Writing Data
Function
Use the KuduClient.newSession() method to generate a KuduSession object and execute the record insertion action to the Kudu table.
Sample Code
The code snippet for writing data is as follows:
// Create a KuduSession. KuduSession session = client.newSession(); for (int i = 0; i < numRows; i++) { Insert insert = table.newInsert(); PartialRow row = insert.getRow(); row.addInt("key", i); // Make even-keyed row have a null 'value'. if (i % 2 == 0) { row.setNull("value"); } else { row.addString("value", "value " + i); } session.apply(insert); } // Call session.close() to end the session and ensure the rows are // flushed and errors are returned. // You can also call session.flush() to do the same without ending the session. // When flushing in AUTO_FLUSH_BACKGROUND mode (the default mode recommended // for most workloads, you must check the pending errors as shown below, since // write operations are flushed to Kudu in background threads. session.close(); if (session.countPendingErrors() != 0) { System.out.println("errors inserting rows"); org.apache.kudu.client.RowErrorsAndOverflowStatus roStatus = session.getPendingErrors(); org.apache.kudu.client.RowError[] errs = roStatus.getRowErrors(); int numErrs = Math.min(errs.length, 5); System.out.println("there were errors inserting rows to Kudu"); System.out.println("the first few errors follow:"); for (int i = 0; i < numErrs; i++) { System.out.println(errs[i]); } if (roStatus.isOverflowed()) { System.out.println("error buffer overflowed: some errors were discarded"); } throw new RuntimeException("error inserting rows to Kudu"); } System.out.println("Inserted " + numRows + " rows");
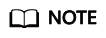
In the sample code, numRows indicates the number of records to be written. Pay special attention to the response result of the request.
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot