Java Example Code
Description
Run the IoTDB SQL statement in JDBC connection mode.
Sample Code
The following code snippet is used as an example. For complete code, see the com.huawei.bigdata.iotdb.JDBCExample class.
jdbc url includes the IP address, RPC port number, username, and password of the node where the IoTDBServer to be connected is located.
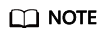
- On FusionInsight Manager, choose Cluster > Services > IoTDB > Instance to view the IP address of the node where the IoTDBServer to be connected is located.
- To obtain the RPC port number, log in to FusionInsight Manager, choose Cluster > Services > IoTDB, click Configuration, and click All Configurations, and search for IOTDB_SERVER_RPC_PORT.
- In security mode, the username and password for logging in to the node where IoTDBServer resides are controlled by FusionInsight Manager. Ensure that the user has the permission to operate the IoTDB service. For details, see Preparing for User Authentication.
- You need to set the username and password for authentication in the local environment variables. You are advised to store the username and password in ciphertext and decrypt them upon using.
- Authentication username is the username for accessing IoTDB.
- Password is the password for accessing IoTDB.
/** * In security mode, the default value of SSL_ENABLE is true. You need to import the truststore.jks file. * In security mode, you can also log in to FusionInsight Manager, choose Cluster > Services > IoTDB > Configuration, search for SSL in the search box, and change the value of SSL_ENABLE to false. After saving the configuration, restart the IoTDB service for the configuration to take effect. Modify the following configuration in the iotdb-client.env file in the Client installation directory/IoTDB/iotdb/conf directory on the client: iotdb_ssl_enable="false" */ private static final String IOTDB_SSL_ENABLE = "true"; // Set it to the SSL_ENABLE value. public static void main(String[] args) throws ClassNotFoundException, SQLException { Class.forName("org.apache.iotdb.jdbc.IoTDBDriver"); // set iotdb_ssl_enable System.setProperty("iotdb_ssl_enable", IOTDB_SSL_ENABLE); if ("true".equals(IOTDB_SSL_ENABLE)) { // set truststore.jks path System.setProperty("iotdb_ssl_truststore", "truststore file path"); } try (Connection connection = DriverManager.getConnection("jdbc:iotdb://IP address of the IoTDBServer instance node:Port/", "authentication username", "authentication user password"); Statement statement = connection.createStatement()) { // set JDBC fetchSize statement.setFetchSize(10000); for (int i = 0; i <= 100; i++) { statement.addBatch(prepareInsertStatment(i)); } statement.executeBatch(); statement.clearBatch(); ResultSet resultSet = statement.executeQuery("select ** from root where time <= 10"); outputResult(resultSet); resultSet = statement.executeQuery("select count(**) from root"); outputResult(resultSet); resultSet = statement.executeQuery( "select count(**) from root where time >= 1 and time <= 100 group by ([0, 100), 20ms, 20ms)"); outputResult(resultSet); } catch (IoTDBSQLException e) { System.out.println(e.getMessage()); } }
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot