Connecting to a Database
After a database is connected, you can run SQL statements the database to perform operations on data.
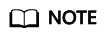
If you use an open-source Java Database Connectivity (JDBC) driver, ensure that the database parameter password_encryption_type is set to 1. If the value is not 1, the connection may fail. A typical error message is "none of the server's SASL authentication mechanisms are supported." To avoid such problems, perform the following operations:
- Set password_encryption_type to 1. For details, see Modifying Database Parameters.
- Create a new database user for connection or reset the password of the existing database user.
- If you use an administrator account, reset the password. For details, see Password Reset.
- If you are a common user, use another client tool (such as Data Studio) to connect to the database and run the ALTER USER statement to change your password.
- Connect to the database.
Here are the reasons why you need to perform these operations:
- MD5 algorithms may by vulnerable to collision attacks and cannot be used for password verification. Currently, GaussDB(DWS) uses the default security design. By default, MD5 password verification is disabled, but MD5 is required by the open-source libpq communication protocol of PostgreSQL. For connectivity purposes, you need to adjust the cryptographic algorithm parameter password_encryption_type and enable the MD5 algorithm.
- The database stores the hash digest of passwords instead of password text. During password verification, the system compares the hash digest with the password digest sent from the client (salt operations are involved). If you change your cryptographic algorithm policy, the database cannot generate a new MD5 hash digest for your existing password. For connectivity purposes, you must manually change your password or create a new user. The new password will be encrypted using the hash algorithm and stored for authentication in the next connection.
Function Prototype
JDBC provides the following three database connection methods:
- DriverManager.getConnection(String url);
- DriverManager.getConnection(String url, Properties info);
- DriverManager.getConnection(String url, String user, String password);
Parameter
Parameter |
Description |
---|---|
url |
gsjdbc4.jar database connection descriptor. The descriptor format can be:
NOTE:
If gsjdbc200.jar is used, replace jdbc:postgresql with jdbc:gaussdb.
|
info |
Database connection properties. Common properties include:
|
user |
Indicates a database user. |
password |
Indicates the password of a database user. |
Examples
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
//gsjdbc4.jar is used as an example. To use gsjdbc200.jar, replace the driver class name org.postgresql with com.huawei.gauss200.jdbc and replace the URL prefix jdbc:postgresql with jdbc:gaussdb. //The following code encapsulates database connection operations into an interface. The database can then be connected using an authorized username and password. public static Connection GetConnection(String username, String passwd) { //Set the driver class. String driver = "org.postgresql.Driver"; //Database connection descriptor. String sourceURL = "jdbc:postgresql://10.10.0.13:8000/postgres?currentSchema=test"; Connection conn = null; try { //Load the driver. Class.forName(driver); } catch (ClassNotFoundException e ){ e.printStackTrace(); return null; } try { //Establish a connection. conn = DriverManager.getConnection(sourceURL, username, passwd); System.out.println("Connection succeed!"); } catch (SQLException e) { e.printStackTrace(); return null; } return conn; } |
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot