60.7.1 Updates
1. Optimized the meeting UI, and changed the menu structure and APIs.
- Meeting locking information was added to the meeting information on the top of the meeting page, and the UI was optimized.
- Some functions in the More menu of the toolbar were moved to the Meeting settings menu under More.
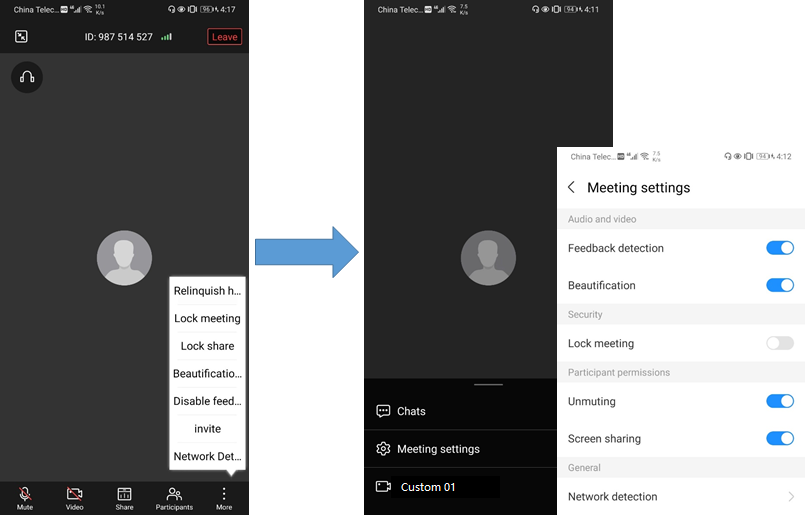
2. The chat function in meetings was added. You can customize whether to display the chat function. For details, see Enabling or Disabling Chat for a Meeting.
3. Optimized the APIs for customizing the meeting control menus, and replaced some APIs.
- On the Participants page in a meeting, the level-1 toolbar menu at the bottom can be customized.
- The menu bar can be configured for four scenarios: voice calls, video calls, voice meetings, and video meetings.
- Participants were distinguished. You can customize the menu displayed upon a click on the meeting title bar.
- The IConfMenuHandle API for configuring menus is discarded.
The following is an example. For details about how to use the APIs, see APIs.
/** * Specify the configuration policy of the meeting control toolbar at the bottom of the meeting, including the level-1 menu, the More menu after you touch the level-1 menu, and the More > Meeting settings menu. */ public interface IToolbarMenuStrategy { /** * Set the level-1 meeting control menu at the bottom of the meeting. * @return Returns the level-1 meeting control menu. */ List<IConfMenu> buildMenuItems(); /** * Set the menu items displayed after you touch More at the bottom of the meeting. * @return Returns the More menu items displayed. */ List<IConfMenu> buildMoreMenuItems(); /** * Set the menu items displayed after you touch More and choose Meeting settings. Two-level grouping is supported. * @return Returns the Meeting settings menu items. */ List<IConfMenu> buildSettingMenuItems(); }
/** * Define a callback on menu touch events. */ public interface OnMenuItemClickListener { /** * Callback invoked when a custom menu is touched. * @param confMenu Indicates the menu that is touched. * @param confInfo Indicates the meeting information. */ void onCustomMenuItemClick(IConfMenu confMenu, ConfInfo confInfo); }
Call the API.
// Customize a menu. if(DemoUtil.needCustom(NeedCustomType.CONF_MENU)){ sdkConfig.setConfMenuHandle(new CustomConfMenu()); ToolBarMenuProxy toolBarMenuProxy = new ToolBarMenuProxy(); // Customize the toolbar in a voice call. toolBarMenuProxy.setCallAudioToolbarHandle(new CustomCallAudioToolbarHandle()); // Customize the toolbar in a video call. toolBarMenuProxy.setCallVideoToolbarHandle(new CustomCallVideoToolbarHandle()); // Customize the toolbar in a voice meeting. toolBarMenuProxy.setConfAudioToolbarHandle(new CustomConfAudioToolbarHandle()); // Customize the toolbar in a video meeting. toolBarMenuProxy.setConfVideoToolbarHandle(new CustomConfVideoToolbarHandle()); sdkConfig.setToolBarMenuProxy(toolBarMenuProxy); // Customize the Participants menu in the meeting. sdkConfig.setParticipantMenuStrategy(new CustomParticipantMenuHandle()); // Customize the handle click events on the custom menus. sdkConfig.setOnMenuItemClickListener(new CustomOnMenuItemClickListener()); }
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot