认证鉴权
调用接口有如下两种认证方式,您可以选择其中一种进行认证鉴权。
- Token认证:通过Token认证通用请求。
- AK/SK认证:通过AK(Access Key ID)/SK(Secret Access Key)加密调用请求。
Token认证
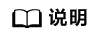
Token的有效期为24小时,需要使用一个Token鉴权时,可以先缓存起来,避免频繁调用。
Token在计算机系统中代表令牌(临时)的意思,拥有Token就代表拥有某种权限。Token认证就是在调用API的时候将Token加到请求消息头,从而通过身份认证,获得操作API的权限。
Token可通过调用获取用户Token接口获取,调用本服务API需要project级别的Token,即调用获取用户Token接口时,请求body中auth.scope的取值需要选择project,如下所示。
username、domainname 的获取请参考获取账号名和账号ID。password为用户密码。
{ "auth": { "identity": { "methods": [ "password" ], "password": { "user": { "name": "username", //IAM用户名 "password": "********", //密码 "domain": { "name": "domainname" //IAM用户所属账号名 } } } }, "scope": { "project": { "name": "xxxxxxxx" } } } }
获取Token后,再调用其他接口时,您需要在请求消息头中添加“X-Auth-Token”,其值即为Token。例如Token值为“ABCDEFJ....”,则调用接口时将“X-Auth-Token: ABCDEFJ....”加到请求消息头即可,如下所示。
GET https://iam.ap-southeast-1.myhuaweicloud.com/v3/auth/projects Content-Type: application/json X-Auth-Token: ABCDEFJ....
AK/SK认证
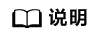
AK/SK签名认证、Token认证方式仅支持消息体大小12M以内的请求使用。
AK/SK认证就是使用AK/SK对请求进行签名,在请求时将签名信息添加到消息头,从而通过身份认证。
- AK(Access Key ID):访问密钥ID。与私有访问密钥关联的唯一标识符;访问密钥ID和私有访问密钥一起使用,对请求进行加密签名。
- SK(Secret Access Key):与访问密钥ID结合使用的密钥,对请求进行加密签名,可标识发送方,并防止请求被修改。
使用AK/SK认证时,您可以基于签名算法使用AK/SK对请求进行签名,也可以使用专门的签名SDK对请求进行签名。详细的签名方法和SDK使用方法请参见API签名指南。
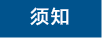
签名SDK只提供签名功能,与服务提供的SDK不同,使用时请注意。
AK/SK获取方式请参考获取AK/SK。
示例代码
下面代码展示了如何对一个请求进行签名,并通过AisAccess发送一个HTTPS请求的过程:
代码分成两个类进行演示:
ResponseProcessUtils:工具类,用于处理结果的返回。
ImageTaggingDemo:进行相关参数ak,sk,region的配置,访问图像标签服务的示例。
- ResponseProcessUtils.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77
package com.huawei.ais.demo; import java.io.FileOutputStream; import java.io.IOException; import java.nio.ByteBuffer; import java.nio.channels.FileChannel; import org.apache.http.HttpResponse; import com.alibaba.fastjson.JSON; import com.alibaba.fastjson.JSONObject; import com.cloud.sdk.util.Base64; import com.huawei.ais.sdk.util.HttpClientUtils; /** * 访问服务返回结果信息验证的工具类 */ public class ResponseProcessUtils { /** * 打印出服务访问完成的HTTP状态码 * * @param response 响应对象 */ public static void processResponseStatus(HttpResponse response) { System.out.println(response.getStatusLine().getStatusCode()); } /** * 打印出服务访问完成后,转化为文本的字符流,主要用于JSON数据的展示 * * @param response 响应对象 * @throws UnsupportedOperationException * @throws IOException */ public static void processResponse(HttpResponse response) throws UnsupportedOperationException, IOException { System.out.println(HttpClientUtils.convertStreamToString(response.getEntity().getContent())); } /** * 处理返回Base64编码的图像文件的生成 * * @param response * @throws UnsupportedOperationException * @throws IOException */ public static void processResponseWithImage(HttpResponse response, String fileName) throws UnsupportedOperationException, IOException { String result = HttpClientUtils.convertStreamToString(response.getEntity().getContent()); JSONObject resp = JSON.parseObject(result); String imageString = (String)resp.get("result"); byte[] fileBytes = Base64.decode(imageString); writeBytesToFile(fileName, fileBytes); } /** * 将字节数组写入到文件, 用于支持二进制文件(如图片)的生成 * @param fileName 文件名 * @param data 数据 * @throws IOException */ public static void writeBytesToFile(String fileName, byte[] data) throws IOException{ FileChannel fc = null; try { ByteBuffer bb = ByteBuffer.wrap(data); fc = new FileOutputStream(fileName).getChannel(); fc.write(bb); } catch (Exception e) { e.printStackTrace(); System.out.println(e.getMessage()); } finally { fc.close(); } } }
- ImageTaggingDemo.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80
package com.huawei.ais.demo.image; import com.alibaba.fastjson.JSON; import com.alibaba.fastjson.JSONObject; import com.alibaba.fastjson.serializer.SerializerFeature; import com.huawei.ais.demo.ResponseProcessUtils; import com.huawei.ais.demo.ServiceAccessBuilder; import com.huawei.ais.sdk.AisAccess; import com.huawei.ais.sdk.util.HttpClientUtils; import org.apache.commons.codec.binary.Base64; import org.apache.commons.io.FileUtils; import org.apache.http.HttpResponse; import org.apache.http.entity.StringEntity; import java.io.File; import java.io.IOException; /** * 图像标签服务的使用示例类 */ public class ImageTaggingDemo { // // 图像标签服务的使用示例函数 // private static void imageTaggingDemo() throws IOException { // 1. 图像标签服务的的基本信息,生成对应的一个客户端连接对象 AisAccess service = ServiceAccessBuilder.builder() .ak("######") // your ak .sk("######") // your sk .region("ap-southeast-1") // 图像识别服务中国-香港的配置 .connectionTimeout(5000) // 连接目标url超时限制 .connectionRequestTimeout(1000) // 连接池获取可用连接超时限制 .socketTimeout(20000) // 获取服务器响应数据超时限制 .build(); try { // // 2.构建访问图像标签服务需要的参数 // String uri = "/v1.0/image/tagging"; byte[] fileData = FileUtils.readFileToByteArray(new File("data/image-tagging-demo-1.jpg")); String fileBase64Str = Base64.encodeBase64String(fileData); JSONObject json = new JSONObject(); json.put("image", fileBase64Str); json.put("threshold", 60); StringEntity stringEntity = new StringEntity(json.toJSONString(), "utf-8"); // 3.传入图像标签服务对应的uri参数, 传入图像标签服务需要的参数, // 该参数主要通过JSON对象的方式传入, 使用POST方法调用服务 HttpResponse response = service.post(uri, stringEntity); // 4.验证服务调用返回的状态是否成功,如果为200, 为成功, 否则失败。 ResponseProcessUtils.processResponseStatus(response); // 5.处理服务返回的字符流,输出识别结果。 JSONObject jsonObject = JSON.parseObject(HttpClientUtils.convertStreamToString(response.getEntity().getContent())); System.out.println(JSON.toJSONString(JSON.parse(jsonObject.toString()), SerializerFeature.PrettyFormat)); } catch (Exception e) { e.printStackTrace(); } finally { // 6.使用完毕,关闭服务的客户端连接 service.close(); } } // // 主入口函数 // public static void main(String[] args) throws IOException { // 测试入口函数 imageTaggingDemo(); } }