Using Hibernate to Connect to a Database
Hibernate is an object-relational mapping (ORM) tool for the Java programming language. It provides an easy-to-use framework for automatically mapping Java objects to database tables, so that developers can operate databases in object-oriented mode. Hibernate frees developers from manually writing a large amount of SQL and JDBC code, which significantly reduces the development workload of the data access layer.
This section describes how to use Hibernate to connect to a GaussDB database and perform operations in it, for example, creating a table, modifying a table, and inserting, deleting, updating, or querying data in a table.
Configuring the POM Dependency
<dependency> <groupId>com.huaweicloud.gaussdb</groupId> <artifactId>opengaussjdbc</artifactId> <version>503.2.T35</version> </dependency> <dependency> <groupId>org.hibernate</groupId> <artifactId>hibernate-core</artifactId> <version>5.3.7.Final</version> </dependency>
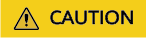
The Maven environment must have been configured before you configure the POM dependency.
Configuring the hibernate.cfg.xml Resource File
<?xml version="1.0" encoding="utf-8"?> <!DOCTYPE hibernate-configuration PUBLIC "-//Hibernate/Hibernate Configuration DTD 3.0//EN" "http://hibernate.sourceforge.net/hibernate-configuration-3.0.dtd"> <hibernate-configuration> <session-factory> <!--GaussDB connection information--> <property name="connection.driver_class">com.huawei.opengauss.jdbc.Driver</property> <property name="connection.url">jdbc:opengauss://***.***.***.*** (Replace ***.***.***.*** with the database IP address.):20000 (Replace 20000 with the database port.)/test? currentSchema=test (Replace test with the target database and schema.)</property> <property name="connection.username">*** (Replace *** with the correct username.)</property> <property name="connection.password">****** (Replace ****** with the correct password.)</property> <!-- The following configurations are optional. --> <!-- Specify whether to support dialects. --> <!-- In PostgreSQL compatibility mode, the following configuration must be set. --> <property name="dialect">org.hibernate.dialect.PostgreSQL82Dialect</property> <!-- Specify whether to print SQL statements when CURD commands are executed. --> <property name="show_sql">true</property> <!--Enable automatic table creation (and update).--> <!-- <property name="hbm2ddl.auto">update</property>--> <!-- <property name="hbm2ddl.auto">create</property>--> <!-- Register resources (entity class mapping file).--> <mapping resource="./student.xml"/> </session-factory> </hibernate-configuration>
Preparing an Entity Class and Entity Class Mapping File
In this example, the Student class and student.xml file are used. The file path is com.howtodoinjava.hibernate.test.dto.
- Create an entity class.
public class Student implements Serializable { int id; String name; // String age; // The constructor, get, and set methods are omitted here. }
- Prepare the student.xml file.
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE hibernate-mapping PUBLIC '-//Hibernate/Hibernate Mapping DTD 3.0//EN' 'http://www.hibernate.org/dtd/hibernate-mapping-3.0.dtd'> <hibernate-mapping> <!-- The mapping between classes and tables is made on the class element. --> <!-- name: fully-qualified class name --> <!-- table: table name --> <class name="com.howtodoinjava.hibernate.test.dto.Student" table="student"> <!-- The mapping of the primary key is made on the id element. --> <!-- name: name of the attribute used as the primary key in the object --> <!-- column: name of the primary key field in the table --> <!-- If the value of name is the same as that of column, column can be omitted. --> <id name="id" column="id"> <!-- Set the class attribute of the generator element to "assigned". The ID must be provided. --> <generator class="assigned" /> <!--** Pay attention to the Hibernate primary key generation policy. **--> </id> <!-- The mapping between attributes and fields is made on the property element. --> <!-- name: attribute name in the class --> <!-- column: field name in the table --> <!-- If the value of name is the same as that of column, column can be omitted. --> <property name="name" /> <!-- Same as the entity class. --> <!--<property name="age"/>--> </class> </hibernate-mapping>
Function Test Example
- Test the connection function.
- Test method:
@Test public void testConnection() { // Load the configuration information. Configuration conf = new Configuration().configure(); // Create the SessionFactory object based on the configuration information. SessionFactory sessionFactory = conf.buildSessionFactory(); // Open a session object related to the database. Session session = sessionFactory.openSession(); System.out.println(session); }
- The breakpoint shows that the connection is successfully established.
Figure 1 Breakpoint debugging
- Test method:
- Enable automatic table creation.
- Comment out the following line in the configuration file:
<property name="hbm2ddl.auto">create</property>
- After deleting the student table from the database, perform the following test method:
@Test public void testCreateTableAndInsertData() { // Create the object to be tested. Student student = new Student(); student.setId(16); student.setName("xiaoming"); // Start a transaction based on session. Configuration conf = new Configuration().configure(); SessionFactory sessionFactory = conf.buildSessionFactory(); Session session = sessionFactory.openSession(); Transaction transaction = session.beginTransaction(); // Use session to save data. session.save(student); // Commit the transaction. transaction.commit(); // After the operation is complete, close the session connection object. session.close(); }
- View the executed SQL statements printed on the console and the result in the GaussDB database.
Figure 2 Executing SQL statementsFigure 3 Query result
The student table is successfully created, and the id = 16, name = "xiaoming" record is inserted into the table.
- Comment out the following line in the configuration file:
- Modify the table (by adding data records).
- Modify the configuration file and configure the path of the schema where the table to be modified is located in the URL.
In this test example, the student table is in schema test under database test. That is, the table path is test.test.student.
<property name="connection.url">jdbc:opengauss://xxx.xxx.xxx.xxx (Replace xxx.xxx.xxx.xxx with the database IP address.):20000 (Replace 20000 with the database port.)/test? currentSchema=test (Replace test with the target database and schema.)</property> <!-- Uncomment update. --> <property name="hbm2ddl.auto">update</property>
- Uncomment the age attribute in the entity class and XML file and execute the following method:
@Test public void testAlterTable() { // Create the object to be tested. Student student = new Student(); student.setId(15); student.setName("xiaohong"); student.setAge("20"); // Start a transaction based on session. Configuration conf = new Configuration().configure(); SessionFactory sessionFactory = conf.buildSessionFactory(); Session session = sessionFactory.openSession(); Transaction transaction = session.beginTransaction(); // Use session to save data. session.save(student); // Commit the transaction. transaction.commit(); // After the operation is complete, close the session connection object. session.close(); }
- View the executed SQL statements printed on the console and the result in the GaussDB database.
Figure 4 Executing SQL statementsFigure 5 Query result
The framework automatically adds data records to the table based on the entity class and XML file.
- Modify the configuration file and configure the path of the schema where the table to be modified is located in the URL.
- Save data into the database.
- Test method:
@Test public void testInsert() { Student s1 = new Student(1,"q"); Student s2 = new Student(2,"w"); Student s3 = new Student(3,"e"); ArrayList<Student> students = new ArrayList<>(); students.add(s1); students.add(s2); students.add(s3); // Start a transaction based on session. Configuration conf = new Configuration().configure(); SessionFactory sessionFactory = conf.buildSessionFactory(); Session session = sessionFactory.openSession(); Transaction transaction = session.beginTransaction(); // Use session to save data. for (Student student : students) { session.save(student); } // Commit the transaction. transaction.commit(); // After the operation is complete, close the session connection object. session.close(); }
- The result is as follows.
Figure 6 Executing SQL statementsFigure 7 Query result
Three rows of data are successfully inserted.
- Test method:
- Query data in HQL mode.
- Test method:
@Test public void testHQL() { // HQL mode Configuration conf = new Configuration().configure(); SessionFactory sessionFactory = conf.buildSessionFactory(); // Create a session. Session session = sessionFactory.openSession(); // Start a transaction. Transaction tx = session.beginTransaction(); // Create an HQL query. String hql = "FROM Student S WHERE S.id = 15"; Query query = session.createQuery(hql); // Execute the query and obtain the result. List results = query.list(); // Commit the transaction. tx.commit(); // End the session. session.close(); }
- The result is as follows.
Figure 8 SQL execution result
- Test method:
- Query data in SQL mode.
- Test method:
@Test public void testQuery() { // Start a transaction based on session. Configuration conf = new Configuration().configure(); SessionFactory sessionFactory = conf.buildSessionFactory(); Session session = sessionFactory.openSession(); Transaction transaction = session.beginTransaction(); // SQL mode List<Student> students = session.createSQLQuery("select * from test.student where id = 1").addEntity(Student.class).list(); for (int i = 0; i < students.size(); i++) { System.out.println(students.get(i)); } students.get(0).setAge("20"); // Commit the transaction. transaction.commit(); // After the operation is complete, close the session connection object. session.close(); }
- The result is as follows.
Figure 9 SQL execution resultFigure 10 Query result
The data record of the student whose ID is 1 is found and the value of age is changed to 20.
- Test method:
- Modify data.
- Test method:
@Test public void testUpdate() { // Start a transaction based on session. Configuration conf = new Configuration().configure(); SessionFactory sessionFactory = conf.buildSessionFactory(); Session session = sessionFactory.openSession(); Transaction transaction = session.beginTransaction(); // SQL mode session.createSQLQuery("update test.student set age = 19 where id = 16").executeUpdate(); // Commit the transaction. transaction.commit(); // After the operation is complete, close the session connection object. session.close(); }
- The result is as follows.
Figure 11 SQL execution resultFigure 12 Query result
The age field of the student whose ID is 16 is successfully changed to 19.
- Test method:
- Delete data.
- Test method:
@Test public void testDelete() { // Start a transaction based on session. Configuration conf = new Configuration().configure(); SessionFactory sessionFactory = conf.buildSessionFactory(); Session session = sessionFactory.openSession(); Transaction transaction = session.beginTransaction(); // SQL mode List<Student> students = session.createSQLQuery("select * from test.student").addEntity(Student.class).list(); System.out.println(students); session.createSQLQuery("delete from test.student where id = " + students.get(0).getId()).executeUpdate(); // Commit the transaction. transaction.commit(); // After the operation is complete, close the session connection object. session.close(); }
- The result is as follows.
Figure 13 SQL execution resultFigure 14 Query result
The record whose ID is 15 has been deleted from the student table.
- Test method:
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot