Using JDBC to Connect to a Database
Java Database Connectivity (JDBC) is a Java API for running SQL statements. It provides unified access APIs for different relational databases, based on which applications process data. The GaussDB library supports JDBC 4.2 and requires JDK 1.8 for code compiling. It does not support JDBC-ODBC bridge.
Prerequisites
Java JDK 8 has been installed on the local PC.
JDBC Package
- gaussdbjdbc.jar: The main class name is com.huawei.gaussdb.jdbc.Driver. The URL prefix of the database connection is jdbc:gaussdb. This driver package is recommended. The Java code examples in this section use the gaussdbjdbc.jar package by default.
- gscejdbc.jar: The main class name is com.huawei.gaussdb.jdbc.Driver. The URL prefix of the database connection is jdbc:gaussdb. This driver package contains the dependent libraries related to encryption and decryption that need to be loaded to the encrypted database. You are advised to use this driver package in encrypted scenarios. Currently, only EulerOS is supported.
- gaussdbjdbc-JRE7.jar: The main class name is com.huawei.gaussdb.jdbc.Driver. The URL prefix of the database connection is jdbc:gaussdb. The gaussdbjdbc-JRE7.jar package is used in the JDK 1.7 environment.
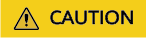
- Before using the gscejdbc.jar driver package, you need to set the environment variable LD_LIBRARY_PATH. For details, see section "Setting Encrypted Equality Queries > Using JDBC to Operate an Encrypted Database" in Feature Guide.
- In JDK 1.8, you are advised to use gaussdbjdbc.jar instead of gaussdbjdbc-JRE7.jar.
- For details about other JDBC JAR packages, see "Application Development Guide" > "JDBC Compatibility Package" in Developer Guide.
Driver Class
Before establishing a database connection, load the com.huawei.gaussdb.jdbc.Driver database driver class.
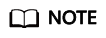
- GaussDB is compatible with PostgreSQL in the use of JDBC. Therefore, when two JDBC drivers are used in the same process, class names may conflict.
- GaussDB JDBC driver has the following enhanced features:
- The SHA256 encryption mode is supported for login.
- The third-party log framework that implements the sf4j API can be connected.
- DR failover is supported.
Environment Class
JDK1.8 must be configured on the client. JDK supports multiple platforms such as Windows and Linux. The following uses Windows as an example to describe how to configure JDK 1.8:
- Enter java -version in the MS-DOS window (command prompt in Windows) to check the JDK version. Ensure that the JDK version is JDK1.8. If JDK is not installed, download the installation package from the official website and install it.
- Configure system environment variables.
- Right-click My computer and choose Properties.
- In the navigation pane, choose Advanced system settings.
- In the System Properties dialog box, click Environment Variables on the Advanced tab page.
- In the System variables area of the Environment Variables dialog box, click New or Edit to configure system variables. For details about the variables, see Table 1.
Table 1 Variables Variable
Operation
Variable Value
JAVA_HOME
- If the variable exists, click Edit.
- If the variable does not exist, click New.
Specifies the Java installation directory.
Example: C:\Program Files\Java\jdk1.8.0_131.
Path
Click Edit.
- If JAVA_HOME is configured, add %JAVA_HOME%\bin before the variable value.
- If JAVA_HOME is not configured, add the following full Java installation path before the variable value:
C:\Program Files\Java\jdk1.8.0_131\bin
CLASSPATH
Click New.
%JAVA_HOME%\lib;%JAVA_HOME%\lib\tools.jar
Loading the Driver
Load the database driver before creating a database connection.
- Implicit loading at any position before a connection is created in the code: Class.forName("com.huawei.gaussdb.jdbc.Driver");
- Parameter transfer during JVM startup: java -Djdbc.drivers=com.huawei.gaussdb.jdbc.Driver jdbctest
jdbctest is the name of a test application.
Function Prototype
- DriverManager.getConnection(String url)
- DriverManager.getConnection(String url, Properties info)
- DriverManager.getConnection(String url, String user, String password)
Parameters
Parameter |
Description |
---|---|
url |
gaussdbjdbc.jar database connection descriptor. If host is set to a server name or an IPv4 address, formats are as follows:
If host is set to an IPv6 address, formats are as follows:
NOTE:
|
info |
For details about common attributes of info, see "Application Development Guide" > "Development Based on JDBC" > "Connecting to a Database" in Developer Guide. |
user |
Database user. |
password |
Password of the database user. |
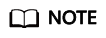
After the uppercaseAttributeName parameter is enabled, if the database contains metadata with a mixture of uppercase and lowercase letters, only the metadata in lowercase letters can be queried and output in uppercase letters. Before using the metadata, ensure that the metadata is stored in lowercase letters to prevent data errors.
Examples
Example 1: Connect to a database.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 |
// The following code encapsulates database connection operations into an interface. The database can then be connected using an authorized username and a password. public static Connection getConnect(String username, String passwd) { // Driver class. String driver = "com.huawei.gaussdb.jdbc.Driver"; // Database connection descriptor. String sourceURL = "jdbc:gaussdb://$ip:$port/database"; Connection conn = null; try { // Load the driver. Class.forName(driver); } catch( Exception e ) { e.printStackTrace(); return null; } try { // Create a connection. conn = DriverManager.getConnection(sourceURL, username, passwd); System.out.println("Connection succeed!"); } catch(Exception e) { e.printStackTrace(); return null; } return conn; } |
Example 2: Use the Properties object as a parameter to create a connection.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 |
// The following code uses the Properties object as a parameter to establish a connection: public static Connection getConnectUseProp(String username, String passwd) { // Driver class. String driver = "com.huawei.gaussdb.jdbc.Driver"; // Database connection descriptor. String sourceURL = "jdbc:gaussdb://$ip:$port/database?"; Connection conn = null; Properties info = new Properties(); try { // Load the driver. Class.forName(driver); } catch( Exception e ) { e.printStackTrace(); return null; } try { info.setProperty("user", username); info.setProperty("password", passwd); // Create a connection. conn = DriverManager.getConnection(sourceURL, info); System.out.println("Connection succeed!"); } catch(Exception e) { e.printStackTrace(); return null; } return conn; } |
For details about common parameters, see "Application Development Guide" > "Development Based on JDBC" > "Common JDBC Parameters" in Developer Guide.
Example 3: Use the streaming read function.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 |
// There will be security risks if the username and password used for authentication are directly written into code. It is recommended that the username and password be stored in the configuration file or environment variables (the password must be stored in ciphertext and decrypted when being used) to ensure security. // In this example, the username and password are stored in environment variables. Before running this example, set environment variables EXAMPLE_USERNAME_ENV and EXAMPLE_PASSWORD_ENV in the local environment (set the environment variable names based on the actual situation). // Establish a connection. public static Connection getConnection(String username, String passwd) { String driver = "com.huawei.gaussdb.jdbc.Driver"; String sourceURL = "jdbc:gaussdb://$ip:$port/database?enableStreamingQuery=true"; Connection conn = null; try { // Load the driver. Class.forName(driver); } catch (Exception e) { e.printStackTrace(); return null; } try { // Create a connection. conn = DriverManager.getConnection(sourceURL, username, passwd); System.out.println("Connection succeed!"); } catch (Exception e) { e.printStackTrace(); return null; } return conn; } // Execute common SQL statements to create table t_user. public static void CreateTable(Connection conn) { Statement stmt = null; try { stmt = conn.createStatement(); // Execute common SQL statements. stmt.executeUpdate("DROP TABLE IF EXISTS t_user"); stmt.executeUpdate("CREATE TABLE t_user(id int, name VARCHAR(20));"); stmt.close(); } catch (SQLException e) { if (stmt != null) { try { stmt.close(); } catch (SQLException e1) { e1.printStackTrace(); } } e.printStackTrace(); } } // Execute a prepared statement to insert data in batches. public static void BatchInsertData(Connection conn) { PreparedStatement pst = null; try { // Generate a prepared statement. pst = conn.prepareStatement("INSERT INTO t_user VALUES (?,?)"); for (int i = 0; i < 20; i++) { // Add parameters. pst.setInt(1, i + 1); pst.setString(2, "name " + (i + 1)); pst.addBatch(); } // Perform batch processing. pst.executeBatch(); pst.close(); } catch (SQLException e) { if (pst != null) { try { pst.close(); } catch (SQLException e1) { e1.printStackTrace(); } } e.printStackTrace(); } } // Enable streaming read and query the content in the t_user table. public static void StreamingQuery(Connection conn) { PreparedStatement pst = null; ResultSet resultSet = null; try { // Query all values in the t_user table. pst = conn.prepareStatement("SELECT * FROM t_user"); pst.setFetchSize(Integer.MIN_VALUE);// Functions the same as ((PgStatement)statement).enableStreamingResults();. resultSet = pst.executeQuery(); while (resultSet.next()) { System.out.println(resultSet.getInt(1)); } } catch (SQLException e) { throw new RuntimeException(e); } finally { if (resultSet != null) { try { resultSet.close(); } catch (SQLException e) { throw new RuntimeException(e); } } if (pst != null) { try { pst.close(); } catch (SQLException e) { throw new RuntimeException(e); } } } } public static void main(String[] args) throws Exception { String userName = System.getenv("EXAMPLE_USERNAME_ENV"); String password = System.getenv("EXAMPLE_PASSWORD_ENV"); Connection conn = getConnection(userName, password); CreateTable(conn); BatchInsertData(conn); StreamingQuery(conn); // Close the connection to the database. try { conn.close(); } catch (SQLException e) { e.printStackTrace(); } } |
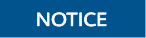
When the streaming read function is used, you need to perform the resultSet.close() or statement.close() operation after the result set is used. Otherwise, the current connection is unavailable.
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot