Customizing a Menu on the Participant List Page
buildParticipantToolBarMenuItems
API Description
This API is used to customize a menu at the bottom of the participant list page.
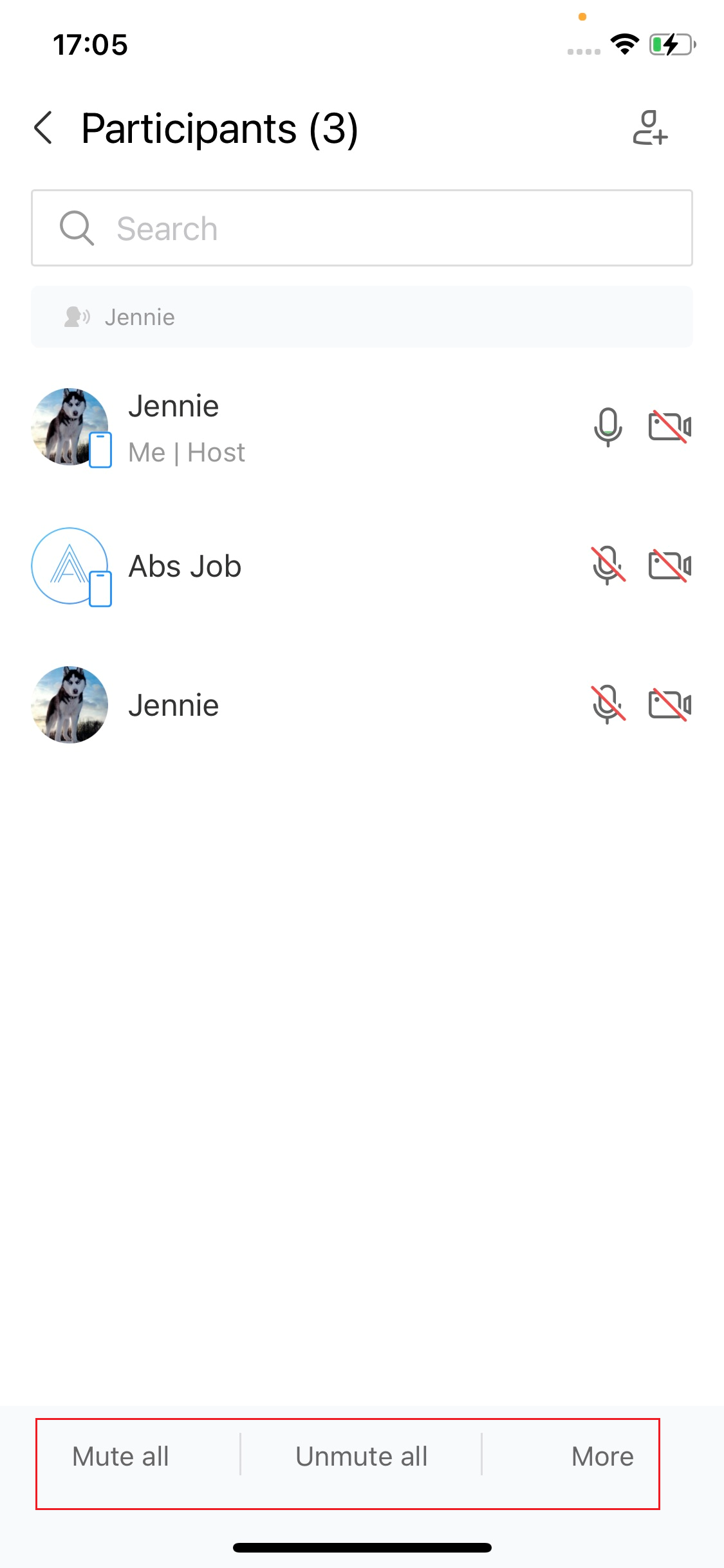
Precautions
None
Method Definition
1
|
- (NSArray <HWMConfToolBarMenuItem *> *)buildParticipantToolBarMenuItems; |
Parameter Description
None
Return Values
None
Sample Code
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
/// Customize a menu at the bottom of the participant list page. - (NSArray <HWMConfToolBarMenuItem *> *)buildParticipantToolBarMenuItems{ // Mute all participants. HWMConfToolBarMenuItem *muteAllItem = [HWMConfToolBarMenuItem defaultMuteAllItem]; // Unmute all participants. HWMConfToolBarMenuItem *unmuteAllItem = [HWMConfToolBarMenuItem defaultUnmuteAllItem]; // Customize More. HWMConfToolBarMenuItem *moreItem = [HWMConfToolBarMenuItem defaultMemberListMoreItem]; // Raise hands. HWMConfToolBarMenuItem *handupItem = [HWMConfToolBarMenuItem defaultHandupItem]; // Apply to be the host. HWMConfToolBarMenuItem *requestChairmanItem = [HWMConfToolBarMenuItem defaultRequestChairmanItem]; return @[muteAllItem, unmuteAllItem, moreItem, handupItem, requestChairmanItem]; } |
buildParticipantToolBarMoreMenuItems
API Description
This API is used to customize More at the bottom of the participant list page.
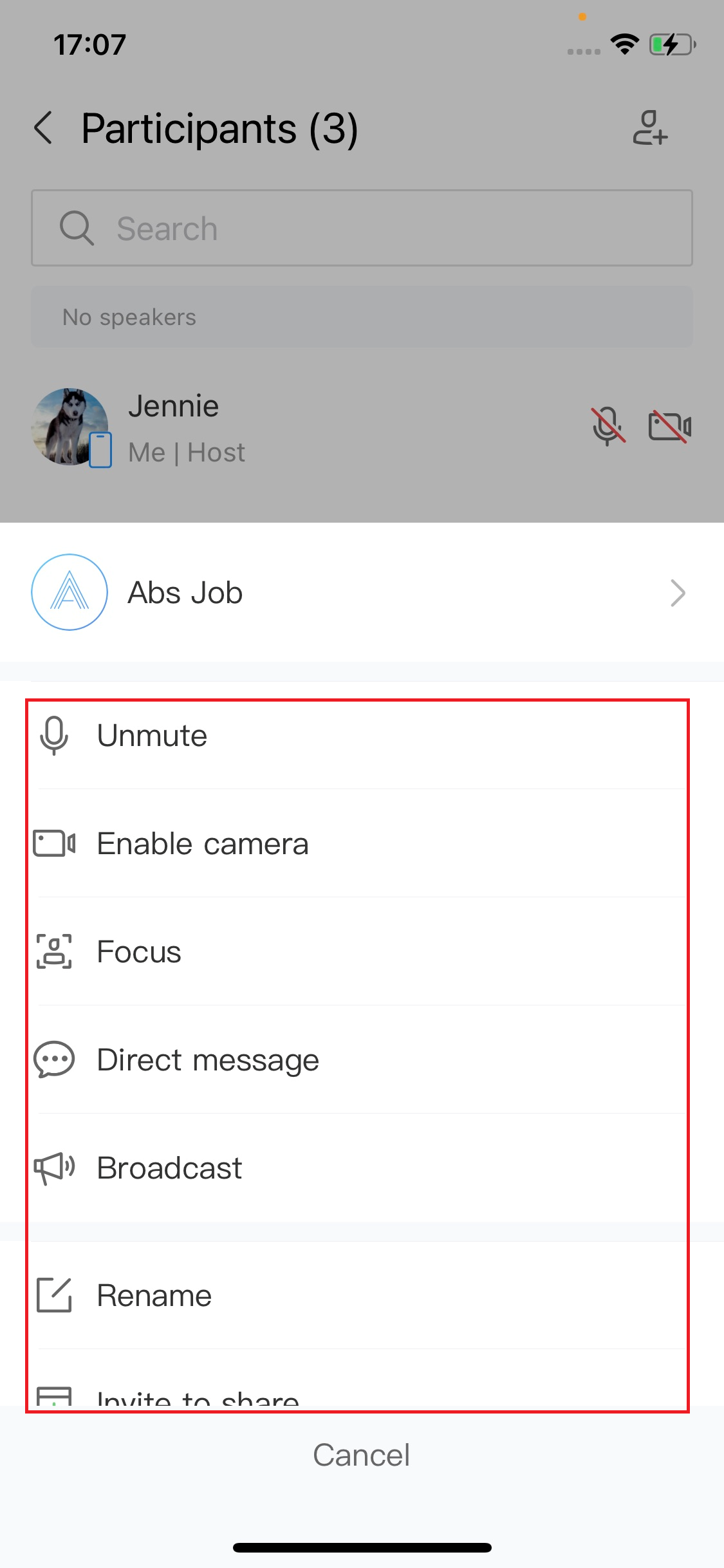
Precautions
None
Method Definition
1
|
- (NSArray <HWMConfMenuItem *> *)buildParticipantToolBarMoreMenuItems; |
Parameter Description
None
Return Values
None
Sample Code
1 2 3 4 5 6 7 8 9 10 11 |
/// Customize More at the bottom of the participant list page. - (NSArray <HWMConfMenuItem *> *)buildParticipantToolBarMoreMenuItems{ // Relinquish the host role. HWMConfMenuItem *releaseChairmanItem = [HWMConfMenuItem defaultReleaseChairmanItem]; // Lock the meeting. HWMConfMenuItem *lockItem = [HWMConfMenuItem defaultLockItem]; // Allow or forbid participants to unmute themselves. HWMConfMenuItem *allowUnmuteItem = [HWMConfMenuItem defaultAllowAttendeeUnmuteItem]; return @[releaseChairmanItem, lockItem, allowUnmuteItem]; } |
buildParticipantTitleBarMenuItems
API Description
This API is used to customize the menu in the upper right corner of the participant list page.
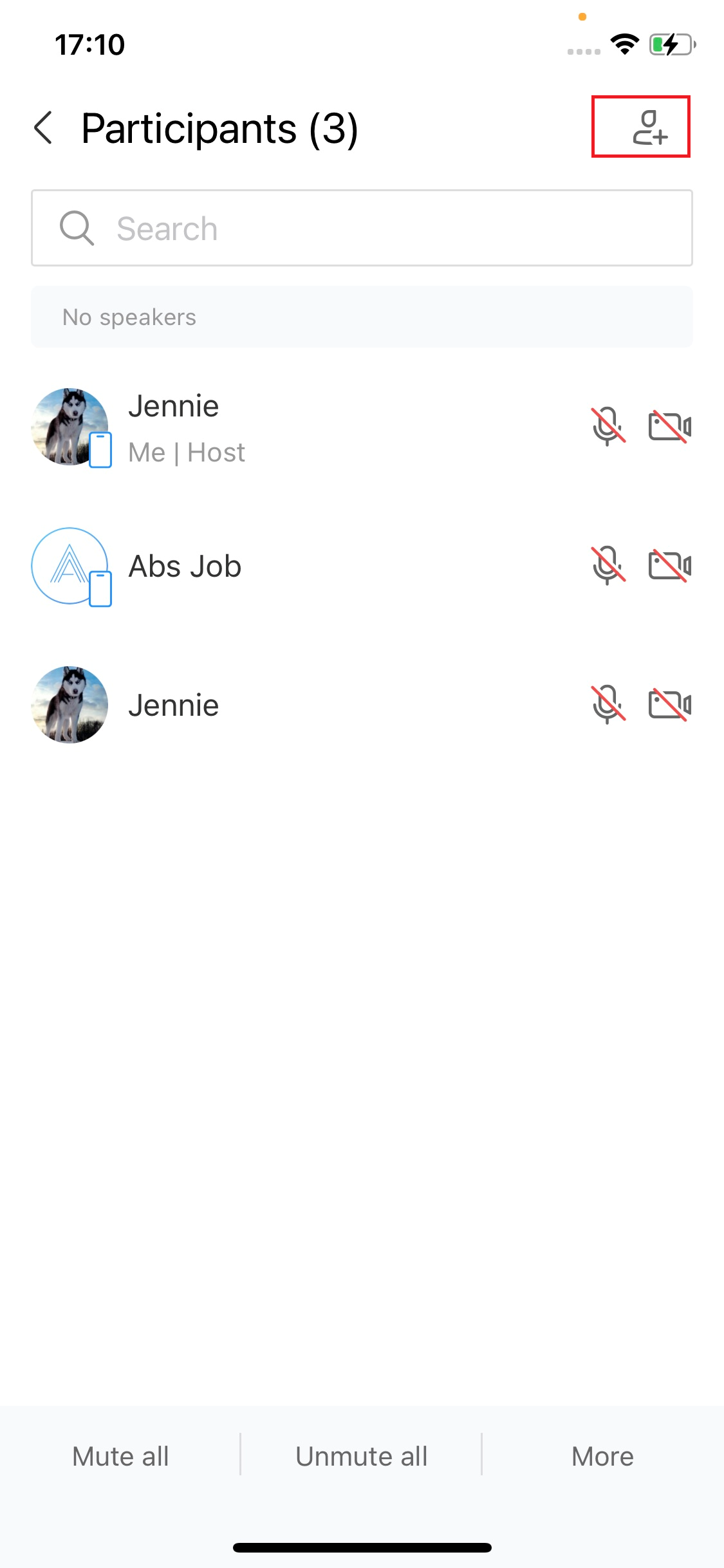
Precautions
None
Method Definition
1
|
- (NSArray <HWMConfToolBarMenuItem *> *)buildParticipantTitleBarMenuItems; |
Parameter Description
None
Return Values
None
Sample Code
1 2 3 4 5 6 7 |
/// Customize the menu in the upper right corner of the participant list page. - (NSArray <HWMConfToolBarMenuItem *> *)buildParticipantTitleBarMenuItems{ HWMConfToolBarMenuItem *shareItem = [HWMConfToolBarMenuItem defaultShareItem]; HWMConfToolBarMenuItem *addressBookItem = [HWMConfToolBarMenuItem defaultAddressBookItem]; return @[shareItem, addressBookItem]; } |
buildParticipantActionSheetItems
API Description
This API is used to customize an action sheet that appears in response to touching a participant in the participant list.
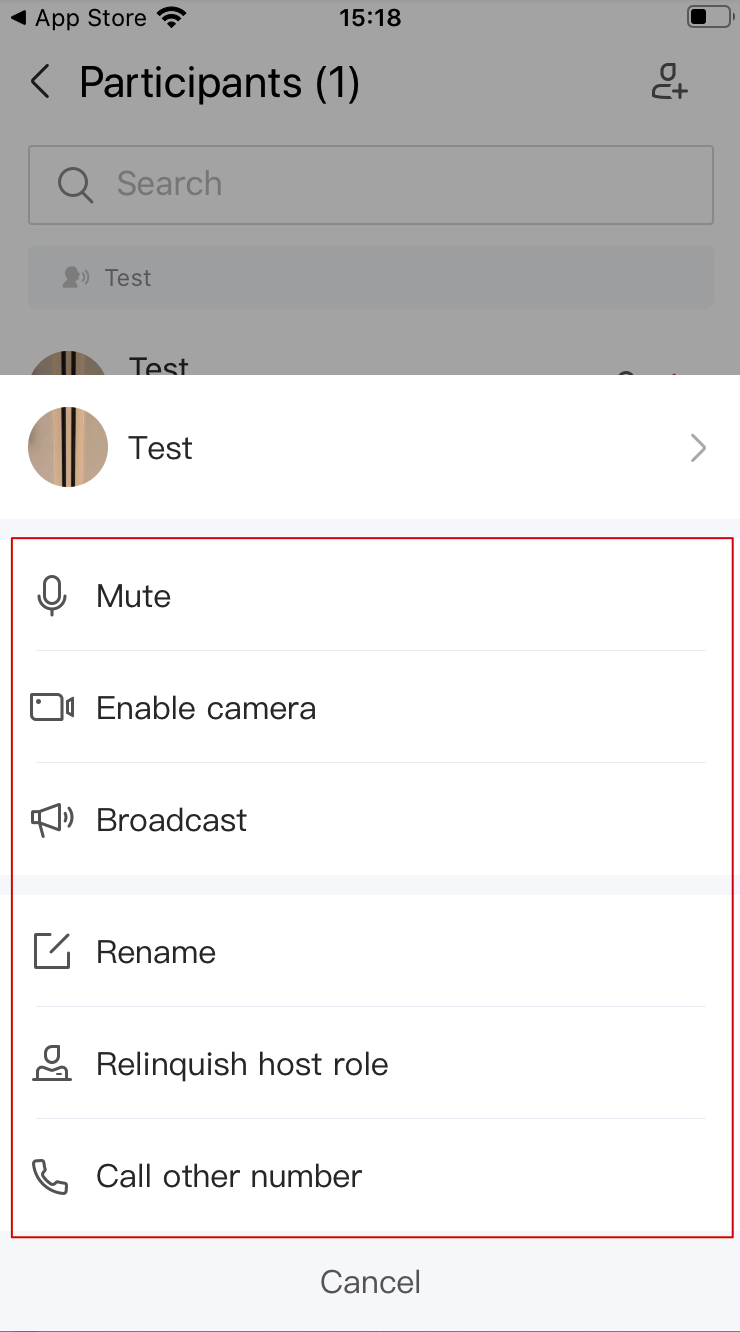
Precautions
None
Method Definition
1
|
- (NSArray <HWMConfParticipantActionMenuItem *> *)buildParticipantActionSheetItems; |
Parameter Description
None
Return Values
None
Sample Code
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
/// Customize an action sheet that appears in response to touches by participants. - (NSArray <HWMConfParticipantActionMenuItem *> *)buildParticipantActionSheetItems{ // Apply to be the host. HWMConfParticipantActionMenuItem *requestChairmanItem = (HWMConfParticipantActionMenuItem *)[HWMConfParticipantActionMenuItem defaultRequestChairmanItem]; // Relinquish the host role. HWMConfParticipantActionMenuItem *releaseChairmanItem = (HWMConfParticipantActionMenuItem *)[HWMConfParticipantActionMenuItem defaultReleaseChairmanItem]; // Start or stop broadcasting a participant. HWMConfParticipantActionMenuItem *broadcastItem = (HWMConfParticipantActionMenuItem *)[HWMConfParticipantActionMenuItem defaultBroadcastItem]; // Mute or unmute the microphone. HWMConfParticipantActionMenuItem *muteItem = (HWMConfParticipantActionMenuItem *)[HWMConfParticipantActionMenuItem defaultMuteItem]; // Hang up the call. HWMConfParticipantActionMenuItem *hangupItem = (HWMConfParticipantActionMenuItem *)[HWMConfParticipantActionMenuItem defaultHangupItem]; // Remove participants. HWMConfParticipantActionMenuItem *removeAttendeeItem = (HWMConfParticipantActionMenuItem *)[HWMConfParticipantActionMenuItem defaultRemoveAttendeeItem]; // Transfer the host role. HWMConfParticipantActionMenuItem *transferItem = (HWMConfParticipantActionMenuItem *)[HWMConfParticipantActionMenuItem defaultTransferChairmanItem]; // Focus on a participant or cancel focus. HWMConfParticipantActionMenuItem *watchItem = (HWMConfParticipantActionMenuItem *)[HWMConfParticipantActionMenuItem defaultWatchItem]; // Raise hands or put hands down. HWMConfParticipantActionMenuItem *handup = (HWMConfParticipantActionMenuItem *)[HWMConfParticipantActionMenuItem defaultHandupItem]; // Call other numbers. HWMConfParticipantActionMenuItem *callOtherItem = (HWMConfParticipantActionMenuItem *)[HWMConfParticipantActionMenuItem defaultCallOtherNumberItem]; // Change the display name in the meeting. HWMConfParticipantActionMenuItem *changeNameItem = (HWMConfParticipantActionMenuItem *)[HWMConfParticipantActionMenuItem defaultChangeNickNameItem]; // Recall the participant. HWMConfParticipantActionMenuItem *recallItem = (HWMConfParticipantActionMenuItem *)[HWMConfParticipantActionMenuItem defaultRecallItem]; return @[releaseChairmanItem, requestChairmanItem, broadcastItem, watchItem, muteItem, transferItem, recallItem, callOtherItem, changeNameItem, handup, hangupItem, removeAttendeeItem]; } |
Customizing the Profile Picture Click Event in buildParticipantActionSheetItems
API Description
This API is used to customize the event when a user touches the profile picture of a participant in the displayed action sheet.
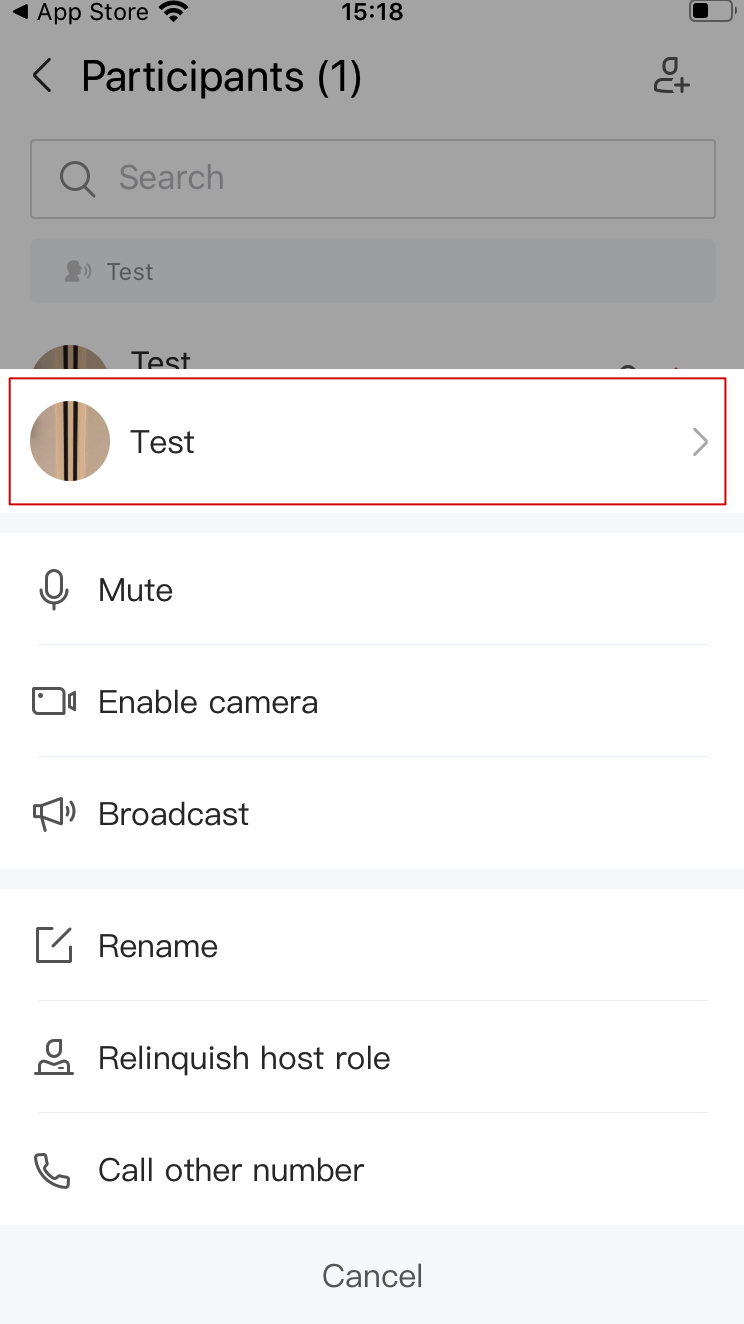
Precautions
- Customize buildParticipantActionSheetItems and add the defaultViewDetails item first.
- Set the delegate object in defaultViewDetails.
- Implement the click event in the delegate object.
Method Definition
None
Parameter Description
None
Return Values
None
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 |
/// Whether to display the arrow on the right of a profile picture and whether the profile picture area can be touched. - (BOOL)isShowAccessoryView:(HWMConfParticipant *)participant { // Return the value as required. return YES; } /// Customize an action sheet that appears in response to touches by participants. - (NSArray <HWMConfParticipantActionMenuItem *> *)buildParticipantActionSheetItems{ /// View details. HWMConfParticipantActionMenuItem *detailsItem = [HWMConfParticipantActionMenuItem defaultViewDetails]; detailsItem.delegate = self; detailsItem.actionClickEvent = ^(HWMConfCtrlType type, HWMConfParticipant * _Nonnull participant) { NSLog(@"Process the click event"); }; /// The preceding information is related to viewing details. // Apply to be the host. HWMConfParticipantActionMenuItem *requestChairmanItem = (HWMConfParticipantActionMenuItem *)[HWMConfParticipantActionMenuItem defaultRequestChairmanItem]; // Relinquish the host role. HWMConfParticipantActionMenuItem *releaseChairmanItem = (HWMConfParticipantActionMenuItem *)[HWMConfParticipantActionMenuItem defaultReleaseChairmanItem]; // Start or stop broadcasting a participant. HWMConfParticipantActionMenuItem *broadcastItem = (HWMConfParticipantActionMenuItem *)[HWMConfParticipantActionMenuItem defaultBroadcastItem]; // Mute or unmute the microphone. HWMConfParticipantActionMenuItem *muteItem = (HWMConfParticipantActionMenuItem *)[HWMConfParticipantActionMenuItem defaultMuteItem]; // Hang up the call. HWMConfParticipantActionMenuItem *hangupItem = (HWMConfParticipantActionMenuItem *)[HWMConfParticipantActionMenuItem defaultHangupItem]; // Remove participants. HWMConfParticipantActionMenuItem *removeAttendeeItem = (HWMConfParticipantActionMenuItem *)[HWMConfParticipantActionMenuItem defaultRemoveAttendeeItem]; // Transfer the host role. HWMConfParticipantActionMenuItem *transferItem = (HWMConfParticipantActionMenuItem *)[HWMConfParticipantActionMenuItem defaultTransferChairmanItem]; // Focus on a participant or cancel focus. HWMConfParticipantActionMenuItem *watchItem = (HWMConfParticipantActionMenuItem *)[HWMConfParticipantActionMenuItem defaultWatchItem]; // Raise hands or put hands down. HWMConfParticipantActionMenuItem *handup = (HWMConfParticipantActionMenuItem *)[HWMConfParticipantActionMenuItem defaultHandupItem]; // Call other numbers. HWMConfParticipantActionMenuItem *callOtherItem = (HWMConfParticipantActionMenuItem *)[HWMConfParticipantActionMenuItem defaultCallOtherNumberItem]; // Change the display name in the meeting. HWMConfParticipantActionMenuItem *changeNameItem = (HWMConfParticipantActionMenuItem *)[HWMConfParticipantActionMenuItem defaultChangeNickNameItem]; // Recall the participant. HWMConfParticipantActionMenuItem *recallItem = (HWMConfParticipantActionMenuItem *)[HWMConfParticipantActionMenuItem defaultRecallItem]; return @[releaseChairmanItem, requestChairmanItem, broadcastItem, watchItem, muteItem, transferItem, recallItem, callOtherItem, changeNameItem, handup, hangupItem, removeAttendeeItem]; } |
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot