Help Center/
MapReduce Service/
Developer Guide (Normal_3.x)/
Kudu Development Guide (Normal Mode)/
Developing an Application/
Sample Code Description/
Read Data
Updated on 2022-08-16 GMT+08:00
Read Data
Function
Generate a KuduScanner object by using the KuduClient.newScannerBuilder(KuduTable table) method, and then read data from the Kudu table by setting the predicate condition.
Sample Code
The code snippet for reading data is as follows:
KuduTable table = client.openTable(tableName); Schema schema = table.getSchema(); // Scan with a predicate on the 'key' column, returning the 'value' and "added" columns. List<String> projectColumns = new ArrayList<>(2); projectColumns.add("key"); projectColumns.add("value"); projectColumns.add("added"); int lowerBound = 0; KuduPredicate lowerPred = KuduPredicate.newComparisonPredicate( schema.getColumn("key"), ComparisonOp.GREATER_EQUAL, lowerBound); int upperBound = numRows / 2; KuduPredicate upperPred = KuduPredicate.newComparisonPredicate( schema.getColumn("key"), ComparisonOp.LESS, upperBound); KuduScanner scanner = client.newScannerBuilder(table) .setProjectedColumnNames(projectColumns) .addPredicate(lowerPred) .addPredicate(upperPred) .build(); // Check the correct number of values and null values are returned, and // that the default value was set for the new column on each row. // Note: scanning a hash-partitioned table will not return results in primary key order. int resultCount = 0; int nullCount = 0; while (scanner.hasMoreRows()) { RowResultIterator results = scanner.nextRows(); while (results.hasNext()) { RowResult result = results.next(); if (result.isNull("value")) { nullCount++; } double added = result.getDouble("added"); if (added != DEFAULT_DOUBLE) { throw new RuntimeException("expected added=" + DEFAULT_DOUBLE + " but got added= " + added); } resultCount++; } }
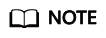
In the sample code:
- projectColumns indicates the column information to be returned.
- lowerPred and upperBound indicate the predicates that take effect on the primary key.
Parent topic: Sample Code Description
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
The system is busy. Please try again later.
For any further questions, feel free to contact us through the chatbot.
Chatbot