Creating an HBase Table
Function Description
HBase allows you to create a table using the createTable method of the org.apache.hadoop.hbase.client.Admin object. You need to specify a table name and a column family name. You can create a table by using either of the following methods, but the latter one is recommended.
- Quickly create a table. A newly created table contains only one region, which will be automatically split into multiple new regions as data increases.
- Create a table using pre-assigned regions. You can pre-assign multiple regions before creating a table. This mode accelerates data write at the beginning of massive data write.
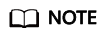
The column name and column family name of a table consist of letters, digits, and underscores (_) but cannot contain any special characters.
Sample Code
The following code snippets are in the testCreateTable method in the HBaseExample class of the com.huawei.bigdata.hbase.examples packet.
public void testCreateTable() { LOG.info("Entering testCreateTable: " + tableName); // Set the column family name to info. byte [] fam = Bytes.toBytes("info"); ColumnFamilyDescriptor familyDescriptor = ColumnFamilyDescriptorBuilder.newBuilder(fam) // Set data encoding methods. HBase provides DIFF,FAST_DIFF,PREFIX // HBase 2.0 removed `PREFIX_TREE` Data Block Encoding from column families. .setDataBlockEncoding(DataBlockEncoding.FAST_DIFF) // Set compression methods, HBase provides two default compression // methods:GZ and SNAPPY // GZ has the highest compression rate,but low compression and // decompression efficiency,fit for cold data // SNAPPY has low compression rate, but high compression and // decompression efficiency,fit for hot data. // it is advised to use SANPPY .setCompressionType(Compression.Algorithm.SNAPPY) .build(); TableDescriptor htd = TableDescriptorBuilder.newBuilder(tableName).setColumnFamily(familyDescriptor).build(); Admin admin = null; try { // Instantiate an Admin object. admin = conn.getAdmin(); if (!admin.tableExists(tableName)) { LOG.info("Creating table..."); admin.createTable(htd); LOG.info(admin.getClusterMetrics()); LOG.info(admin.listNamespaceDescriptors()); LOG.info("Table created successfully."); } else { LOG.warn("table already exists"); } } catch (IOException e) { LOG.error("Create table failed.", e); } finally { if (admin != null) { try { // Close the Admin object. admin.close(); } catch (IOException e) { LOG.error("Failed to close admin ", e); } } } LOG.info("Exiting testCreateTable."); }
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot