Copying an Object
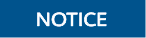
If you have any questions during development, post them on the Issues page of GitHub.
The object copy operation can create a copy for an existing object in OBS.
You can call copy_object to copy an object. When copying an object, you can rewrite properties and ACL for it, as well as set restriction conditions.

- If the source object to be copied is in the Archive storage class, you must restore it first.
Copying an Object in Simple Mode
The parameters are as follows:
Field |
Type |
Mandatory or Optional |
Description |
---|---|---|---|
option |
The context of the bucket. For details, see Configuring option. |
Mandatory |
Bucket parameter |
key |
char * |
Mandatory |
Object name |
version_id |
char * |
Optional |
Object version ID |
object_info |
obs_copy_destination_object_info * |
Mandatory |
Indicates the information of the copied object. |
object_info->destination_bucket |
char * |
Mandatory |
Bucket where the target object is located |
object_info->destination_key |
char * |
Mandatory |
Target object name |
object_info->last_modified_return |
int64_t * |
Mandatory |
Latest time when the object was modified |
object_info->etag_return_size |
int |
Mandatory |
Cache size of ETag |
object_info->etag_return |
char * |
Mandatory |
Cache of ETag |
is_copy |
unsigned int |
Mandatory |
Indicates whether the metadata of the target object is copied from the source object or replaced with the metadata contained in the request. |
put_properties |
obs_put_properties* |
Optional |
Properties of the uploaded object |
encryption_params |
server_side_encryption_params * |
Optional |
Configuring server-side encryption |
handler |
obs_response_handler * |
Mandatory |
Callback function |
callback_data |
void * |
Optional |
Callback data |
Sample code:
static void test_copy_object() { obs_status ret_status = OBS_STATUS_BUTT; char eTag[OBS_COMMON_LEN_256] = {0}; int64_t lastModified; // Create and initialize option. obs_options option; init_obs_options(&option); option.bucket_options.host_name = "<your-endpoint>"; option.bucket_options.bucket_name = "<Your bucketname>"; // Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables ACCESS_KEY_ID and SECRET_ACCESS_KEY. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. option.bucket_options.access_key = getenv("ACCESS_KEY_ID"); option.bucket_options.secret_access_key = getenv("SECRET_ACCESS_KEY"); // Set the destination object information. obs_copy_destination_object_info objectinfo ={0}; objectinfo.destination_bucket = target_bucket; objectinfo.destination_key = destinationKey; objectinfo.etag_return = eTag; objectinfo.etag_return_size = sizeof(eTag); objectinfo.last_modified_return = &lastModified; // Set response callback function. obs_response_handler responseHandler = { &response_properties_callback, &response_complete_callback }; // Copy an object. copy_object(&option, key, version_id, &objectinfo, 1, NULL, NULL,&responseHandler,&ret_status); if (OBS_STATUS_OK == ret_status) { printf("test_copy_object successfully. \n"); } else { printf("test_copy_object failed(%s).\n", obs_get_status_name(ret_status)); } }
Copying an Object by Specifying Conditions
When copying an object, you can specify one or more restriction conditions. If the conditions are met, the object will be copied. Otherwise, an exception will be thrown.
You can set the following conditions:
Parameter |
Description |
---|---|
if_modified_since |
Copies the source object if it has been modified since the specified time; otherwise, an exception is thrown. |
if_not_modified_since |
Copies the source object if it has not been modified since the specified time; otherwise, an exception is thrown. |
if_match_etag |
Copies the source object if its ETag is the same as the one specified by this parameter; otherwise, an exception is thrown. |
if_not_match_etag |
Copies the source object if its ETag is different from the one specified by this parameter; otherwise, an exception is thrown. |

- The ETag of the source object is the MD5 check value of the source object.
- If the object copy request includes if_not_modified_since, if_match_etag, if_modified_since, or if_not_match_etag, and the specified condition is not met, the copy will fail and an exception will be thrown with HTTP status code 412 precondition failed returned.
- if_modified_since and if_not_match_etag can be used together. So do if_not_modified_since and if_match_etag.
Sample code:
static void test_copy_object_condition() { obs_status ret_status = OBS_STATUS_BUTT; char eTag[OBS_COMMON_LEN_256] = {0}; int64_t lastModified; // Create and initialize option. obs_options option; init_obs_options(&option); option.bucket_options.host_name = "<your-endpoint>"; option.bucket_options.bucket_name = "<Your bucketname>"; // Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables ACCESS_KEY_ID and SECRET_ACCESS_KEY. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. option.bucket_options.access_key = getenv("ACCESS_KEY_ID"); option.bucket_options.secret_access_key = getenv("SECRET_ACCESS_KEY"); // Set the destination object information. obs_copy_destination_object_info objectinfo ={0}; objectinfo.destination_bucket = target_bucket; objectinfo.destination_key = destinationKey; objectinfo.etag_return = eTag; objectinfo.etag_return_size = sizeof(eTag); objectinfo.last_modified_return = &lastModified; // Set conditions. obs_put_properties putProperties={0}; init_put_properties(&putProperties); putProperties.get_conditions.if_match_etag = "<etag>"; putProperties.get_conditions.if_modified_since = "<time>"; putProperties.get_conditions.if_not_match_etag = "<etag>"; putProperties.get_conditions.if_not_modified_since = "<time>"; // Set response callback function. obs_response_handler responseHandler = { &response_properties_callback, &response_complete_callback }; // Copy an object. copy_object(&option, key, version_id, &objectinfo, 1, &putProperties, NULL,&responseHandler,&ret_status); if (OBS_STATUS_OK == ret_status) { printf("test_copy_object successfully. \n"); } else { printf("test_copy_object failed(%s).\n", obs_get_status_name(ret_status)); } }
Modifying an Object ACL
Sample code:
static void test_copy_object_acl() { obs_status ret_status = OBS_STATUS_BUTT; char eTag[OBS_COMMON_LEN_256] = {0}; int64_t lastModified; // Create and initialize option. obs_options option; init_obs_options(&option); option.bucket_options.host_name = "<your-endpoint>"; option.bucket_options.bucket_name = "<Your bucketname>"; // Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables ACCESS_KEY_ID and SECRET_ACCESS_KEY. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. option.bucket_options.access_key = getenv("ACCESS_KEY_ID"); option.bucket_options.secret_access_key = getenv("SECRET_ACCESS_KEY"); // Set the destination object information. obs_copy_destination_object_info objectinfo ={0}; objectinfo.destination_bucket = target_bucket; objectinfo.destination_key = destinationKey; objectinfo.etag_return = eTag; objectinfo.etag_return_size = sizeof(eTag); objectinfo.last_modified_return = &lastModified; // Modify an object ACL. obs_put_properties putProperties={0}; init_put_properties(&putProperties); putProperties.canned_acl = OBS_CANNED_ACL_PUBLIC_READ; // Set response callback function. obs_response_handler responseHandler = { &response_properties_callback, &response_complete_callback }; // Copy an object. copy_object(&option, key, version_id, &objectinfo, 1, &putProperties, NULL,&responseHandler,&ret_status); if (OBS_STATUS_OK == ret_status) { printf("test_copy_object successfully. \n"); } else { printf("test_copy_object failed(%s).\n", obs_get_status_name(ret_status)); } }
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot