Using the keytab File for JDBC Authentication
Description
Use the keytab file for JDBC authentication.
Preparations
Log in to FusionInsight Manager, choose System > Permission > User, and download the user credential prepared in Preparing the Developer Account.
Sample Code
The following code snippet is used as an example. For complete code, see the com.huawei.bigdata.iotdb.JDBCbyKerberosExample class.
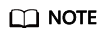
- On FusionInsight Manager, choose Cluster > Services > IoTDB > Instance to view the IP address of the node where the IoTDBServer to be connected is located.
- To obtain the RPC port number, log in to FusionInsight Manager, choose Cluster > Services > IoTDB, click Configuration, and click All Configurations, and search for IOTDB_SERVER_RPC_PORT.
- In security mode, the username and password for logging in to the node where IoTDBServer resides are controlled by FusionInsight Manager. Ensure that the user has the permission to operate the IoTDB service. For details, see Preparing the Developer Account.
- Log in to FusionInsight Manager and choose System > Permission > Domain and Mutual Trust. On the page that is displayed, the value of Local Domain is the domain name.
package com.huawei.bigdata.iotdb; import com.huawei.iotdb.client.security.IoTDBClientKerberosFactory; import org.apache.iotdb.jdbc.IoTDBSQLException; import org.ietf.jgss.GSSException; import java.sql.Connection; import java.sql.DriverManager; import java.sql.SQLException; import java.sql.Statement; import java.util.Base64; import javax.security.auth.login.LoginException; public class JDBCbyKerberosExample { /** * In security mode, the default value of SSL_ENABLE is true. You need to import the truststore.jks file. * In security mode, you can also log in to FusionInsight Manager, choose Cluster > Services > IoTDB > Configuration, search for SSL in the search box, and change the value of SSL_ENABLE to false. After saving the configuration, restart the IoTDB service for the configuration to take effect. Modify the following configuration in the iotdb-client.env file in the Client installation directory/IoTDB/iotdb/conf directory on the client: iotdb_ssl_enable="false" */ private static final String IOTDB_SSL_ENABLE = "true"; // Set it to the SSL_ENABLE value. private static final String JAVA_KRB5_CONF = "java.security.krb5.conf"; /** * Location of krb5.conf file */ private static final String KRB5_CONF_DEST = "Location of the krb5.conf file in the downloaded authentication credential"; /** * Location of keytab file */ private static final String KEY_TAB_DEST = "Location of the user.keytab file in the downloaded authentication credential"; /** * User principal */ private static final String CLIENT_PRINCIPAL = "User Principal (usually in the format of username@local domain, for example, iotdb_admin@HADOOP.COM)"; /** * Server principal, 'iotdb_server_kerberos_principal' in iotdb-datanode.properties */ private static final String SERVER_PRINCIPAL = "iotdb/hadoop.hadoop.com@HADOOP.COM";// You can obtain this parameter value by searching for iotdb_server_kerberos_principal in ${BIGDATA_HOME}/FusionInsight_IoTDB_*/*_*_IoTDBServer/etc/iotdb-datanode.properties on any node with the IoTDB service installed. /** * Get kerberos token as password * @return kerberos token * @throws LoginException loginException * @throws GSSException GSSException */ public static String getAuthToken() throws LoginException, GSSException { IoTDBClientKerberosFactory kerberosHandler = IoTDBClientKerberosFactory.getInstance(); System.setProperty(JAVA_KRB5_CONF, KRB5_CONF_DEST); kerberosHandler.loginSubjectFromKeytab(PRINCIPAL, KEY_TAB_DEST); byte[] tokens = kerberosHandler.generateServiceToken(PRINCIPAL); return Base64.getEncoder().encodeToString(tokens); } public static void main(String[] args) throws SQLException { // set iotdb_ssl_enable System.setProperty("iotdb_ssl_enable", IOTDB_SSL_ENABLE); if ("true".equals(IOTDB_SSL_ENABLE)) { // set truststore.jks path System.setProperty("iotdb_ssl_truststore", "truststore file path"); } try (Connection connection = DriverManager.getConnection("jdbc:iotdb://IP address of the IoTDBServer instance node:port number/", "Authentication username", getAuthToken()); Statement statement = connection.createStatement()) { // set JDBC fetchSize statement.setFetchSize(10000); try { statement.execute("SET STORAGE GROUP TO root.sg1"); statement.execute( "CREATE TIMESERIES root.sg1.d1.s1 WITH DATATYPE=INT64, ENCODING=RLE, COMPRESSOR=SNAPPY"); statement.execute( "CREATE TIMESERIES root.sg1.d1.s2 WITH DATATYPE=INT64, ENCODING=RLE, COMPRESSOR=SNAPPY"); statement.execute( "CREATE TIMESERIES root.sg1.d1.s3 WITH DATATYPE=INT64, ENCODING=RLE, COMPRESSOR=SNAPPY"); } catch (IoTDBSQLException e) { System.out.println(e.getMessage()); } } catch (GSSException | LoginException e) { System.out.println(e.getMessage()); } } }
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot