Connecting to the Database (Using SSL)
The Go driver supports SSL connections to the database. After the SSL mode is enabled, if the Go driver connects to the database server in SSL mode, the Go driver uses the standard TLS 1.3 protocol by default, and the TLS version must be 1.2 or later. This section describes how applications establish an SSL connection to the database by using the Go driver. Before the connection, you must obtain the certificates and private keys required for the client and server. For details about obtaining these files, see related documents and commands of OpenSSL.
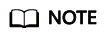
In SSL-based certificate authentication mode, you do not need to specify the user password in the connection string.
Configuring the Client
Upload the certificate files client.key, client.crt, and cacert. pem to the client.
Example 1:
// Take two-way authentication as an example. func main() { dsnStr := "host=127.0.0.1 port=1611 user=testuser password=Gauss_234 dbname=postgres sslcert=certs/client.crt sslkey=certs/client.key sslpassword=abcd " parameters := []string { " sslmode=require", " sslmode=verify-ca sslrootcert=certs/cacert.pem", } for _, param := range parameters { db, err:= sql.Open("opengauss", dsnStr+param) if err != nil { log.Fatal(err) } var f1 int err = db.QueryRow("select 1").Scan(&f1) if err != nil { log.Fatal(err) } else { fmt.Printf("RESULT: select 1: %d\n", f1) } db.Close() } }
Example 2:
// Verify sslpassword. func main() { dsnStr := "host=127.0.0.1 port=1611 user=testuser password=Gauss_234 dbname=postgres" connStrs := []string { " sslmode=verify-ca sslcert=certs/client_rsa.crt sslkey=certs/client_rsa.key sslpassword=abcd sslrootcert=certs/cacert_rsa.pem", " sslmode=verify-ca sslcert=certs/client_ecdsa.crt sslkey=certs/client_ecdsa.key sslpassword=abcd sslrootcert=certs/cacert_ecdsa.pem", } for _, connStr := range connStrs { db, err := sql.Open("opengauss", dsnStr + connStr) if err != nil { log.Fatal(err) } var f1 int err = db.QueryRow("select 1").Scan(&f1) if err != nil { if !strings.HasPrefix(err.Error(), "connect failed.") { log.Fatal(err) } } db.Close() } }
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot