Introduction to App ID Authentication
Authentication using app IDs is supported to make integrating Huawei Cloud Meeting easier and more secure for third-party applications. An app ID identifies an application. An app ID can be used on a third-party desktop terminal, mobile terminal, and web application at the same time.
How App ID Authentication Works
- App ID authentication on a third-party client
Figure 1 App ID authentication process on a third-party client
Prerequisites:
- You have requested and obtained an app ID and appKey on the Huawei Cloud Meeting console.
- You have integrated the signature generation algorithm on your own server. For details, see Signature Generation Algorithms for Third-Party Service Integration.
- The app ID is passed during SDK initialization. For details, see section "Initialization" in Client SDK Reference.
Process:
- The third-party client sends the third-party user ID to the third-party server.
- The third-party server generates a signature for authentication based on the app ID, user ID, and application key.
- The third-party server returns the value of Signature, ExpireTime, and Nonce.
- The third-party client calls the login API of the client SDK. The parameters include User ID, Signature, ExpireTime, and Nonce.
- The client SDK sends an authentication request to the Huawei Cloud Meeting server.
- After the authentication, the SDK obtains the access token. The token is inaccessible to the third-party client and is maintained and updated by the SDK.
- App ID authentication on a third-party server
Figure 2 App ID authentication process on a third-party server
Prerequisites:
- You have requested and obtained an app ID and appKey on the Huawei Cloud Meeting console.
- You have integrated the signature generation algorithm on your own server. For details, see Signature Generation Algorithms for Third-Party Service Integration.
Process:
- The third-party server uses the third-party user ID, app ID, appKey, ExpireTime, and Nonce required in API calling to generate a signature for authentication.
- The third-party server calls the app ID authentication API (a REST API) of the Huawei Cloud Meeting server.
- After the authentication is successful, a token is returned.
- The third-party server uses the access token to call other service APIs.
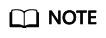
1. The validity period of an access token is 12 to 24 hours.
2. The User ID parameter is optional when you are generating a signature or calling the appAuth API. If the User ID parameter is not specified, the creator of the enterprise, namely, the enterprise owner is used by default.
3. The third-party application must ensure each user ID is unique in the enterprise. If the third party is a service provider and provides an application to multiple enterprises, both User ID and Corp ID are mandatory when you call the APIs.
4. If you want to use the Huawei Cloud Meeting contacts, information, such as email address, name, and phone number, can be carried during login authentication. The information will be written into Huawei Cloud Meeting contacts.
Requesting an App ID
- Use your Huawei Cloud account to log in to the Huawei Cloud Meeting console. Ensure that you have subscribed to Huawei Cloud Meeting using the Huawei Cloud account or have bound the Huawei Cloud account to a Huawei Cloud Meeting enterprise administrator account. For details, see Preparations in Development Process.
- In the navigation pane, choose Applications and click Create Application. In the displayed dialog box, enter the name and description of the third-party application.
Figure 3 Creating an application
- Click Create.
An app ID and appKey are generated.
Figure 4 Application created
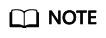
- An appKey is the key used to generate the authentication signature. The key must be properly kept on the third-party server. Otherwise, meeting resources may be stolen.
- appKeys must be stored on third-party servers rather than third-party clients. appKeys stored on clients can be easily obtained through decompilation.
- appKeys can only be reset. There is no way to retrieve lost keys. When a key is lost, generate a new one. The original key will expire in one month.
Signature Generation Algorithms for Third-Party Service Integration
If an application is used only in a single enterprise, the algorithm for generating an authentication signature is as follows:
Signature = HexEncode(HMAC-SHA256((App ID + ":" + User ID + ":" + ExpireTime + ":" + Nonce), appKey))
If an application is developed by a service provider and used in multiple enterprises, the algorithms for generating an authentication signature in different scenarios are as follows:
- When enterprise users manage their meetings:
Signature = HexEncode(HMAC-SHA256((App ID + ":" + CorpID + ":" + User ID + ":" + ExpireTime + ":" + Nonce), appKey))
- When enterprise administrators manage enterprise resources:
Signature = HexEncode(HMAC-SHA256((App ID + ":" + CorpID + "::" + ExpireTime + ":" + Nonce), appKey))
An enterprise administrator can also have a user ID. The user ID must have the administrator permissions.
- When service provider administrators manage service provider resources (for example, creating enterprises or allocating resources to enterprises):
Signature = HexEncode(HMAC-SHA256((App ID + ":::" + ExpireTime + ":" + Nonce), appKey))
In the preceding algorithms:
- The input data of HMAC-SHA256 is the values of App ID, CorpID (optional), User ID (optional), ExpireTime, and Nonce. The values must be separated by colons (:). For example, d5e17******************489e:alice@ent01:1604020600:EycLQs************************nINuU1EBpQ
In SP mode, the colons (:) cannot be omitted even if User ID and Corp ID are left empty.
- The key of HMAC-SHA256 is the value of appKey. For example, tZAe********q32T.
- The binary number generated by HMAC-SHA256 needs to be converted into a hexadecimal string (HexEncode). The signature generated by the preceding data and key is 2a8c780c***************************************************5a6c44f3c1b3c2455d.
- ExpireTime: expiration timestamp of the authentication signature. The unit is second. For example, if the current system timestamp is 1604020000 and you want to set the validity period of the signature to 10 minutes, the value of ExpireTime is 1604020600 (1604020000 + 10 x 60).
- Nonce: a random character string, which must be different each time when the authentication signature is calculated. The string has 32 to 64 characters.
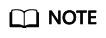
1. Because the authentication signature has a validity period, the difference between the system time of the third-party server and that of the Huawei Cloud Meeting server cannot be too large and must be smaller than the validity period specified by ExpireTime, for example, 10 minutes in the preceding example. The time of the Huawei Cloud Meeting server is synchronized with the standard NTP time.
2. When ExpireTime is set to 0, the signature does not expire. To prevent replay attacks, do not set ExpireTime to 0.
Source code of the signature generation algorithm in Java:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 |
import java.io.UnsupportedEncodingException; import java.security.InvalidKeyException; import java.security.NoSuchAlgorithmException; import javax.crypto.Mac; import javax.crypto.spec.SecretKeySpec; public class HmacSHA256 { // Hexadecimal character set private final static char[] DIGEST_ARRAYS = { '0', '1', '2', '3', '4', '5', '6', '7', '8', '9', 'a', 'b', 'c', 'd', 'e', 'f' }; /** * Function: signature generation algorithm * Input parameters: * 1. data: HMAC-SHA256 input data * 2. key: appKey * Output parameter: a hexadecimal character string generated using the value of HMAC-SHA256 */ public static String encode(String data, String key) { byte[] hashByte; try { Mac sha256HMAC = Mac.getInstance("HmacSHA256"); SecretKeySpec secretKey = new SecretKeySpec(key.getBytes("UTF-8"), "HmacSHA256"); sha256HMAC.init(secretKey); hashByte = sha256HMAC.doFinal(data.getBytes("UTF-8")); } catch (NoSuchAlgorithmException | UnsupportedEncodingException | InvalidKeyException e) { return null; } return bytesToHex(hashByte); } /** * Function: converting an array in bytes into a hexadecimal string * Input parameter: * 1. bytes: byte array to be converted * Output parameter: hexadecimal character string */ private static String bytesToHex(byte[] bytes) { StringBuffer hexStr = new StringBuffer(); for (int i = 0; i < bytes.length; i++) { hexStr.append(DIGEST_ARRAYS[bytes[i] >>> 4 & 0X0F]); hexStr.append(DIGEST_ARRAYS[bytes[i] & 0X0F]); } return hexStr.toString(); } } |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
import hmac from hashlib import sha256 class HmacSHA256: def __init__(self, sig_data, sig_key): self.data = sig_data self.key = sig_key def encode(self): try: sig_data = self.data.encode('utf-8') secret_key = self.key.encode('utf-8') signature = hmac.new(secret_key, sig_data, digestmod=sha256).hexdigest() except Exception as e: print (e) raise e return signature |
Source code of the signature generation algorithm in C++:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 |
#include <openssl/hmac.h> #include <string.h> #include <iostream> using namespace std; const int HMAC_ENCODE_SUCCESS = 0; const int HMAC_ENCODE_FAIL = -1; const int HMAC_SHA256_STR_LEN = 65; /** * Function: converting a byte stream into a hexadecimal character string * Input parameters: * 1. input: byte stream pointer * 2. len: number of bytes in a byte stream * Output parameters: * 3. output: output buffer * 6. output_length: length of the signature character string * Returned value: * 0: failed * Integer greater than 0: length of the character string after conversion */ int Byte2HexStr(char * output, unsigned char * input, unsigned int len) { if ((NULL == output) || (NULL == input)) { return 0; } unsigned int i = 0; for (i = 0; i < len; i++) { sprintf_s(output + 2*i, HMAC_SHA256_STR_LEN-2*i, "%x%x", (input[i] >> 4) & 0x0F, input[i]&0x0F); } *(output + 2*i) = '\0'; return 2*i; } /** * Function: signature generation algorithm * Input parameters: * 1. key: appKey * 2. key_length: length of the appKey * 3. input: HMAC-SHA256 input data * 4. input_length: length of the character string in the HMAC-SHA256 input data * Output parameters: * 5. output: output buffer * 6. output_length: length of the signature character string * Returned value: * 0: successful * -1: failed */ int HmacEncode(const char * key, unsigned int key_length, const char * input, unsigned int input_length, char output[HMAC_SHA256_STR_LEN], unsigned int &output_length) { // Calculate the byte stream of HMAC_SHA256. const EVP_MD * engine = EVP_sha256(); unsigned char * byte_output = (unsigned char*)malloc(EVP_MAX_MD_SIZE); if (NULL == byte_output) { output_length = 0; return HMAC_ENCODE_FAIL; } unsigned int byte_output_length = 0; HMAC_CTX *ctx; ctx = HMAC_CTX_new(); HMAC_Init(ctx, key, strlen(key), engine); HMAC_Update(ctx, (unsigned char*)input, strlen(input)); HMAC_Final(ctx, byte_output, &byte_output_length); HMAC_CTX_free(ctx); // Convert the HMAC_SHA256 byte stream into a hexadecimal character string. int ret = Byte2HexStr(output, byte_output, byte_output_length); free(byte_output); if (0 == ret) { output_length = 0; return HMAC_ENCODE_FAIL; } else { output_length = ret; return HMAC_ENCODE_SUCCESS; } } |
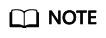
The signature generation algorithm in C++ is based on OpenSSL 1.1.0 or later. Compile and install the OpenSSL library first. For details, see OpenSSL.
Source code of the signature generation algorithm in JavaScript:
1 2 3 4 5 6 7 8 9 10 11 |
<script src="https://cdnjs.cloudflare.com/ajax/libs/crypto-js/3.1.9-1/crypto-js.js"> </script> <script> /** * 1. Do not include the signature generation algorithm in the frontend code. Otherwise, the appKey may be leaked, causing enterprise resource theft. * 2. The sample code is used only for debugging. */ function genSignature(data,appKey){ var sign = CryptoJS.HmacSHA256(data,appKey).toString(); return sign } </script> |
App ID Authentication on Third-Party Clients Integrated with the UI SDK
When a third-party client initializes the SDK, App ID needs to be passed.
- For details about the initialization API of the Android SDK, see Android SDK Initialization in the Client SDK Reference.
- For details about the initialization API of the iOS SDK, see iOS SDK Initialization in Client SDK Reference.
- For details about the initialization API of the Windows SDK, see Windows SDK Initialization in Client SDK Reference.
- For details about the initialization API of the macOS SDK, see macOS SDK Initialization in Client SDK Reference.
When a third-party client logs in, obtain Signature, ExpireTime, and Nonce from the third-party server and call the login API of the SDK to complete authentication.
- For details about the login API of the Android SDK, see Android SDK Login in Client SDK Reference.
- For details about the login API of the iOS SDK, see iOS SDK Login in Client SDK Reference.
- For details about the login API of the Windows SDK, see Windows SDK Login in Client SDK Reference.
- For details about the login API of the macOS SDK, see macOS SDK Login in Client SDK Reference.
App ID Authentication on Third-Party Servers
Third-party servers authenticate app IDs through the app ID authentication API and obtain the access token. For details, see section Authenticating an App ID in Server API Reference.
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot