Clients in Python
Access a DCS Redis instance through redis-py on an ECS in the same VPC. For more information about how to use other Redis clients, visit the Redis official website.
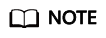
Use redis-py to connect to single-node, master/standby, and Proxy Cluster instances and redis-py-cluster to connect to Redis Cluster instances.
Prerequisites
- A DCS Redis instance has been created and is in the Running state.
- An ECS has been created. For details about how to create an ECS, see Purchasing an ECS.
- If the ECS runs the Linux OS, ensure that the Python compilation environment has been installed on the ECS.
Procedure
- View the IP address/domain name and port number of the DCS Redis instance to be accessed.
For details, see Viewing Instance Details.
- Log in to the ECS.
The following uses CentOS as an example to describe how to access an instance using a Python client.
- Access the DCS Redis instance.
If the system does not provide Python, run the following yum command to install it:
yum install pythonThe Python version must be 3.6 or later. If the default Python version is earlier than 3.6, perform the following operations to change it:
- Run the rm -rf python command to delete the Python symbolic link.
- Run the ln -s pythonX.X.X python command to create another Python link. In the command, X.X.X indicates the Python version number.
- If the instance is a single-node, master/standby, or Proxy Cluster instance:
- Install Python and redis-py.
- If the system does not provide Python, run the yum command to install it.
- Run the following command to download and decompress the redis-py package:
wget https://github.com/andymccurdy/redis-py/archive/master.zip
unzip master.zip
- Go to the directory where the decompressed redis-py package is saved, and install redis-py.
After the installation, run the python command. redis-py have been successfully installed if the following command output is displayed:
Figure 1 Running the python command
- Use the redis-py client to connect to the instance. In the following steps, commands are executed in CLI mode. (Alternatively, write the commands into a Python script and then execute the script.)
- Run the python command to enter the CLI mode. You have entered CLI mode if the following command output is displayed:
Figure 2 Entering the CLI mode
- Run the following command to access the chosen DCS Redis instance:
r = redis.StrictRedis(host='XXX.XXX.XXX.XXX', port=6379, password='******');
XXX.XXX.XXX.XXX indicates the IP address/domain name of the DCS instance and 6379 is an example port number of the instance. For details about how to obtain the IP address/domain name and port, see 1. Change them as required. ****** indicates the password used for logging in to the chosen DCS Redis instance. This password is defined during DCS Redis instance creation.
You have successfully accessed the instance if the following command output is displayed. Enter commands to perform read and write operations on the database.
Figure 3 Redis connected successfully
- Run the python command to enter the CLI mode. You have entered CLI mode if the following command output is displayed:
- Install Python and redis-py.
- If the instance is a Redis Cluster instance:
- Install the redis-py-cluster client.
- Access the DCS Redis instance by using redis-py-cluster.
In the following steps, commands are executed in CLI mode. (Alternatively, write the commands into a Python script and then execute the script.)
- Run the python command to enter the CLI mode.
- Run the following command to access the chosen DCS Redis instance. If the instance does not have a password, exclude password='******' from the command.
>>> from rediscluster import RedisCluster >>> startup_nodes = [{"host": "192.168.0.143", "port": "6379"},{"host": "192.168.0.144", "port": "6379"},{"host": "192.168.0.145", "port": "6379"},{"host": "192.168.0.146", "port": "6379"}] >>> rc = RedisCluster(startup_nodes=startup_nodes, decode_responses=True, password='******') >>> rc.set("foo", "bar") True >>> print(rc.get("foo")) 'bar'
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.