SplitSelect
The internal function Fn::SplitSelect splits a string into a string array based on the specified separator, and then returns the elements of the specified index in the array.
JSON
{ "Fn::SplitSelect" : ["String", "Separator", index] }
Parameter |
Type |
Description |
---|---|---|
String |
String |
String to be split. |
Separator |
String |
Separator. |
index |
int |
Index value of the target element in the array, starting from 0. |
Return Values |
String |
Substring of the specified index after a string is split by the specified separator. |
Example:
{ "Fn::SplitSelect": ["a|b|c", "|", 1] } return: "b"
Fn::Sub
The internal function Fn::Sub replaces variables in an input string with specified values. You can use this function in a template to construct a dynamic string.
JSON
{ "Fn::Sub" : [ "String", { "Var1Name": Var1Value, "Var2Name": Var2Value } ] }
Parameter |
Type |
Description |
---|---|---|
String |
String |
A string that contains variables. Variables are defined using placeholders (${}). |
VarName |
String |
Variable name, which must be defined in the String parameter. |
VarValue |
String |
Variable value. Function nesting is supported. |
Return Values |
String |
Value of string after replacement in the original String parameter |
Example:
{ "Fn::Sub": ["${token};hmacsha256", { "token": { "Fn::HmacSHA256": ["${iotda::mqtt::username}", { "Fn::Base64Decode": "${iotda::mqtt::client_id}" }] } }] } If: ${iotda::mqtt::username}="test_device_username" ${iotda::device::client_id}="OozqTPlCWTTJjEH/5s+T6w==" return: "0773c4fd6c92902a1b2f4a45fdcdec416b6fc2bc6585200b496e460e2ef31c3d"
Fn::SubStringAfter
The internal function Fn::SubStringAfter truncates the substring after the specified separator in a string.
JSON
{ "Fn::SubStringAfter" : ["content", "separator"] }
Parameter |
Type |
Description |
---|---|---|
content |
String |
String to be truncated. |
separator |
String |
Separator. |
Return Values |
String |
Substring after the specified separator that separates the string. |
Example:
{ "Fn::SubStringAfter": ["content:123456", ":"] ] return: "123456"
Fn::SubStringBefore
The internal function Fn::SubStringBefore truncates the substring before the specified separator in a string.
JSON
{ "Fn::SubStringBefore" : ["content", "separator"] }
Parameter |
Type |
Description |
---|---|---|
content |
String |
String to be truncated. |
separator |
String |
Separator. |
Return Values |
String |
Substring before the specified separator that separates the string. |
Example:
{ "Fn::SubStringBefore": ["content:123456", ":"] ] return: "content"
Ref
The internal function Ref returns the value of the specified referenced parameter. The referenced parameter must be declared in the template.
JSON
{ "Ref" : "paramName" }
Parameter |
Type |
Description |
---|---|---|
paramName |
String |
Name of the referenced parameter. |
Return Values |
String |
Value of the referenced parameter. |
Example:
{ "Ref": "iotda::mqtt::username" } When iotda::mqtt::username="device_123" return: "device_123"
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot