AK/SK-based Pushing Authentication
Function
After AK/SK authentication is enabled and the AK and SK are set, the platform adds the signature timestamp (X-Sdk-Date) and the hash code (Authorization) used for message authentication when pushing the HTTP status report.
Notes
It takes about 5 minutes for the AK/SK to take effect. During this period, the verification of status reports or uplink SMS push may fail. You can use the dual-AK/SK mode. That is, two AKs/SKs can take effect at the same time. The Access field in the Authorization request header can be used to determine the valid SK used by the current request.
Authentication Method
The Maven dependency needs to be introduced, which is used in the sample code to implement AK/SK signature.
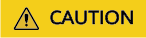
Replace the value of version in the following sample code with the actual SDK version. For details about SDK versions, see SDK Center.
<dependency> <groupId>org.apache.commons</groupId> <artifactId>commons-lang3</artifactId> <version>3.14.0</version> </dependency> <dependency> <groupId>commons-io</groupId> <artifactId>commons-io</artifactId> <version>2.11.0</version> </dependency> <dependency> <!--Replace it with the actual path.--> <systemPath>${project.basedir}/libs/java-sdk-core-XXX.jar</systemPath> <groupId>com.huawei.apigateway</groupId> <artifactId>java-sdk-core</artifactId> <version>SDK package version</version> <scope>system</scope> </dependency>
Java example:
@RestController public class StatusReportController { private static final Pattern AUTHORIZATION_PATTERN_SHA256 = Pattern.compile( "SDK-HMAC-SHA256\\s+Access=([^,]+),\\s?SignedHeaders=([^,]+),\\s?Signature=(\\w+)"); private static Map<String, String> secretMap = new HashMap<>(); static { secretMap.put("exampleAk", "exampleSk*1231d881wd"); } static class Response { int returnCode; String returnCodeDesc; Response(int returnCode, String returnCodeDesc) { this.returnCode = returnCode; this.returnCodeDesc = returnCodeDesc; } public int getReturnCode() { return returnCode; } public String getReturnCodeDesc() { return returnCodeDesc; } } @PostMapping("/status") public ResponseEntity<Response> smsHwStatusReport(HttpServletRequest request) { if (!doAuth(request)) { // If the authentication fails, the status code 401 is returned. return ResponseEntity .status(HttpStatus.UNAUTHORIZED) // Set the HTTP status to 401. .contentType(MediaType.APPLICATION_JSON) .body(new Response(401, "Unauthorized")); } // Process the status report normally. return ResponseEntity .status(HttpStatus.OK) .contentType(MediaType.APPLICATION_JSON) .body(new Response(0, "Success")); } public boolean doAuth(HttpServletRequest request) { try { if (StringUtils.isEmpty(request.getHeader("Authorization"))) { // The authorization header is not included. return false; } Matcher match = AUTHORIZATION_PATTERN_SHA256.matcher(request.getHeader("Authorization")); if (!match.find()) { // Incorrect Authorization format. return false; } String ak = match.group(1); // Obtain the access key. String body = new String(IOUtils.toByteArray(request.getInputStream()), StandardCharsets.UTF_8); // // Obtain the message body string. Request r = new Request(); r.setAppKey(ak); r.setSecret(secretMap.get(ak)); // Obtain the secret key. r.setUrl(request.getRequestURI()); // Obtain the message path. r.setBody(body); r.setMethod(request.getMethod()); Enumeration<String> headerNames = request.getHeaderNames(); while (headerNames.hasMoreElements()) { String headerName = headerNames.nextElement(); r.addHeader(headerName.toLowerCase(Locale.ROOT), request.getHeader(headerName)); } Signer signer = new Signer(); return signer.verify(r); } catch (Exception e) { return false; } } }
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot