Help Center/
Meeting/
Client SDK Reference/
iOS SDK/
API Reference/
UI Customization/
Customizing the Menu That Appears in Response to Touches on the Title Bar of the Meeting Page
Updated on 2024-07-30 GMT+08:00
Customizing the Menu That Appears in Response to Touches on the Title Bar of the Meeting Page
buildTitleBarPopViewItems
API Description
This API is used to customize the menu that appears in response to touches on the title bar of the meeting page.
Figure 1 Customizing the menu that appears in response to touches on the title bar of the meeting page
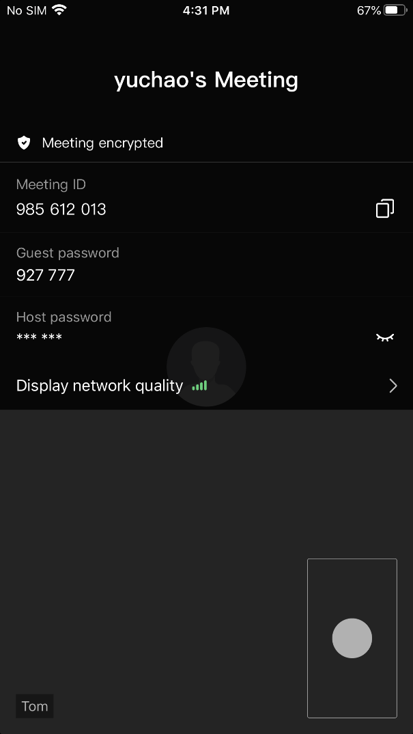
Precautions
- The host password is visible only to the host. Other customized menus are displayed in the same way for all participants.
- Customized cells need to comply with HWMInMeetingCellDelegate unless when you only change the default sequence.
Method Definition
1 2 3 4 5 6 7 8 9 |
- (NSArray <HWMInMeetingCellModel *> *)buildTitleBarPopViewItems; // Customize a cell as follows based on HWMInMeetingCellDelegate. /// Cell delegate /// @param cellType cellType - (UITableViewCell *)cellForRowWithCellType:(HWMConfInfoCellType)cellType inTableView:(UITableView *)tableView indexPath:(NSIndexPath *)indexPath; /// Cell tapping event delegate /// @param cellType cellType - (void)cellSelectedWithCellType:(HWMConfInfoCellType)cellType; |
Parameter Description
None
Return Values
None
Sample Code
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 |
/// Customize the menu that appears in response to touches on the title bar of the meeting page. - (NSArray <HWMInMeetingCellModel *> *)buildTitleBarPopViewItems{ HWMInMeetingCellModel *subjectCell = [HWMInMeetingCellModel defaultSubjectCell]; HWMInMeetingCellModel *encryptCell = [HWMInMeetingCellModel defaultEncryptedCell]; HWMInMeetingCellModel *confIdCell = [HWMInMeetingCellModel defaultConfIdCell]; HWMInMeetingCellModel *generalPasswordCell = [HWMInMeetingCellModel defaultGeneralPasswordCell]; HWMInMeetingCellModel *hostPasswordCell = [HWMInMeetingCellModel defaultHostPasswordCell]; HWMInMeetingCellModel *lockCell = [HWMInMeetingCellModel defaultConfLockCell]; HWMInMeetingCellModel *qosCell = [HWMInMeetingCellModel defaultQosCell]; return @[subjectCell,encryptCell, confIdCell, generalPasswordCell, hostPasswordCell, lockCell, qosCell]; } /// The height of the cell delegate can be adaptive (by adding layout constraints). You can customize the cell display. The following code is for reference only. /// @param cellType cellType - (UITableViewCell *)cellForRowWithCellType:(HWMConfInfoCellType)cellType inTableView:(nonnull UITableView *)tableView indexPath:(nonnull NSIndexPath *)indexPath{ NSString *reuseIdentifier = [NSString stringWithFormat:@"CustomCell_%ld", cellType]; UITableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:reuseIdentifier]; if (cell == nil) { cell = [[UITableViewCell alloc] initWithStyle:UITableViewCellStyleDefault reuseIdentifier:reuseIdentifier]; } cell.contentView.backgroundColor = [UIColor clearColor]; cell.backgroundColor = [UIColor clearColor]; cell.selectionStyle = UITableViewCellSelectionStyleNone; UIImageView * safeImg = [[UIImageView alloc] initWithImage:[UIImage imageNamed:@"custom_conf_setting"]]; [cell.contentView addSubview:safeImg]; UILabel * safeLabel = [[UILabel alloc] init]; safeLabel.font = [UIFont systemFontOfSize:14]; safeLabel.textColor = [UIColor whiteColor]; safeLabel.text = reuseIdentifier; [cell.contentView addSubview:safeLabel]; [safeImg mas_makeConstraints:^(MASConstraintMaker *make) { make.left.mas_equalTo(16); make.size.mas_equalTo(CGSizeMake(16, 16)); make.centerY.mas_equalTo(safeLabel); }]; [safeLabel mas_makeConstraints:^(MASConstraintMaker *make) { make.left.mas_equalTo(safeImg.mas_right).offset(10); make.top.mas_equalTo(10); make.height.mas_equalTo(34); make.bottom.mas_equalTo(-10); }]; return cell; } /// Cell tapping event delegate /// @param cellType cellType - (void)cellSelectedWithCellType:(HWMConfInfoCellType)cellType{ [UIUtil showMessage:[NSString stringWithFormat:@"Menu %lu tapped.", (unsigned long)cellType]]; } |
Parent topic: UI Customization
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
The system is busy. Please try again later.
For any further questions, feel free to contact us through the chatbot.
Chatbot