type DB
The following table describes type DB.
Method |
Description |
Return Value |
(db *DB)Begin() |
Starts a transaction. The isolation level of the transaction is determined by the driver. |
*Tx and error |
(db *DB)BeginTx(ctx context.Context, opts *TxOptions) |
Starts a transaction with a specified transaction isolation level. A specified context is used until the transaction is committed or rolled back. If the context is canceled, the SQL package rolls back the transaction. |
*Tx and error |
(db *DB)Close() |
Closes the database and releases all the opened resources. |
error |
(db *DB)Exec(query string, args ...interface{}) |
Performs an operation that does not return rows of data. |
Result and error |
(db *DB)ExecContext(ctx context.Context, query string, args ...interface{}) |
Performs an operation that does not return rows of data in a specified context. |
Result and error |
(db *DB)Ping() |
Checks whether the database connection is still valid and establishes a connection if necessary. |
error |
(db *DB)PingContext(ctx context.Context) |
Checks whether the database connection is still valid in a specified context and establishes a connection if necessary. |
error |
(db *DB)Prepare(query string) |
Creates a prepared statement for subsequent queries or executions. |
*Stmt and error |
(db *DB)PrepareContext(ctx context.Context, query string) |
Creates a prepared statement for subsequent queries or executions in a specified context. |
*Stmt and error |
(db *DB)Query(query string, args ...interface{}) |
Executes a query and returns multiple rows of data. |
*Rows and error |
(db *DB)QueryContext(ctx context.Context, query string, args ...interface{}) |
Executes a query and returns multiple rows of data in a specified context. |
*Rows and error |
(db *DB)QueryRow(query string, args ...interface{}) |
Executes a query that returns only one row of data. |
*Row |
(db *DB)QueryRowContext(ctx context.Context, query string, args ...interface{}) |
Executes a query that returns only one row of data in a specified context. |
*Row |
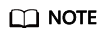
- The Query(), QueryContext(), QueryRow(), and QueryRowContext() APIs are usually used in query statements, such as SELECT. The Exec() API is used for executing operation statements. If query APIs are used to execute non-query statements, the execution result may be unexpected. Therefore, you are advised not to use the query APIs to execute non-query statements, such as UPDATE and INSERT.
- The result of executing a query statement using a query API needs to be obtained through the Next() API in type Rows. If the result is not obtained through the Next() API, unexpected errors may occur.
Parameters
Parameter |
Description |
ctx |
Specified context. |
query |
Executed SQL statement. |
args |
Parameter that needs to be bound to the executed SQL statement. Binding by location and binding by name are supported. For details, see Examples. |
opts |
Transaction isolation level and transaction access mode. The transaction isolation level (opts.Isolation) supports sql.LevelReadUncommitted, sql.LevelReadCommitted, sql.LevelRepeatableRead, and sql.LevelSerializable. The transaction access mode (opts.ReadOnly) can be true (read only) or false (read write). |
Examples
// In this example, the username and password are stored in environment variables. Before running this example, set environment variables in the local environment (set the environment variable names based on the actual situation). func main() { hostip := os.Getenv("GOHOSTIP") // GOHOSTIP indicates the IP address written into the environment variable. port := os.Getenv("GOPORT") // GOPORT indicates the port number written into the environment variable. usrname := os.Getenv("GOUSRNAME") // GOUSRNAME indicates the username written into the environment variable. passwd := os.Getenv("GOPASSWD") // GOPASSWDW indicates the user password written into the environment variable. str := "host=" + hostip + " port=" + port + " user=" + usrname + " password=" + passwd + " dbname=postgres sslmode=disable" db, err:= sql.Open("opengauss", str) if err != nil { log.Fatal(err) } defer db.Close() err = db.Ping() if err != nil { log.Fatal(err) } _, err = db.Exec("drop table if exists testuser.test") _, err = db.Exec("create table test(id int, name char(10))") // Binding by location _, err = db.Exec("insert into test(id, name) values(:1, :2)", 1, "Zhang San") if err != nil { log.Fatal(err) } // Binding by name _, err = db.Exec("insert into test(id, name) values(:id, :name)", sql.Named("id", 1), sql.Named("name", "Zhang San")) if err != nil { log.Fatal(err) } }
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot