公共要求
注:使用前请务必先仔细阅读使用注意事项。
样例 |
|
---|---|
环境要求 |
JDK 1.6及以上版本。 |
引用库 |
httpclient、httpcore、httpmime、commons-codec、commons-logging、jackson-databind、jackson-annotations、jackson-core、fastjson、log4j |
将下列代码样例复制到新建java文件中即可运行。
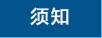
- 本文档所述Demo在提供服务的过程中,可能会涉及个人数据的使用,建议您遵从国家的相关法律采取足够的措施,以确保用户的个人数据受到充分的保护。
- 本文档所述Demo仅用于功能演示,不允许客户直接进行商业使用。
- 本文档信息仅供参考,不构成任何要约或承诺。
公共依赖
使用Java样例时请参考以下工程目录结构:
Constant.java
/* * File Name: com.huawei.utils.Constant.java * * Copyright Notice: * Copyright 1998-2008, Huawei Technologies Co., Ltd. ALL Rights Reserved. * * Warning: This computer software sourcecode is protected by copyright law * and international treaties. Unauthorized reproduction or distribution * of this sourcecode, or any portion of it, may result in severe civil and * criminal penalties, and will be prosecuted to the maximum extent * possible under the law. */ package com.huawei.utils; public class Constant { // purchase and get : base_url,appid,secret // please replace the IP and Port, when you use the demo. public static final String BASE_URL = "https://{domain}:{port}"; // please replace the appId and secret, when you use the demo. public static final String CLICK2CALL_APPID = "*****"; public static final String CLICK2CALL_SECRET = "*****"; public static final String CALLNOTIFY_APPID = "*****"; public static final String CALLNOTIFY_SECRET = "*****"; public static final String CALLVERIFY_APPID = "*****"; public static final String CALLVERIFY_SECRET = "*****"; // *************************** The following constants do not need to be // modified *********************************// /* * request header * 1. HEADER_APP_AUTH * 2. HEADER_APP_AKSK * 3. AUTH_HEADER_VALUE * 4. AKSK_HEADER_FORMAT */ public static final String HEADER_APP_AUTH = "Authorization"; public static final String HEADER_APP_AKSK = "X-AKSK"; public static final String AUTH_HEADER_VALUE = "AKSK realm=\"SDP\",profile=\"UsernameToken\",type=\"Appkey\""; public static final String AKSK_HEADER_FORMAT = "UsernameToken Username=\"%s\",PasswordDigest=\"%s\",Nonce=\"%s\",Created=\"%s\""; /* * Voice Call: * 1. VOICE_CALL_COMERCIAL * 2. VOICE_VERIFICATION_COMERCIAL * 3. CALL_NOTIFY_COMERCIAL * 4. VOICE_FILE_DOWNLOAD */ public static final String VOICE_CALL_COMERCIAL = BASE_URL + "/rest/httpsessions/click2Call/v2.0"; public static final String VOICE_VERIFICATION_COMERCIAL = BASE_URL + "/rest/httpsessions/callVerify/v1.0"; public static final String CALL_NOTIFY_COMERCIAL = BASE_URL + "/rest/httpsessions/callnotify/v2.0"; public static final String VOICE_FILE_DOWNLOAD = BASE_URL + "/rest/provision/voice/record/v1.0"; }
HttpsUtil.java
package com.huawei.utils; import org.apache.http.HttpResponse; import org.apache.http.NameValuePair; import org.apache.http.client.config.RequestConfig; import org.apache.http.client.methods.HttpGet; import org.apache.http.client.methods.HttpPost; import org.apache.http.client.methods.HttpUriRequest; import org.apache.http.client.utils.URIBuilder; import org.apache.http.conn.ssl.SSLConnectionSocketFactory; import org.apache.http.conn.ssl.SSLContextBuilder; import org.apache.http.conn.ssl.TrustStrategy; import org.apache.http.entity.ContentType; import org.apache.http.entity.StringEntity; import org.apache.http.impl.client.CloseableHttpClient; import org.apache.http.impl.client.DefaultHttpClient; import org.apache.http.impl.client.HttpClients; import java.io.IOException; import java.net.URISyntaxException; import java.security.KeyManagementException; import java.security.KeyStoreException; import java.security.NoSuchAlgorithmException; import java.security.cert.CertificateException; import java.security.cert.X509Certificate; import java.util.List; import java.util.Map; import javax.net.ssl.HostnameVerifier; import javax.net.ssl.HttpsURLConnection; import javax.net.ssl.SSLContext; import javax.net.ssl.SSLSession; import javax.net.ssl.TrustManager; import javax.net.ssl.X509TrustManager; @SuppressWarnings("deprecation") public class HttpsUtil extends DefaultHttpClient { public final static String CONTENT_LENGTH = "Content-Length"; private static CloseableHttpClient httpClient = getHttpClient(); public static CloseableHttpClient getHttpClient() { SSLContext sslcontext = null; try { sslcontext = new SSLContextBuilder().loadTrustMaterial(null, new TrustStrategy() { @Override public boolean isTrusted(X509Certificate[] arg0, String arg1) throws CertificateException { return true; } }).build(); } catch (KeyManagementException e) { return null; } catch (NoSuchAlgorithmException e) { return null; } catch (KeyStoreException e) { return null; } // Allow TLSv1, TLSv1.1, TLSv1.2 protocol only SSLConnectionSocketFactory sslsf = new SSLConnectionSocketFactory(sslcontext, new String[] {"TLSv1", "TLSv1.1", "TLSv1.2"}, null, SSLConnectionSocketFactory.ALLOW_ALL_HOSTNAME_VERIFIER); CloseableHttpClient httpclient = HttpClients.custom() .setDefaultRequestConfig(RequestConfig.custom().setRedirectsEnabled(false).build()) .setSSLSocketFactory(sslsf).build(); return httpclient; } public HostnameVerifier hv = new HostnameVerifier() { public boolean verify(String urlHostName, SSLSession session) { return true; } }; public void trustAllHttpsCertificates() throws Exception { TrustManager[] trustAllCerts = new TrustManager[1]; TrustManager tm = new miTM(); trustAllCerts[0] = tm; SSLContext sc = SSLContext.getInstance("SSL"); sc.init(null, trustAllCerts, null); HttpsURLConnection.setDefaultSSLSocketFactory(sc.getSocketFactory()); } static class miTM implements TrustManager, X509TrustManager { public java.security.cert.X509Certificate[] getAcceptedIssuers() { return null; } public boolean isServerTrusted(X509Certificate[] certs) { return true; } public boolean isClientTrusted(X509Certificate[] certs) { return true; } public void checkServerTrusted(java.security.cert.X509Certificate[] certs, String authType) throws java.security.cert.CertificateException { return; } public void checkClientTrusted(java.security.cert.X509Certificate[] certs, String authType) throws java.security.cert.CertificateException { return; } } public StreamClosedHttpResponse doPostJsonGetStatusLine(String url, Map<String, String> headerMap, String content) { HttpPost request = new HttpPost(url); addRequestHeader(request, headerMap); request.setEntity(new StringEntity(content, ContentType.APPLICATION_JSON)); HttpResponse response = executeHttpRequest(request); if (null == response) { System.out.println("The response body is null."); } return (StreamClosedHttpResponse) response; } public HttpResponse doGetWithParas(String url, List<NameValuePair> queryParams, Map<String, String> headerMap) throws Exception { HttpGet request = new HttpGet(); addRequestHeader(request, headerMap); URIBuilder builder; try { builder = new URIBuilder(url); } catch (URISyntaxException e) { System.out.printf("URISyntaxException: {}", e); throw new Exception(e); } if (queryParams != null && !queryParams.isEmpty()) { builder.setParameters(queryParams); } request.setURI(builder.build()); return executeHttpRequest(request); } public StreamClosedHttpResponse doGetWithParasGetStatusLine(String url, List<NameValuePair> queryParams, Map<String, String> headerMap) throws Exception { HttpResponse response = doGetWithParas(url, queryParams, headerMap); if (null == response) { System.out.println("The response body is null."); } return (StreamClosedHttpResponse) response; } private static void addRequestHeader(HttpUriRequest request, Map<String, String> headerMap) { if (headerMap == null) { return; } for (String headerName : headerMap.keySet()) { if (CONTENT_LENGTH.equalsIgnoreCase(headerName)) { continue; } String headerValue = headerMap.get(headerName); request.addHeader(headerName, headerValue); } } private HttpResponse executeHttpRequest(HttpUriRequest request) { HttpResponse response = null; try { response = httpClient.execute(request); } catch (Exception e) { System.out.println("executeHttpRequest failed."); System.out.println(e.getStackTrace()); } finally { try { response = new StreamClosedHttpResponse(response); } catch (IOException e) { System.out.println("IOException: " + e.getMessage()); } } return response; } }
JsonUtil.java
/* * Copyright Notice: * Copyright 1998-2008, Huawei Technologies Co., Ltd. ALL Rights Reserved. * * Warning: This computer software sourcecode is protected by copyright law * and international treaties. Unauthorized reproduction or distribution * of this sourcecode, or any portion of it, may result in severe civil and * criminal penalties, and will be prosecuted to the maximum extent * possible under the law. */ package com.huawei.utils; import com.fasterxml.jackson.databind.DeserializationFeature; import com.fasterxml.jackson.databind.ObjectMapper; import com.fasterxml.jackson.databind.SerializationFeature; import java.io.IOException; public class JsonUtil { private static ObjectMapper objectMapper; static { objectMapper = new ObjectMapper(); // 设置FAIL_ON_EMPTY_BEANS属性,当序列化空对象不要抛异常 objectMapper.configure(SerializationFeature.FAIL_ON_EMPTY_BEANS, false); // 设置FAIL_ON_UNKNOWN_PROPERTIES属性,当JSON字符串中存在Java对象没有的属性,忽略 objectMapper.configure(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES, false); } /** * Convert Object to JsonString * * @param jsonObj * @return */ public static String jsonObj2Sting(Object jsonObj) { String jsonString = null; try { jsonString = objectMapper.writeValueAsString(jsonObj); } catch (IOException e) { System.out.printf("pasre json Object[{}] to string failed.", jsonString); } return jsonString; } /** * Convert JsonString to Simple Object * * @param jsonString * @param cls * @return */ public static <T> T jsonString2SimpleObj(String jsonString, Class<T> cls) { T jsonObj = null; try { jsonObj = objectMapper.readValue(jsonString, cls); } catch (IOException e) { System.out.printf("pasre json Object[{}] to string failed.", jsonString); } return jsonObj; } }
StreamClosedHttpResponse.java
/* * Copyright Notice: * Copyright 1998-2008, Huawei Technologies Co., Ltd. ALL Rights Reserved. * * Warning: This computer software sourcecode is protected by copyright law * and international treaties. Unauthorized reproduction or distribution * of this sourcecode, or any portion of it, may result in severe civil and * criminal penalties, and will be prosecuted to the maximum extent * possible under the law. */ package com.huawei.utils; import java.io.IOException; import java.util.Locale; import org.apache.http.Header; import org.apache.http.HeaderIterator; import org.apache.http.HttpEntity; import org.apache.http.HttpResponse; import org.apache.http.ProtocolVersion; import org.apache.http.StatusLine; import org.apache.http.params.HttpParams; @SuppressWarnings("deprecation") public class StreamClosedHttpResponse implements HttpResponse { private final HttpResponse original; private final String content; public StreamClosedHttpResponse(final HttpResponse original) throws UnsupportedOperationException, IOException { this.original = original; HttpEntity entity = original.getEntity(); if (entity != null && entity.isStreaming()) { String encoding = entity.getContentEncoding() != null ? entity.getContentEncoding().getValue() : null; content = StreamUtil.inputStream2String(entity.getContent(), encoding); } else { content = null; } } @Override public StatusLine getStatusLine() { return original.getStatusLine(); } @Override public void setStatusLine(final StatusLine statusline) { original.setStatusLine(statusline); } @Override public void setStatusLine(final ProtocolVersion ver, final int code) { original.setStatusLine(ver, code); } @Override public void setStatusLine(final ProtocolVersion ver, final int code, final String reason) { original.setStatusLine(ver, code, reason); } @Override public void setStatusCode(final int code) throws IllegalStateException { original.setStatusCode(code); } @Override public void setReasonPhrase(final String reason) throws IllegalStateException { original.setReasonPhrase(reason); } @Override public HttpEntity getEntity() { return original.getEntity(); } @Override public void setEntity(final HttpEntity entity) { original.setEntity(entity); } @Override public Locale getLocale() { return original.getLocale(); } @Override public void setLocale(final Locale loc) { original.setLocale(loc); } @Override public ProtocolVersion getProtocolVersion() { return original.getProtocolVersion(); } @Override public boolean containsHeader(final String name) { return original.containsHeader(name); } @Override public Header[] getHeaders(final String name) { return original.getHeaders(name); } @Override public Header getFirstHeader(final String name) { return original.getFirstHeader(name); } @Override public Header getLastHeader(final String name) { return original.getLastHeader(name); } @Override public Header[] getAllHeaders() { return original.getAllHeaders(); } @Override public void addHeader(final Header header) { original.addHeader(header); } @Override public void addHeader(final String name, final String value) { original.addHeader(name, value); } @Override public void setHeader(final Header header) { original.setHeader(header); } @Override public void setHeader(final String name, final String value) { original.setHeader(name, value); } @Override public void setHeaders(final Header[] headers) { original.setHeaders(headers); } @Override public void removeHeader(final Header header) { original.removeHeader(header); } @Override public void removeHeaders(final String name) { original.removeHeaders(name); } @Override public HeaderIterator headerIterator() { return original.headerIterator(); } @Override public HeaderIterator headerIterator(final String name) { return original.headerIterator(name); } @Override public String toString() { final StringBuilder sb = new StringBuilder("HttpResponseProxy{"); sb.append(original); sb.append('}'); return sb.toString(); } @Override @Deprecated public HttpParams getParams() { return original.getParams(); } @Override @Deprecated public void setParams(final HttpParams params) { original.setParams(params); } public String getContent() { return content; } }
StreamUtil.java
/* * Copyright Notice: * Copyright 1998-2008, Huawei Technologies Co., Ltd. ALL Rights Reserved. * * Warning: This computer software sourcecode is protected by copyright law * and international treaties. Unauthorized reproduction or distribution * of this sourcecode, or any portion of it, may result in severe civil and * criminal penalties, and will be prosecuted to the maximum extent * possible under the law. */ package com.huawei.utils; import java.io.Closeable; import java.io.IOException; import java.io.InputStream; import java.io.InputStreamReader; public class StreamUtil { private static final String DEFAULT_ENCODING = "utf-8"; public static String inputStream2String(InputStream in, String charsetName) { if (in == null) { return null; } InputStreamReader inReader = null; try { if (StringUtil.strIsNullOrEmpty(charsetName)) { inReader = new InputStreamReader(in, DEFAULT_ENCODING); } else { inReader = new InputStreamReader(in, charsetName); } int readLen = 0; char[] buffer = new char[1024]; StringBuilder strBuf = new StringBuilder(); while ((readLen = inReader.read(buffer)) != -1) { strBuf.append(buffer, 0, readLen); } return strBuf.toString(); } catch (IOException e) { System.out.println(e); } finally { closeStream(inReader); } return null; } public static void closeStream(Closeable closeable) { if (closeable != null) { try { closeable.close(); } catch (IOException e) { System.out.println(e); } } } }
StringUtil.java
/* * Copyright Notice: * Copyright 1998-2008, Huawei Technologies Co., Ltd. ALL Rights Reserved. * * Warning: This computer software sourcecode is protected by copyright law * and international treaties. Unauthorized reproduction or distribution * of this sourcecode, or any portion of it, may result in severe civil and * criminal penalties, and will be prosecuted to the maximum extent * possible under the law. */ package com.huawei.utils; //import java.nio.charset.Charset; import java.nio.charset.StandardCharsets; import java.text.SimpleDateFormat; import java.util.Base64; // JDK version≥1.8 import java.util.Calendar; import java.util.Locale; import java.util.TimeZone; import java.util.UUID; //import org.apache.commons.codec.binary.Base64; //JDK version<1.8 import javax.crypto.Mac; import javax.crypto.spec.SecretKeySpec; public class StringUtil { public static boolean strIsNullOrEmpty(String s) { return (null == s || s.trim().length() < 1); } public static String buildAKSKHeader(String appKey, String appSecret) throws Exception { if (StringUtil.strIsNullOrEmpty(appKey) || StringUtil.strIsNullOrEmpty(appSecret)) { return null; } SimpleDateFormat format = new SimpleDateFormat("yyyy-MM-dd'T'HH:mm:ss'Z'"); format.setTimeZone(TimeZone.getTimeZone("UTC")); Calendar calendar = Calendar.getInstance(); String time = format.format(calendar.getTime()); String stNonce = UUID.randomUUID().toString().replace("-", "").toUpperCase(Locale.ROOT); String str = stNonce + time; Mac mac = Mac.getInstance("HmacSHA256"); mac.init(new SecretKeySpec(appSecret.getBytes(StandardCharsets.UTF_8), "HmacSHA256")); byte[] authBytes = mac.doFinal(str.getBytes(StandardCharsets.UTF_8)); String passwordDigestBase64Str = encodeBase64(authBytes); return String.format(Constant.AKSK_HEADER_FORMAT, appKey, passwordDigestBase64Str, stNonce, time); } private static String encodeBase64(byte[] bytes) { if (bytes.length == 0) { return null; } else { return new String(org.apache.commons.codec.binary.Base64.encodeBase64(bytes), StandardCharsets.UTF_8); } } }