Connecting Spring Cloud Applications to CSE Engines
This section describes how to connect Spring Cloud applications to CSE engines and use the most common functions of CSE engines. For details about the development guide, see Using Microservice Engine Functions.
In the Spring Cloud Huawei Samples project, you can find the code corresponding to the development methods in this section.
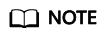
Spring Cloud needs to use Spring Cloud Huawei to connect to CSE engines. This document describes how to integrate and use Spring Cloud Huawei in Spring Cloud.
Prerequisites
- Microservice applications have been developed based on Spring Cloud.
For details about microservice application development in the Spring Cloud microservice framework, see https://spring.io/projects/spring-cloud.
- Version requirements. See Requirements for Microservice Development Framework of a.
- This document assumes that you use Maven for dependency management and packaging in your project. You are familiar with the Maven dependency management mechanism and are able to modify the dependency management and dependency in the pom.xml file.
Procedure
- Add dependencies to the pom.xml file of the project.
- If you develop microservices using Spring Cloud, introduce the following dependencies:
<dependency> <groupId>com.huaweicloud</groupId> <artifactId>spring-cloud-starter-huawei-service-engine</artifactId> </dependency>
The spring-cloud-starter-huawei-service-engine module consists of the following dependent modules:
<!-- Registry and discovery module --> <dependency> <groupId>com.huaweicloud</groupId> <artifactId>spring-cloud-starter-huawei-discovery</artifactId> </dependency> <!-- Configuration center module --> <dependency> <groupId>com.huaweicloud</groupId> <artifactId>spring-cloud-starter-huawei-config</artifactId> </dependency> <!-- Service governance module --> <dependency> <groupId>com.huaweicloud</groupId> <artifactId>spring-cloud-starter-huawei-governance</artifactId> </dependency> <!-- Dark launch module --> <dependency> <groupId>com.huaweicloud</groupId> <artifactId>spring-cloud-starter-huawei-router</artifactId> </dependency>
- If you develop the gateway using Spring Cloud, introduce the following dependencies:
<dependency> <groupId>com.huaweicloud</groupId> <artifactId>spring-cloud-starter-huawei-service-engine-gateway</artifactId> </dependency>
The spring-cloud-starter-huawei-service-engine-gateway module consists of the following dependent modules:
<!-- Registry and discovery module --> <dependency> <groupId>com.huaweicloud</groupId> <artifactId>spring-cloud-starter-huawei-discovery</artifactId> </dependency> <!-- Configuration center module --> <dependency> <groupId>com.huaweicloud</groupId> <artifactId>spring-cloud-starter-huawei-config</artifactId> </dependency> <!-- Service governance module --> <dependency> <groupId>com.huaweicloud</groupId> <artifactId>spring-cloud-starter-huawei-governance</artifactId> </dependency> <!-- Dark launch module --> <dependency> <groupId>com.huaweicloud</groupId> <artifactId>spring-cloud-starter-huawei-router</artifactId> </dependency> <!-- Gateway module --> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-gateway</artifactId> </dependency>
You are advised to use Maven Dependency Management to manage the third-party software dependencies of a project. Introduce the following dependencies to the project:
<dependencyManagement> <dependencies> <!-- configure user spring cloud / spring boot versions --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-dependencies</artifactId> <version>${spring-boot.version}</version> <type>pom</type> <scope>import</scope> </dependency> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-dependencies</artifactId> <version>${spring-cloud.version}</version> <type>pom</type> <scope>import</scope> </dependency> <!-- configure spring cloud huawei version --> <dependency> <groupId>com.huaweicloud</groupId> <artifactId>spring-cloud-huawei-bom</artifactId> <version>${spring-cloud-huawei.version}</version> <type>pom</type> <scope>import</scope> </dependency> </dependencies> </dependencyManagement>
Skip the operation if your project already contains the preceding dependencies.
If other registry and discovery libraries, such as Eureka, are used in your project, you need to adjust the project as follows:
- Delete the dependencies related to Eureka from the project. For example:
<dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-netflix-eureka-server</artifactId> </dependency>
- If @EnableEurekaServer is used in the code, delete it and replace it with @EnableDiscoveryClient.
The spring-cloud-starter-huawei-service-engine component provides functions such as service registration, configuration center, service governance, dark launch, and contract management. Contract management is not mandatory for the running of Spring Cloud microservice applications. The microservice engine limits the number of contracts. When the number of microservice application contracts exceeds the limit, the registry fails. If the legacy system cannot be properly split to reduce the number of contracts, the dependency can be excluded and the contract management function is not used.
<dependency> <groupId>com.huaweicloud</groupId> <artifactId>spring-cloud-starter-huawei-service-engine</artifactId> <exclusions> <exclusion> <groupId>com.huaweicloud</groupId> <artifactId>spring-cloud-starter-huawei-swagger</artifactId> </exclusion> </exclusions> </dependency>
- If you develop microservices using Spring Cloud, introduce the following dependencies:
- Configure microservice information.
Add the microservice description to the bootstrap.yml file. If the bootstrap.yml file is not available in the project, create one.
spring: application: name: basic-provider cloud: servicecomb: discovery: enabled: true address: http://127.0.0.1:30100 appName: basic-application serviceName: ${spring.application.name} version: 0.0.1 healthCheckInterval: 15 config: serverAddr: http://127.0.0.1:{port} serverType: {servertype}
- healthCheckInterval is in seconds.
- For microservice engine 1.x, {port} is 30103 and {servertype} is config-center.
- For microservice engine 2.x, {port} is 30110 and {servertype} is kie (recommended) or config-center.
- (Optional) Configure the AK/SK.
Perform this step only when you use the professional microservice engine.
The AK/SK is configured in the bootstrap.yml file. The plaintext configuration is provided by default. You can also customize the encryption storage scheme. For details about how to obtain the AK/SK and project name, see Obtaining the AK/SK and Project Name.
- Add the following configuration in plaintext to the bootstrap.yml file:
spring: cloud: servicecomb: credentials: enabled: true accessKey: AK #Enter the AK. secretKey: SK #Enter the SK. akskCustomCipher: default project: Project name #Enter the project name.
- Custom implementation
- Implement the com.huaweicloud.common.util.Cipher API using either of the following methods:
- String name(), which is the name definition of spring.cloud.servicecomb.credentials.akskCustomCipher and needs to be added to the configuration file.
- char[] decode(char[] encrypted), which is the decryption API used to decrypt secretKey.
public class CustomCipher implements Cipher
To implement encryption and decryption, you need to use BootstrapConfiguration as the startup add-in. Add the following statement first:
@Configuration public class MyAkSKCipherConfiguration { @Bean public Cipher customCipher() { return new CustomCipher(); } }
Add the META-INF/spring.factories file to define the configuration:
org.springframework.cloud.bootstrap.BootstrapConfiguration=\ com.huaweicloud.common.transport.MyAkSKCipherConfiguration
- After the custom configuration is complete, you can use the new decryption algorithm in the bootstrap.yaml file.
spring: cloud: servicecomb: credentials: enabled: true accessKey: AK #Enter the AK. secretKey: SK #Enter the encrypted SK. akskCustomCipher: youciphername #Enter the returned name of the name() method in the implementation class. project: Project name #Enter the project name.
If you do not want to write the AK/SK into the configuration file, you can configure the AK/SK by adding environment variables for the microservice. For details, see Method 2.
- Implement the com.huaweicloud.common.util.Cipher API using either of the following methods:
- Add the following configuration in plaintext to the bootstrap.yml file:
- (Optional) Configure security authentication parameters.
Perform this step only when you use the exclusive microservice engine and enable security authentication. In other scenarios, skip this step.
After security authentication is enabled for a microservice engine, all called APIs can be called only after a token is obtained. For details about the authentication process, see RBAC.
To use security authentication, obtain the username and password from the microservice engine and then add the following configuration to the configuration file. The password is stored in plaintext by default. You can customize the encryption algorithm for the password.
- Configuration in plaintext
spring: cloud: servicecomb: credentials: account: name: username password: password cipher: default
- Custom encryption algorithms for storage
Implement the com.huaweicloud.common.util.Cipher API using either of the following methods: String name(), which is the name definition of spring.cloud.servicecomb.credentials.cipher and needs to be added to the configuration file. char[] decode(char[] encrypted), which is the decryption API used to decrypt secretKey. public class CustomCipher implements Cipher To implement encryption and decryption, you need to use BootstrapConfiguration as the startup add-in. Add the following statement first: @Configuration public class MyCipherConfiguration { @Bean public Cipher customCipher() { return new CustomCipher(); } } Add the META-INF/spring.factories file to define the configuration: org.springframework.cloud.bootstrap.BootstrapConfiguration=\ com.huaweicloud.common.transport.MyCipherConfiguration After the custom configuration is complete, you can use the new decryption algorithm in the bootstrap.yaml file.
spring: cloud: servicecomb: credentials: account: name: username password: password cipher: user-defined algorithm name
The RBAC function requires 1.6.0-Hoxton or later.
If you use the professional microservice engine, the watch function is not available. You need to disable the watch function in the configuration file. Otherwise, error logs will be periodically printed. Set watch to false for the service center. The watch function is disabled by default in 1.6.0-Hoxton and later. Therefore, you do not need to set the watch function.
spring: cloud: servicecomb: discovery: watch: false
- Configuration in plaintext
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot