Performing Initialization
Application Scenario
To use the OpenEye softphone interface to register a phone number, an agent needs to initialize the components.
Prerequisites
- The WebDemo has been downloaded.
- The local OpenEye program package has been installed and the local OpenEye program has been started.
- The server information in param.js has been configured.
Process Description
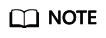
The code path in the demo is WebDemo\js\OpenEye_SDK.js.
- Initialize the SDK by referring to OpenEye_SDK (Creating and Initializing an Object).
After opening the webdemo page, you can receive a message returned by the OpenEye. Interfaces under OpeneyeLogin can be invoked only after the onOpeneyeLoginReady message is received. Interfaces under OpeneyeCall can be invoked only after the onOpeneyeCallReady message is received.
function initOpeneye(){ if (global_openEye_SDK== null) { global_openEye_SDK = new OpenEye_SDK({ onOpeneyeDeamonReady: onOpeneyeDeamonReady, onOpeneyeDeamonClose: onOpeneyeDeamonClose, serviceStartUp: serviceStartUp, serviceShutDown: serviceShutDown, onOpeneyeLoginReady: onOpeneyeLoginReady, onOpeneyeLoginClose: onOpeneyeLoginClose, onOpeneyeCallReady: onOpeneyeCallReady, onOpeneyeCallClose: onOpeneyeCallClose, onVersionInfoNotify : onVersionInfoNotify }); } } function onVersionInfoNotify(data) { writeLog("version is " + data.param.version); } function onOpeneyeDeamonReady() { writeLog("OpenEye Deamon is Ready"); } function onOpeneyeDeamonClose() { writeLog("OpenEye Deamon is Closed, please restart OpenEye Deamon it."); global_openEye_SDK= null; } function serviceStartUp() { writeLog("Openeye Service StartUp"); } function serviceShutDown() { writeLog("Openeye Service is shutdown, please restart it."); } function onOpeneyeLoginReady(){ writeLog("onOpeneyeLoginReady"); } function onOpeneyeLoginClose(){ writeLog("onOpeneyeLoginClose"); } function onOpeneyeCallClose() { writeLog("onOpeneyeCallClose"); } function onOpeneyeCallReady() { writeLog("onOpeneyeCallReady"); }
- Initialize OpenEyeCall parameters and listen to call events.
function initOpeneyeCall(localIp) { console.info("onOpeneyeCallReady"); initOpeneyeCall(); }
- Invoke the events of the OpenEyeCall that are listened on by invoking the setBasicCallEvent interface.
function initOpeneyeCall() { global_openEye_SDK.openEyeCall.setBasicCallEvent({ onCallIncoming: onCallIncoming, onCallOutGoing: onCallOutGoing, onCallRingBack: onCallRingBack, onCallConnected: onCallConnected, onCallEnded: onCallEnded, onCallEndedFailed: onCallEndedFailed, onCallRtpCreated: onCallRtpCreated, onCallOpenVideoReq: onCallOpenVideoReq }); }
- Invoke the config interface to set the SIP server information of OpenEyeCall.
Ensure that the SIP server information is correct. Otherwise, the account cannot be registered.
function sipBasicCfg() { global_cloudIPCC_SDK.openEyeCall.config({ networkInfo: { serverAddr: GlobalSipIp, sipServerPort: GlobalSipPort, sipTransportMode: 0, httpPort: 0 }, }, {response: configResponse}); } function configResponse(data) { if (data.result == 0) { writeLog("Set OpenEyeCall Sip Config Success."); register(); } else { writeLog("Set OpenEyeCall Sip Config Failed."); } }
- Invoke the events of the OpenEyeCall that are listened on by invoking the setBasicCallEvent interface.
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot