type Tx
The following table describes type Tx.
Method |
Description |
Return Value |
(tx *Tx)Commit() |
Commits a transaction. |
error |
(tx *Tx)Exec(query string, args ...interface{}) |
Performs an operation that does not return rows of data. |
Result and error |
(tx *Tx)ExecContext(ctx context.Context, query string, args ...interface{}) |
Performs an operation that does not return rows of data in a specified context. |
Result and error |
(tx *Tx)Prepare(query string) |
Creates a prepared statement for subsequent queries or executions. The returned statement is executed within a transaction and cannot be used when the transaction is committed or rolled back. |
*Stmt and error |
(tx *Tx)PrepareContext(ctx context.Context, query string) |
Creates a prepared statement for subsequent queries or executions. The returned statement is executed within a transaction and cannot be used when the transaction is committed or rolled back. The specified context will be used in the preparation phase, not in the transaction execution phase. The statement returned by this method will be executed in the transaction context. |
*Stmt and error |
(tx *Tx)Query(query string, args ...interface{}) |
Executes a query that returns rows of data. |
*Rows and error |
(tx *Tx)QueryContext(ctx context.Context, query string, args ...interface{}) |
Executes a query that returns rows of data in a specified context. |
*Rows and error |
(tx *Tx)QueryRow(query string, args ...interface{}) |
Executes a query that returns only one row of data. |
*Row |
(tx *Tx)QueryRowContext(ctx context.Context, query string, args ...interface{}) |
Executes a query that returns only one row of data in a specified context. |
*Row |
(tx *Tx) Rollback() |
Rolls back a transaction. |
error |
(tx *Tx)Stmt(stmt *Stmt) |
Returns a transaction-specific prepared statement for an existing statement. Example: str, err := db.Prepare("insert into t1 values(:1, :2)") tx, err := db.Begin() res, err := tx.Stmt(str).Exec(1, "aaa") |
*Stmt |
(tx *Tx)StmtContext(ctx context.Context, stmt *Stmt) |
Returns a transaction-specific prepared statement for an existing statement in a specified context. |
*Stmt |
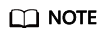
- The Query(), QueryContext(), QueryRow(), and QueryRowContext() APIs are usually used in query statements, such as SELECT. The Exec() API is used for executing operation statements. If query APIs are used to execute non-query statements, the execution result may be unexpected. Therefore, you are advised not to use the query APIs to execute non-query statements, such as UPDATE and INSERT.
- The result of executing a query statement using a query API needs to be obtained through the Next() API in type Rows. If the result is not obtained through the Next() API, unexpected errors may occur.
Parameters
Parameter |
Description |
ctx |
Specified context. |
query |
Executed SQL statement. |
args |
Parameter that needs to be bound to the executed SQL statement. Binding by location and binding by name are supported. For details, see Examples in section "type DB." |
stmt |
Existing prepared statement, which is generally the prepared statement returned by the PREPARE statement |
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot